Java Reference
In-Depth Information
86
cancelJButton.setEnabled(
false
);
// disable Cancel button
87
88
try
89
{
90
// retrieve and display doInBackground return value
91
statusJLabel.setText(
"Found "
+ get() +
" primes.")
;
92
}
93
catch
(InterruptedException | ExecutionException |
94
CancellationException ex)
95
{
96
statusJLabel.setText(ex.getMessage());
97
}
98
}
99
}
// end class PrimeCalculator
Fig. 23.26
|
Calculates the first
n
primes, displaying them as they are found. (Part 3 of 3.)
Class
PrimeCalculator
extends
SwingWorker
(line 13), with the first type parameter
indicating the return type of method
doInBackground
and the second indicating the type
of intermediate results passed between methods
publish
and
process
. In this case, both
type parameters are
Integer
s. The constructor (lines 23-34) takes as arguments an integer
that indicates the upper limit of the prime numbers to locate, a
JTextArea
used to display
primes in the GUI, one
JButton
for initiating a calculation and one for canceling it, and
a
JLabel
used to display the status of the calculation.
Sieve of Eratosthenes
Line 33 initializes the elements of the
boolean
array
primes
to
true
with
Arrays
method
fill
.
PrimeCalculator
uses this array and the
Sieve of Eratosthenes
algorithm (described
in Exercise 7.27) to find all primes less than
max
. The Sieve of Eratosthenes takes a list of
integers and, beginning with the first prime number, filters out all multiples of that prime.
It then moves to the next prime, which will be the next number that's not yet filtered out,
and eliminates all of its multiples. It continues until the end of the list is reached and all
nonprimes have been filtered out. Algorithmically, we begin with element
2
of the
bool-
ean
array and set the cells corresponding to all values that are multiples of 2 to
false
to
indicate that they're divisible by 2 and thus not prime. We then move to the next array
element, check whether it's
true
, and if so set all of its multiples to
false
to indicate that
they're divisible by the current index. When the whole array has been traversed in this way,
all indices that contain
true
are prime, as they have no divisors.
Method
doInBackground
In method
doInBackground
(lines 37-73), the control variable
i
for the loop (lines 43-
70) controls the current index for implementing the Sieve of Eratosthenes. Line 45 calls
the inherited
SwingWorker
method
isCancelled
to determine whether the user has
clicked the
Cancel
button. If
isCancelled
returns
true
, method
doInBackground
returns
the number of primes found so far (line 46) without finishing the computation.
If the calculation isn't canceled, line 49 calls
setProgress
to update the percentage of
the array that's been traversed so far. Line 53 puts the currently executing thread to sleep
for up to 4 milliseconds. We discuss the reason for this shortly. Line 61 tests whether the
element of array
primes
at the current index is
true
(and thus prime). If so, line 63 passes
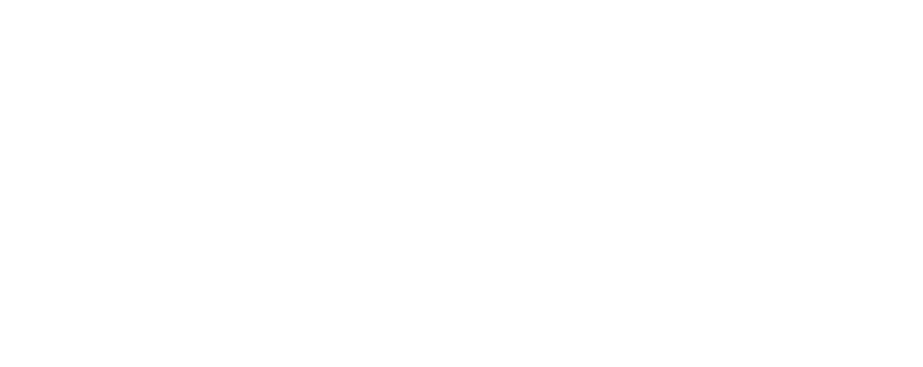

