Java Reference
In-Depth Information
10
public void
start(Stage primaryStage) {
11
// Create a pane
12
Pane pane =
new
Pane();
create a pane
13
14
for
(
int
i =
0
; i <
16
; i++) {
15
// Create an ellipse and add it to pane
16 Ellipse e1 =
new
Ellipse(
150
,
100
,
100
,
50
);
17 e1.setStroke(Color.color(Math.random(), Math.random(),
18 Math.random()));
19 e1.setFill(Color.WHITE);
20
create an ellipse
set random color for stroke
set fill color
rotate ellipse
add ellipse to pane
e1.setRotate(i *
180
/
16
);
21
pane.getChildren().add(e1);
22 }
23
24
// Create a scene and place it in the stage
25 Scene scene =
new
Scene(pane,
300
,
200
);
26 primaryStage.setTitle(
"ShowEllipse"
);
// Set the stage title
27 primaryStage.setScene(scene);
// Place the scene in the stage
28 primaryStage.show();
// Display the stage
29 }
30 }
The program repeatedly creates an ellipse (line 16), sets a random stroke color (lines
17-18), sets its fill color to white (line 19), rotates it (line 20), and adds the rectangle to the
pane (line 21).
14.11.5
Arc
An arc is conceived as part of an ellipse, defined by the parameters
centerX
,
centerY
,
radiusX
,
radiusY
,
startAngle
,
length
, and an arc type (
ArcType.OPEN
,
ArcType
.CHORD
, or
ArcType.ROUND
). The parameter
startAngle
is the starting angle; and
length
is the spanning angle (i.e., the angle covered by the arc). Angles are measured in degrees and
follow the usual mathematical conventions (i.e., 0 degrees is in the easterly direction, and
positive angles indicate counterclockwise rotation from the easterly direction), as shown in
Figure 14.36a.
The
Arc
class defines an arc. The UML diagram for the
Arc
class is shown in Figure 14.35.
Listing 14.18 gives an example that demonstrates ellipses, as shown in Figure 14.36b.
The getter and setter methods for property values
and a getter for property itself are provided in the class,
but omitted in the UML diagram for brevity.
javafx.scene.shape.Arc
-centerX: DoubleProperty
-centerY: DoubleProperty
-radiusX: DoubleProperty
-radiusY: DoubleProperty
-startAngle: DoubleProperty
-length: DoubleProperty
-type: ObjectProperty<ArcType>
The x-coordinate of the center of the ellipse (default
0
).
The y-coordinate of the center of the ellipse (default
0
).
The horizontal radius of the ellipse (default:
0
).
The vertical radius of the ellipse (default:
0
).
The start angle of the arc in degrees.
The angular extent of the arc in degrees.
The closure type of the arc (
ArcType.OPEN, ArcType.CHORD,
ArcType.ROUND
).
+Arc()
+Arc(x: double, y: double,
radiusX: double, radiusY:
double, startAngle: double,
length: double)
Creates an empty
Arc
.
Creates an
Arc
with the specified arguments.
F
IGURE
14.35
The
Arc
class defines an arc.




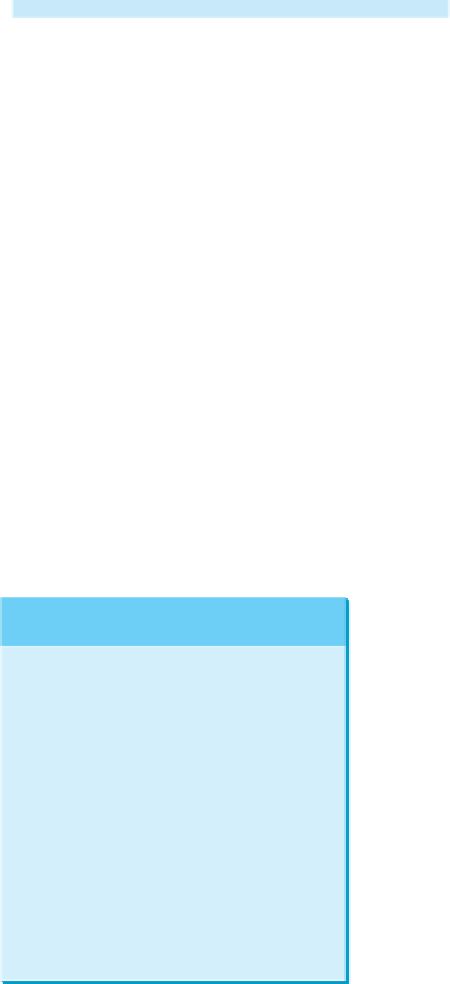
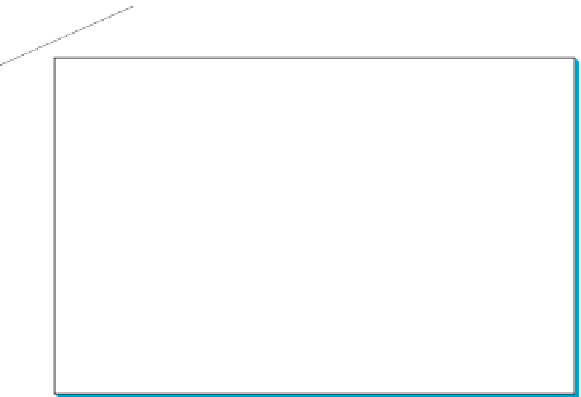





















Search WWH ::

Custom Search