Java Reference
In-Depth Information
Answers to Self-Review Exercises
10.1
a) abstract. b) concrete. c) Polymorphism. d)
abstract
. e) downcasting.
10.2
a) False. An abstract class can include methods with implementations and
abstract
meth-
ods. b) False. Trying to invoke a subclass-only method with a superclass variable is not allowed.
c) False. Only a concrete subclass must implement the method. d) True.
10.3
a)
default
methods. b)
static
. c) functional interface.
Exercises
10.4
How does polymorphism enable you to program “in the general” rather than “in the spe-
cific”? Discuss the key advantages of programming “in the general.”
10.5
What are abstract methods? Describe the circumstances in which an abstract method would
be appropriate.
10.6
How does polymorphism promote extensibility?
10.7
Discuss three proper ways in which you can assign superclass and subclass references to vari-
ables of superclass and subclass types.
10.8
Compare and contrast abstract classes and interfaces. Why would you use an abstract class?
Why would you use an interface?
10.9
(Java SE 8 Interfaces)
Explain how
default
methods enable you to add new methods to an
existing interface without breaking the classes that implemented the original interface.
10.10
(Java SE 8 Interfaces)
What is a functional interface?
10.11
(Java SE 8 Interfaces)
Why is it useful to be able to add
static
methods to interfaces?
10.12
(Payroll System Modification)
Modify the payroll system of Figs. 10.4-10.9 to include
pri-
vate
instance variable
birthDate
in class
Employee
. Use class
Date
of Fig. 8.7 to represent an em-
ployee's birthday. Add
get
methods to class
Date
. Assume that payroll is processed once per month.
Create an array of
Employee
variables to store references to the various employee objects. In a loop,
calculate the payroll for each
Employee
(polymorphically), and add a $100.00 bonus to the person's
payroll amount if the current month is the one in which the
Employee
's birthday occurs.
10.13
(Project:
Shape
Hierarchy)
Implement the
Shape
hierarchy shown in Fig. 9.3. Each
Two-
DimensionalShape
should contain method
getArea
to calculate the area of the two-dimensional
shape. Each
ThreeDimensionalShape
should have methods
getArea
and
getVolume
to calculate the
surface area and volume, respectively, of the three-dimensional shape. Create a program that uses
an array of
Shape
references to objects of each concrete class in the hierarchy. The program should
print a text description of the object to which each array element refers. Also, in the loop that pro-
cesses all the shapes in the array, determine whether each shape is a
TwoDimensionalShape
or a
ThreeDimensionalShape
. If it's a
TwoDimensionalShape
, display its area. If it's a
ThreeDimension-
alShape
, display its area and volume.
10.14
(Payroll System Modification)
Modify the payroll system of Figs. 10.4-10.9 to include an
additional
Employee
subclass
PieceWorker
that represents an employee whose pay is based on the
number of pieces of merchandise produced. Class
PieceWorker
should contain
private
instance
variables
wage
(to store the employee's wage per piece) and
pieces
(to store the number of pieces
produced). Provide a concrete implementation of method
earnings
in class
PieceWorker
that cal-
culates the employee's earnings by multiplying the number of pieces produced by the wage per
piece. Create an array of
Employee
variables to store references to objects of each concrete class in
the new
Employee
hierarchy. For each
Employee
, display its
String
representation and earnings.
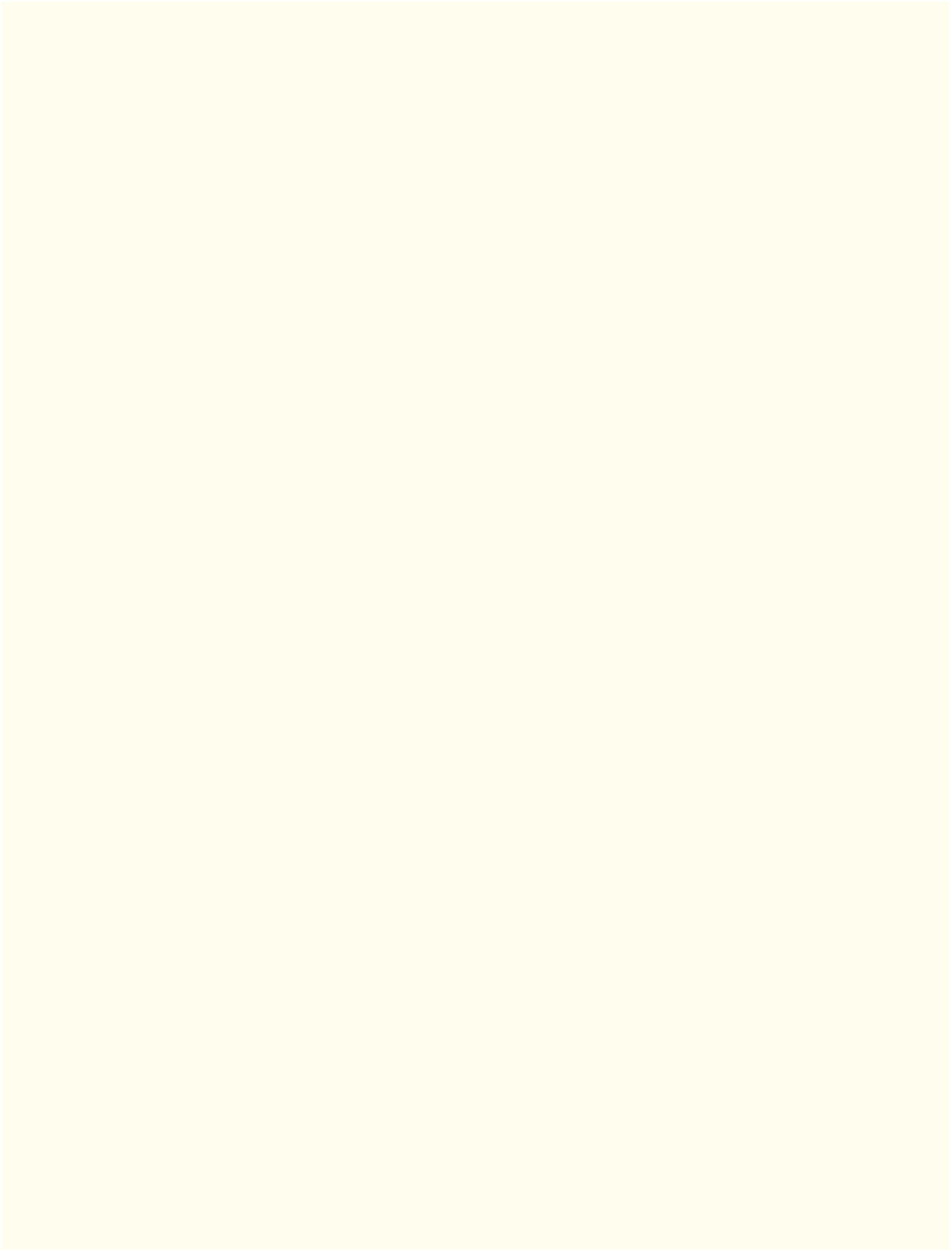