Java Reference
In-Depth Information
has only six elements (with indexes 0-5). Because array bounds checking is performed at
execution time, the JVM generates an
exception
—specifically line 19 throws an
ArrayIndexOutOfBoundsException
to notify the program of this problem. At this point
the
try
block terminates and the
catch
block begins executing—if you declared any local
variables in the
try
block, they're now
out of scope
(and no longer exist), so they're not ac-
cessible in the
catch
block.
The
catch
block declares an exception parameter (
e
) of type (
IndexOutOfRangeEx-
ception
). The
catch
block can handle exceptions of the specified type. Inside the
catch
block, you can use the parameter's identifier to interact with a caught exception object.
Error-Prevention Tip 7.1
When writing code to access an array element, ensure that the array index remains greater
than or equal to 0 and less than the length of the array. This would prevent
ArrayIndex-
OutOfBoundsException
s if your program is correct.
Software Engineering Observation 7.1
Systems in industry that have undergone extensive testing are still likely to contain bugs.
Our preference for industrial-strength systems is to catch and deal with runtime excep-
tions, such as
ArrayIndexOutOfBoundsException
s, to ensure that a system either stays up
and running or degrades gracefully, and to inform the system's developers of the problem.
When lines 21-26
catch
the exception, the program displays a message indicating the
problem that occurred. Line 23
implicitly
calls the exception object's
toString
method to
get the error message that's implicitly stored in the exception object and display it. Once
the message is displayed in this example, the exception is considered
handled
and the pro-
gram continues with the next statement after the
catch
block's closing brace. In this ex-
ample, the end of the for statement is reached (line 27), so the program continues with the
increment of the control variable in line 15. We discuss exception handling again in
Chapter 8, and more deeply in Chapter 11.
The examples in the chapter thus far have used arrays containing elements of primitive
types. Recall from Section 7.2 that the elements of an array can be either primitive types
or reference types. This section uses random-number generation and an array of reference-
type elements, namely objects representing playing cards, to develop a class that simulates
card shuffling and dealing. This class can then be used to implement applications that play
specific card games. The exercises at the end of the chapter use the classes developed here
to build a simple poker application.
We first develop class
Card
(Fig. 7.9), which represents a playing card that has a face
(e.g.,
"Ace"
,
"Deuce"
,
"Three"
, …,
"Jack"
,
"Queen"
,
"King"
) and a suit (e.g.,
"Hearts"
,
"Diamonds"
,
"Clubs"
,
"Spades"
). Next, we develop the
DeckOfCards
class (Fig. 7.10),
which creates a deck of 52 playing cards in which each element is a
Card
object. We then
build a test application (Fig. 7.11) that demonstrates class
DeckOfCards
's card shuffling
and dealing capabilities.


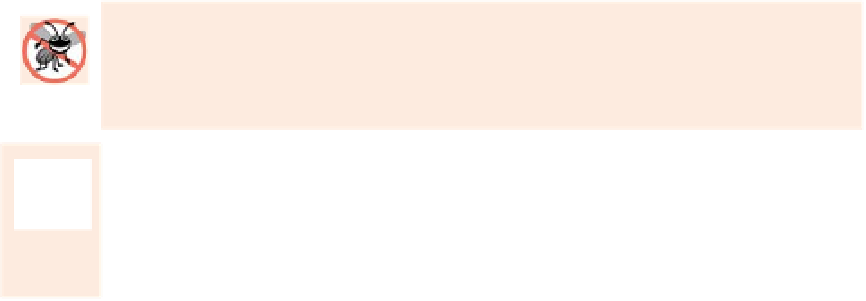


