Java Reference
In-Depth Information
68
// return Employee's first and last name combined
69
public
String getName()
70
{
71
return
String.format(
"%s %s"
, getFirstName(), getLastName());
72
}
73
74
// return a String containing the Employee's information
75
@Override
76
public
String toString()
77
{
78
return
String.format(
"%-8s %-8s %8.2f %s"
,
79
getFirstName(), getLastName(), getSalary(), getDepartment());
80
}
// end method toString
81
}
// end class Employee
Fig. 17.9
|
Employee
class for use in Figs. 17.10-17.16. (Part 3 of 3.)
Class
ProcessingEmployees
(Figs. 17.10-17.16) is split into several figures so we can
show you the lambda and streams operations with their corresponding outputs.
Figure 17.10 creates an array of
Employee
s (lines 17-24) and gets its
List
view (line 27).
1
// Fig. 17.10: ProcessingEmployees.java
2
// Processing streams of Employee objects.
3
import
java.util.Arrays;
4
import
java.util.Comparator;
5
import
java.util.List;
6
7
8
9
10
11
12
import
java.util.Map;
import
java.util.TreeMap;
import
java.util.function.Function;
import
java.util.function.Predicate;
import
java.util.stream.Collectors;
public class
ProcessingEmployees
13
{
14
public static void
main(String[] args)
15
{
16
// initialize array of Employees
17
Employee[] employees = {
18
new
Employee(
"Jason"
,
"Red"
,
5000
,
"IT"
),
19
new
Employee(
"Ashley"
,
"Green"
,
7600
,
"IT"
),
20
new
Employee(
"Matthew"
,
"Indigo"
,
3587.5
,
"Sales"
),
21
new
Employee(
"James"
,
"Indigo"
,
4700.77
,
"Marketing"
),
22
new
Employee(
"Luke"
,
"Indigo"
,
6200
,
"IT"
),
23
new
Employee(
"Jason"
,
"Blue"
,
3200
,
"Sales"
),
24
new
Employee(
"Wendy"
,
"Brown"
,
4236.4
,
"Marketing"
)};
25
26
// get List view of the Employees
27
List<Employee> list = Arrays.asList(employees);
Fig. 17.10
|
Creating an array of
Employee
s, converting it to a
List
and displaying the
List
.
(Part 1 of 2.)
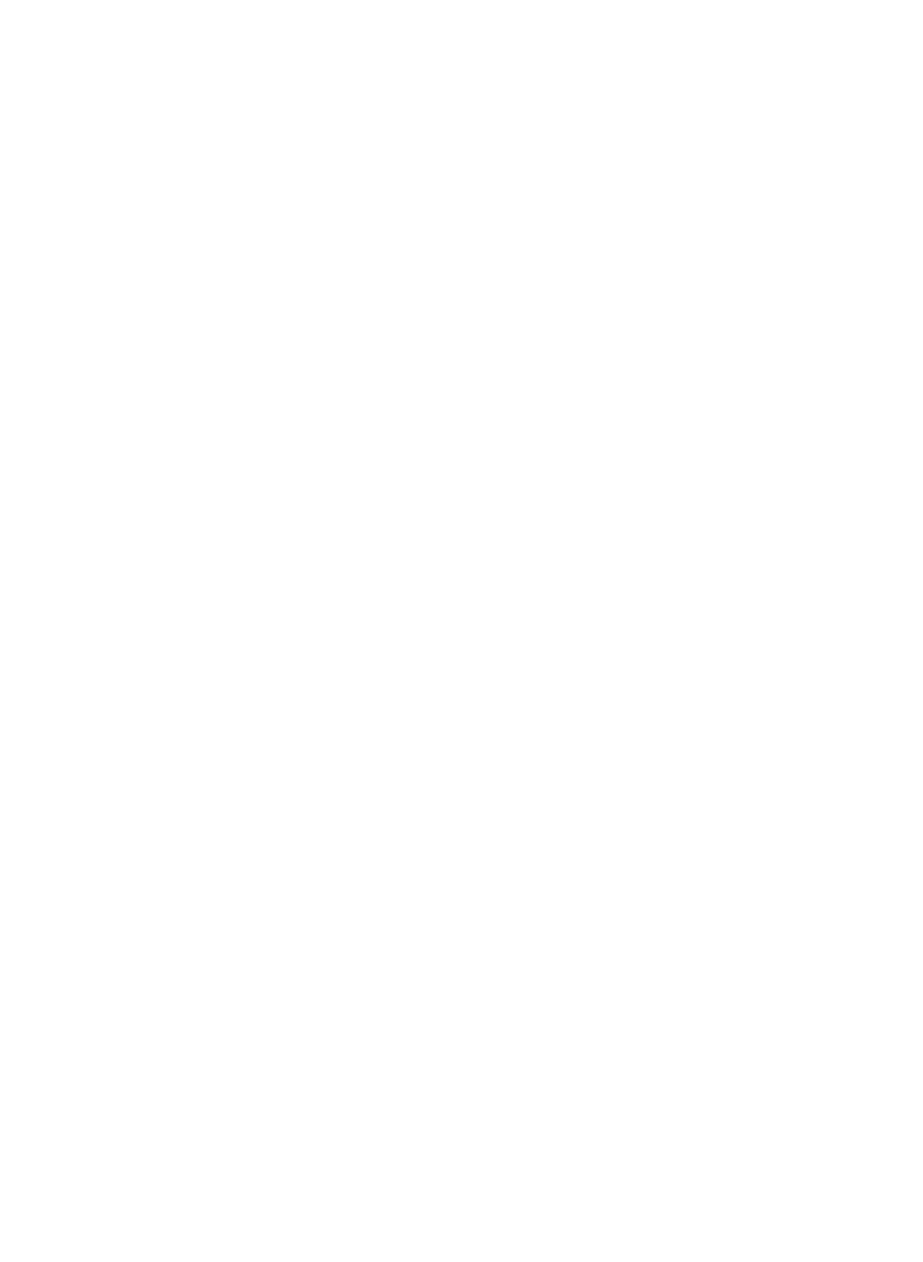


















