Java Reference
In-Depth Information
Note
The preceding statement creates an array using the following syntax:
new
elementType[]{value0, value1, ..., value
k
};
There is no explicit reference variable for the array. Such array is called an
anonymous
array
.
anonymous array
Java uses
pass-by-value
to pass arguments to a method. There are important differences
between passing the values of variables of primitive data types and passing arrays.
pass-by-value
For an argument of a primitive type, the argument's value is passed.
■
For an argument of an array type, the value of the argument is a reference to an array;
this reference value is passed to the method. Semantically, it can be best described as
pass-by-sharing
, that is, the array in the method is the same as the array being passed.
Thus, if you change the array in the method, you will see the change outside the method.
■
pass-by-sharing
Take the following code, for example:
public class
Test {
public static void
main(String[] args) {
int
x =
1
;
// x represents an int value
int
[] y =
new int
[
10
];
// y represents an array of int values
m(x, y);
// Invoke m with arguments x and y
System.out.println(
"x is "
+ x);
System.out.println(
"y[0] is "
+ y[
0
]);
}
public static void
m(
int
number,
int
[] numbers) {
number =
1001
;
// Assign a new value to number
numbers[
0
] =
5555
;
// Assign a new value to numbers[0]
}
}
x is 1
y[0] is 5555
You may wonder why after
m
is invoked,
x
remains
1
, but
y[0]
become
5555
. This is
because
y
and
numbers
, although they are independent variables, reference the same array, as
illustrated in Figure 7.5. When
m(x, y)
is invoked, the values of
x
and
y
are passed to
num-
ber
and
numbers
. Since
y
contains the reference value to the array,
numbers
now contains
the same reference value to the same array.
Stack
Heap
Activation record for
method
m
int[]
numbers:
int
number: 1
reference
Arrays are
stored in a
heap.
An array of
ten
int
values is
stored here
Activation record for the
main method
int[] y
:
int x
:1
reference
F
IGURE
7.5
The primitive type value in
x
is passed to
number
, and the reference value in
y
is passed to
numbers
.










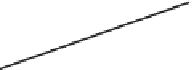






























Search WWH ::

Custom Search