Java Reference
In-Depth Information
The program creates three tasks (lines 4-6). To run them concurrently, three threads are created
(lines 9-11). The
start()
method (lines 14-16) is invoked to start a thread that causes the
run()
method in the task to be executed. When the
run()
method completes, the thread
terminates.
Because the first two tasks,
printA
and
printB
, have similar functionality, they
can be defined in one task class
PrintChar
(lines 21-41). The
PrintChar
class imple-
ments
Runnable
and overrides the
run()
method (lines 36-40) with the print-character
action. This class provides a framework for printing any single character a given number
of times. The runnable objects,
printA
and
printB
, are instances of the
PrintChar
class.
The
PrintNum
class (lines 44-58) implements
Runnable
and overrides the
run()
method (lines 53-57) with the print-number action. This class provides a framework for print-
ing numbers from
1
to
n
, for any integer
n
. The runnable object
print100
is an instance of
the class
printNum
class.
Note
If you don't see the effect of these three threads running concurrently, increase the
number of characters to be printed. For example, change line 4 to
effect of concurrency
Runnable printA =
new
PrintChar(
'a'
, 10000);
Important Note
The
run()
method in a task specifies how to perform the task. This method is auto-
matically invoked by the JVM. You should not invoke it. Invoking
run()
directly merely
executes this method in the same thread; no new thread is started.
run()
method
30.3
✓
✓
How do you define a task class? How do you create a thread for a task?
Check
30.4
What would happen if you replace the
start()
method with the
run()
method in
lines 14-16 in Listing 30.1?
Point
print100.start();
printA.start();
printB.start();
print100.run();
printA.run();
printB.run();
Replaced by
30.5
What is wrong in the following two programs? Correct the errors.
public class
Test
implements
Runnable {
public static void
main(String[] args) {
new
Test();
public class
Test
implements
Runnable {
public static void
main(String[] args) {
new
Test();
}
}
public
Test() {
Test task =
new
Test();
new
Thread(task).start();
}
public
Test() {
Thread t =
new
Thread(
this
);
t.start();
t.start();
}
public void
run() {
System.out.println(
"test"
);
}
}
public void
run() {
System.out.println(
"test"
);
}
}
(a)
(b)




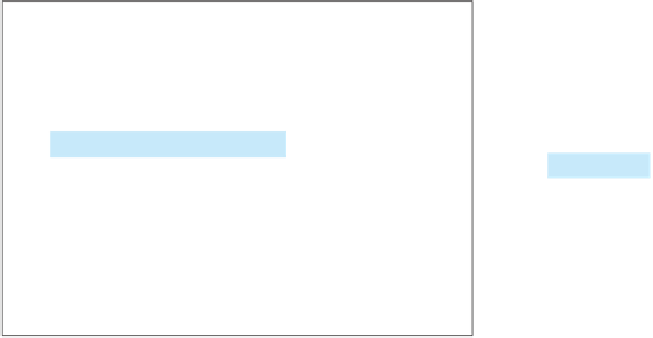

















Search WWH ::

Custom Search