Java Reference
In-Depth Information
4
catch
(MalformedURLException ex) {
5 ex.printStackTrace();
6 }
A
MalformedURLException
is thrown if the URL string has a syntax error. For example,
runtime error because two slashes (
//
) are required after the colon (
:
). Note that the
http://
prefix is required for the
URL
class to recognize a valid URL. It would be wrong if you replace
line 2 with the following code:
URL url =
new
URL(
"www.google.com/index.html"
);
After a
URL
object is created, you can use the
openStream()
method defined in the
URL
class
to open an input stream and use this stream to create a
Scanner
object as follows:
Scanner input =
new
Scanner(url.openStream());
Now you can read the data from the input stream just like from a local file. The example in
Listing 12.17 prompts the user to enter a URL and displays the size of the file.
L
ISTING
12.17
ReadFileFromURL.java
1
import
java.util.Scanner;
2
3
public class
ReadFileFromURL {
4
public static void
main(String[] args) {
5 System.out.print(
"Enter a URL: "
);
6
String URLString =
new
Scanner(System.in).next();
enter a URL
7
8
try
{
9 java.net.URL url =
new
java.net.URL(URLString);
10
int
count =
0
;
11 Scanner input =
new
Scanner(url.openStream());
12
while
(input.hasNext()) {
13 String line = input.nextLine();
14 count += line.length();
15 }
16
17 System.out.println(
"The file size is "
+ count +
" characters"
);
18 }
19
catch
(java.net.MalformedURLException ex) {
20 System.out.println(
"Invalid URL"
);
21 }
22
catch
(java.io.IOException ex) {
23 System.out.println(
"I/O Errors: no such file"
);
24 }
25 }
26 }
create a
URL
object
create a
Scanner
object
more to read?
read a line
MalformedURLException
IOException
Enter a URL: http://cs.armstrong.edu/liang/data/Lincoln.txt
The file size is 1469 characters
Enter a URL: http://www.yahoo.com
The file size is 190006 characters




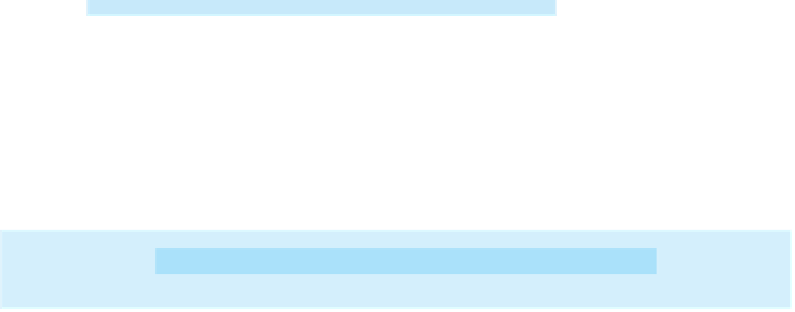



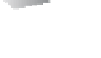








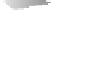




























Search WWH ::

Custom Search