Java Reference
In-Depth Information
26
// output list contents
27
System.out.println(
"ArrayList: "
);
28
29
for
(
int
count =
0
; count <
list.size()
list.get(count)
; count++)
30
System.out.printf(
"%s "
,
);
31
32
// remove from list the colors contained in removeList
33
removeColors(list, removeList);
34
35
// output list contents
36
System.out.printf(
"%n%nArrayList after calling removeColors:%n"
);
37
38
for
(String color : list)
39
System.out.printf(
"%s "
, color);
40
}
41
42
// remove colors specified in collection2 from collection1
43
private static void
removeColors(
Collection<String> collection1
,
44
Collection<String> collection2
)
45
{
46
// get iterator
47
Iterator<String> iterator = collection1.iterator();
48
49
// loop while collection has items
50
while
(
iterator.hasNext()
)
51
{
52
if
(collection2.contains(iterator.next()))
iterator.remove();
// remove current element
53
54
}
55
}
56
}
// end class CollectionTest
ArrayList:
MAGENTA RED WHITE BLUE CYAN
ArrayList after calling removeColors:
MAGENTA CYAN
Fig. 16.2
|
Collection
interface demonstrated via an
ArrayList
object. (Part 2 of 2.)
Lines 13 and 20 declare and initialize
String
arrays
colors
and
removeColors
. Lines
14 and 21 create
ArrayList<String>
objects and assign their references to
List<String>
variables
list
and
removeList
, respectively. Recall that
ArrayList
is a
generic
class, so we
can specify a
type argument
(
String
in this case) to indicate the type of the elements in each
list. Because you specify the type to store in a collection at compile time, generic collec-
tions provide compile-time
type safety
that allows the compiler to catch attempts to use
invalid types. For example, you cannot store
Employee
s in a collection of
String
s.
Lines 16-17 populate
list
with
String
s stored in array
colors
, and lines 23-24 pop-
ulate
removeList
with
String
s stored in array
removeColors
using
List
method
add
.
Lines 29-30 output each element of
list
. Line 29 calls
List
method
size
to get the
number of elements in the
ArrayList
. Line 30 uses
List
method
get
to retrieve indi-
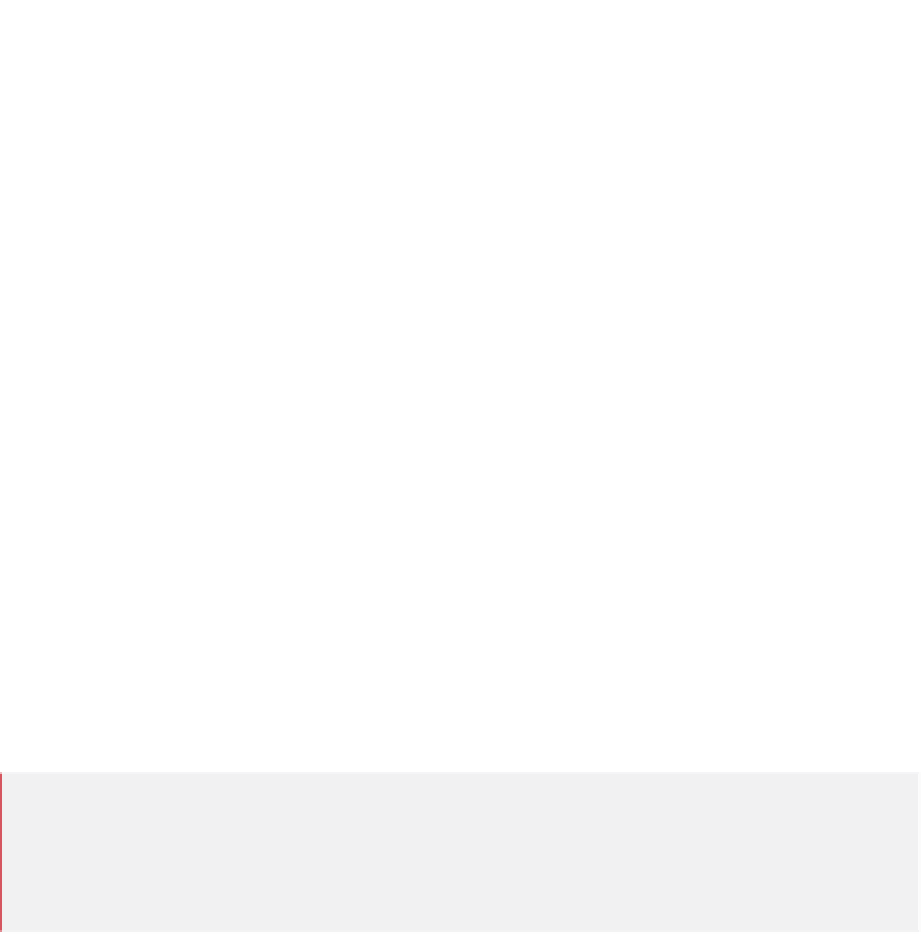




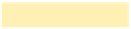










