Java Reference
In-Depth Information
Note
Arrays are objects in Java (objects are introduced in ChapterĀ 9). The JVM stores the
objects in an area of memory called the
heap
, which is used for dynamic memory
allocation.
ListingĀ 7.3 gives another program that shows the difference between passing a primitive data
type value and an array reference variable to a method.
The program contains two methods for swapping elements in an array. The first method,
named
swap
, fails to swap two
int
arguments. The second method, named
swapFirst-
TwoInArray
, successfully swaps the first two elements in the array argument.
heap
L
ISTING
7.3
TestPassArray.java
1
public class
TestPassArray {
2
/** Main method */
3
public static void
main(String[] args) {
4
int
[] a = {
1
,
2
};
5
6
// Swap elements using the swap method
7 System.out.println(
"Before invoking swap"
);
8 System.out.println(
"array is {"
+ a[
0
] +
", "
+ a[
1
] +
"}"
);
9 swap(a[
0
], a[
1
]);
10 System.out.println(
"After invoking swap"
);
11 System.out.println(
"array is {"
+ a[
0
] +
", "
+ a[
1
] +
"}"
);
12
13
// Swap elements using the swapFirstTwoInArray method
14 System.out.println(
"Before invoking swapFirstTwoInArray"
);
15 System.out.println(
"array is {"
+ a[
0
] +
", "
+ a[
1
] +
"}"
);
16 swapFirstTwoInArray(a);
17 System.out.println(
"After invoking swapFirstTwoInArray"
);
18 System.out.println(
"array is {"
+ a[
0
] +
", "
+ a[
1
] +
"}"
);
19 }
20
21
/** Swap two variables */
22
public static void
swap(
int
n1,
int
n2) {
23
int
temp = n1;
24 n1 = n2;
25 n2 = temp;
26 }
27
28
/** Swap the first two elements in the array */
29
public static void
swapFirstTwoInArray(
int
[] array) {
30
int
temp = array[
0
];
31 array[
0
] = array[
1
];
32 array[
1
] = temp;
33 }
34 }
false swap
swap array elements
Before invoking swap
array is {1, 2}
After invoking swap
array is {1, 2}
Before invoking swapFirstTwoInArray
array is {1, 2}
After invoking swapFirstTwoInArray
array is {2, 1}







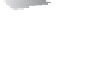






















Search WWH ::

Custom Search