Java Reference
In-Depth Information
First Drawing Application
Our first drawing application simply draws two lines. Class
DrawPanel
(Fig. 4.18) per-
forms the actual drawing, while class
DrawPanelTest
(Fig. 4.19) creates a window to dis-
play the drawing. In class
DrawPanel
, the
import
statements in lines 3-4 allow us to use
class
Graphics
(from package
java.awt)
, which provides various methods for drawing
text and shapes onto the screen, and class
JPanel
(from package
javax.swing
), which pro-
vides an area on which we can draw.
1
// Fig. 4.18: DrawPanel.java
2
// Using drawLine to connect the corners of a panel.
3
4
5
6
7
import
java.awt.Graphics;
import
javax.swing.JPanel;
public
class
DrawPanel
extends
JPanel
{
8
// draws an X from the corners of the panel
9
public
void
paintComponent(Graphics g)
10
{
11
// call paintComponent to ensure the panel displays correctly
12
super
.paintComponent(g);
13
14
int
width = getWidth();
// total width
int
height = getHeight();
// total height
15
16
17
// draw a line from the upper-left to the lower-right
18
g.drawLine(
0
,
0
, width, height);
19
20
// draw a line from the lower-left to the upper-right
21
g.drawLine(
0
, height, width,
0
);
22
}
23
}
// end class DrawPanel
Fig. 4.18
|
Using
drawLine
to connect the corners of a panel.
1
// Fig. 4.19: DrawPanelTest.java
2
// Creating JFrame to display DrawPanel.
3
4
5
import
javax.swing.JFrame;
public
class
DrawPanelTest
6
{
7
public
static
void
main(String[] args)
8
{
9
// create a panel that contains our drawing
10
DrawPanel panel =
new
DrawPanel();
11
12
// create a new frame to hold the panel
13
JFrame application =
new
JFrame();
14
15
// set the frame to exit when it is closed
16
application.setDefaultCloseOperation(
JFrame.EXIT_ON_CLOSE
);
Fig. 4.19
|
Creating
JFrame
to display
DrawPanel
. (Part 1 of 2.)
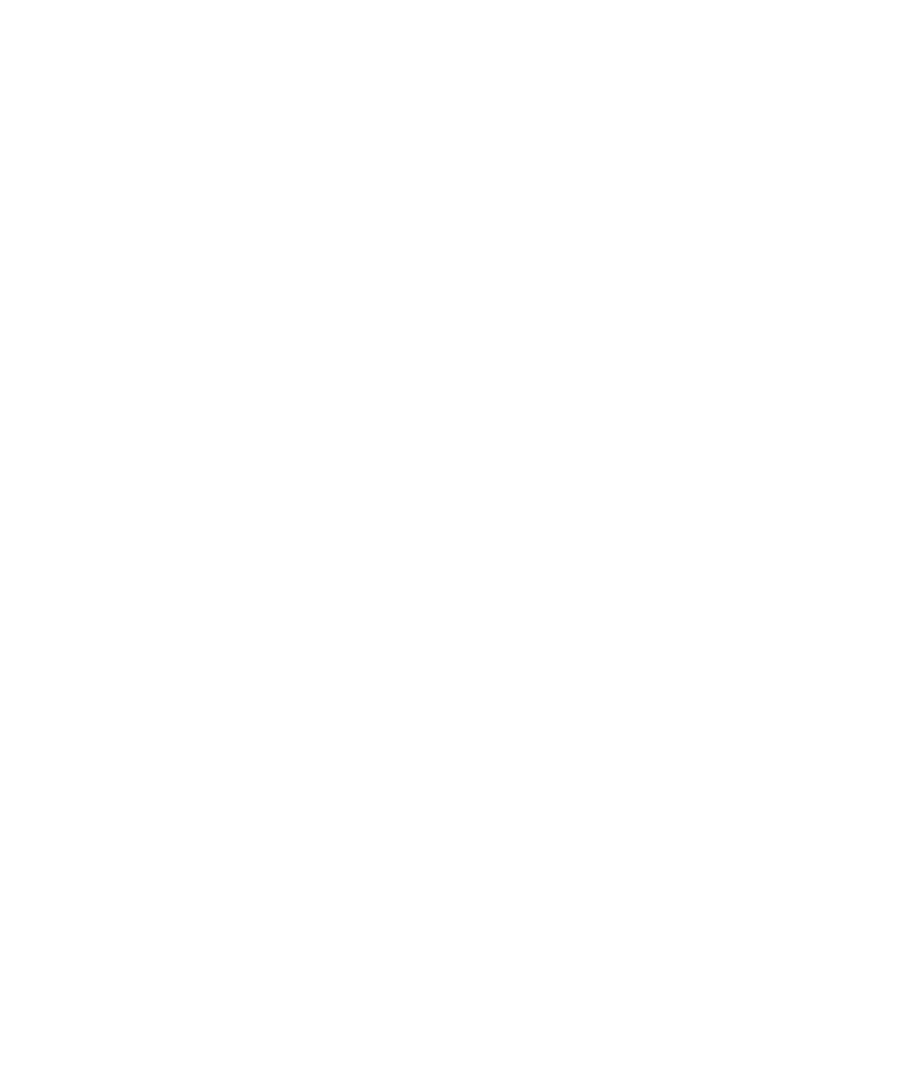

























