Java Reference
In-Depth Information
The
Thread
class contains the constructors for creating threads for tasks and the
methods for controlling threads.
Key
Point
Figure 30.4 shows the class diagram for the
Thread
class.
«interface»
java.lang.Runnable
java.lang.Thread
+Thread()
Creates an empty thread.
+Thread(task: Runnable)
Creates a thread for a specified task.
+start(): void
Starts the thread that causes the
run()
method to be invoked by the JVM.
+isAlive(): boolean
+setPriority(p: int): void
+join(): void
Tests whether the thread is currently running.
Sets priority
(ranging from 1 to 10) for this thread.
Waits for this thread to finish.
Puts a thread to sleep for a specified time in milliseconds.
Causes a thread to pause temporarily and allow other threads to execute.
p
+sleep(millis: long): void
+yield(): void
+interrupt(): void
Interrupts this thread.
F
IGURE
30.4
The
Thread
class contains the methods for controlling threads.
Note
Since the
Thread
class implements
Runnable
, you could define a class that extends
Thread
and implements the
run
method, as shown in Figure 30.5a, and then create
an object from the class and invoke its
start
method in a client program to start the
thread, as shown in Figure 30.5b.
separating task from thread
java.lang.Thread
CustomThread
// Client class
public class
Client {
...
public void
// Custom thread class
public class
someMethod() {
CustomThread extends Thread {
...
// Create a thread
CustomThread thread1 =
...
public CustomThread(...) {
...
}
new
CustomThread(...);
// Start a thread
thread1.start();
...
// Override the run method in Runnable
public void run() {
// Tell system how to perform this task
...
}
...
}
// Create another thread
CustomThread thread2 =
new
CustomThread(...);
// Start a thread
thread2.start();
}
...
}
(a)
(b)
F
IGURE
30.5
Define a thread class by extending the
Thread
class.


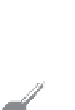


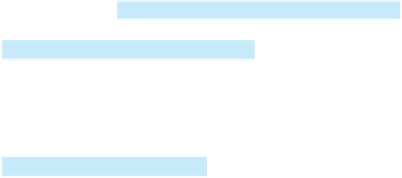
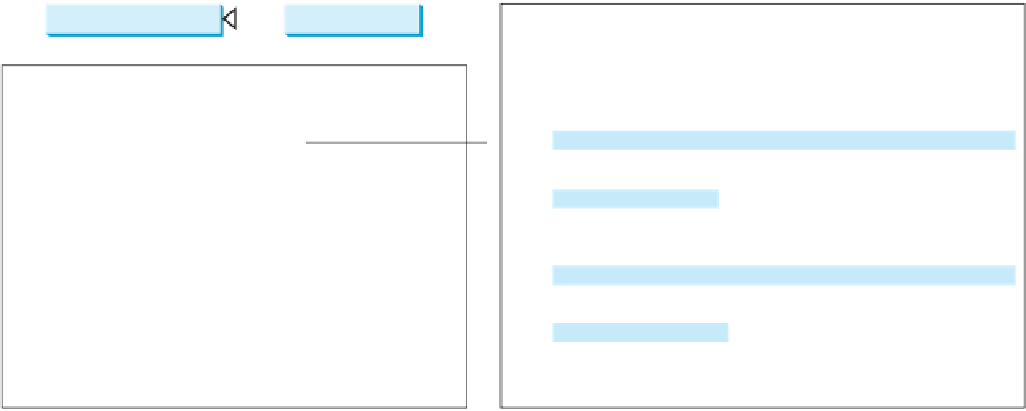
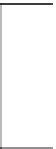

























Search WWH ::

Custom Search