Java Reference
In-Depth Information
field width of 5, followed by a colon and a space, to align the label
"100: "
with the other
bar labels. The nested
for
statement (lines 23-24) outputs the bars. Note the loop-con-
tinuation condition at line 23 (
stars < array[counter]
). Each time the program reaches
the inner
for
, the loop counts from
0
up to
array[counter]
, thus using a value in
array
to determine the number of asterisks to display. In this example,
no
students received a
grade below 60, so
array[0]
-
array[5]
contain zeroes, and
no
asterisks are displayed next
to the first six grade ranges. In line 19, the format specifier
%02d
indicates that an
int
value
should be formatted as a field of two digits. The
0
flag
in the format specifier displays a
leading
0
for values with fewer digits than the field width (
2
).
Sometimes, programs use counter variables to summarize data, such as the results of a sur-
vey. In Fig. 6.7, we used separate counters in our die-rolling program to track the number
of occurrences of each side of a six-sided die as the program rolled the die 6,000,000 times.
An array version of this application is shown in Fig. 7.7.
1
// Fig. 7.7: RollDie.java
2
// Die-rolling program using arrays instead of switch.
3
import
java.security.SecureRandom;
4
5
public class
RollDie
6
{
7
public static
void
main(String[] args)
8
{
9
SecureRandom randomNumbers =
new
SecureRandom();
10
int
[] frequency =
new
int[
7
];
// array of frequency counters
11
12
// roll die 6,000,000 times; use die value as frequency index
13
for
(
int
roll =
1
; roll <=
6000000
; roll++)
14
++frequency[
1
+ randomNumbers.nextInt(
6
)];
15
16
System.out.printf(
"%s%10s%n"
,
"Face"
,
"Frequency"
);
17
18
// output each array element's value
19
for
(
int
face =
1
; face < frequency.length; face++)
20
System.out.printf(
"%4d%10d%n"
, face, frequency[face]);
21
}
22
}
// end class RollDie
Face Frequency
1 999690
2 999512
3 1000575
4 999815
5 999781
6 1000627
Fig. 7.7
|
Die-rolling program using arrays instead of
switch
.
Figure 7.7 uses the array
frequency
(line 10) to count the occurrences of each side of
the die.
The single statement in line 14 of this program replaces lines 22-45 of Fig. 6.7.
Line
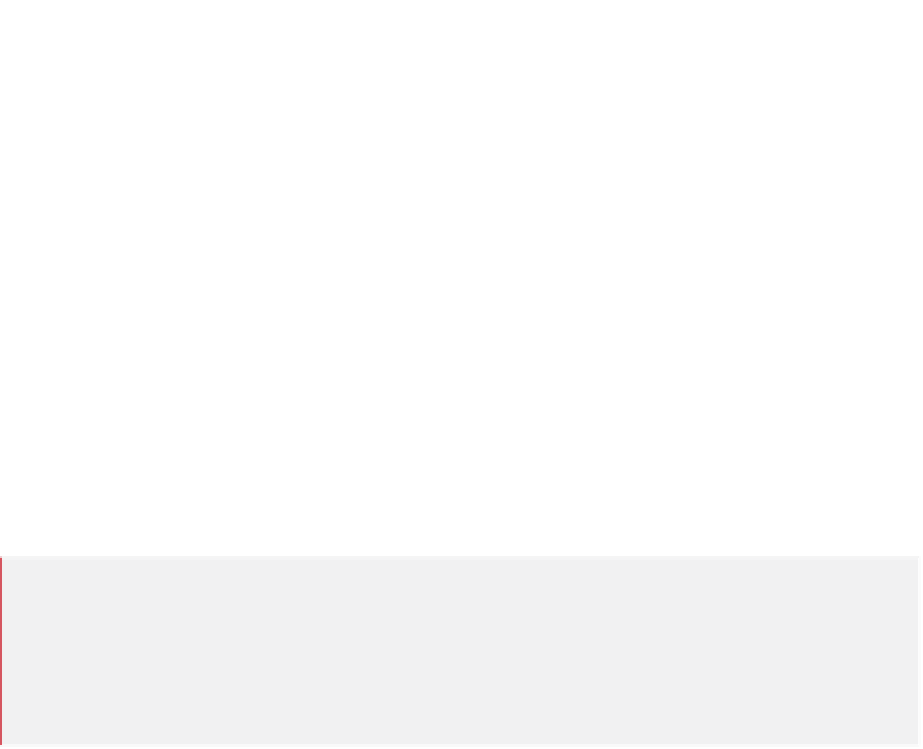






