Java Reference
In-Depth Information
Deserializing an Object
The following DeserializeDemo program deserializes the Employee object cre-
ated in the SerializeDemo program. Study the program and try to determine
its output, which is shown in Figure 16.9.
import java.io.*;
public class DeserializeDemo
{
public static void main(String [] args)
{
Employee e = null;
try
{
FileInputStream fileIn =
new FileInputStream(“employee.ser”);
ObjectInputStream in = new ObjectInputStream(fileIn);
e = (Employee) in.readObject();
in.close();
fileIn.close();
}catch(IOException i)
{
i.printStackTrace();
return;
}catch(ClassNotFoundException c)
{
System.out.println(“Employee class not found”);
c.printStackTrace();
return;
}
System.out.println(“Just deserialized Employee...”);
System.out.println(“Name: “ + e.name);
System.out.println(“Address: “ + e.address);
System.out.println(“SSN: “ + e.SSN);
System.out.println(“Number: “ + e.number);
}
}
Figure 16.9
Output of the DeserializeDemo program.

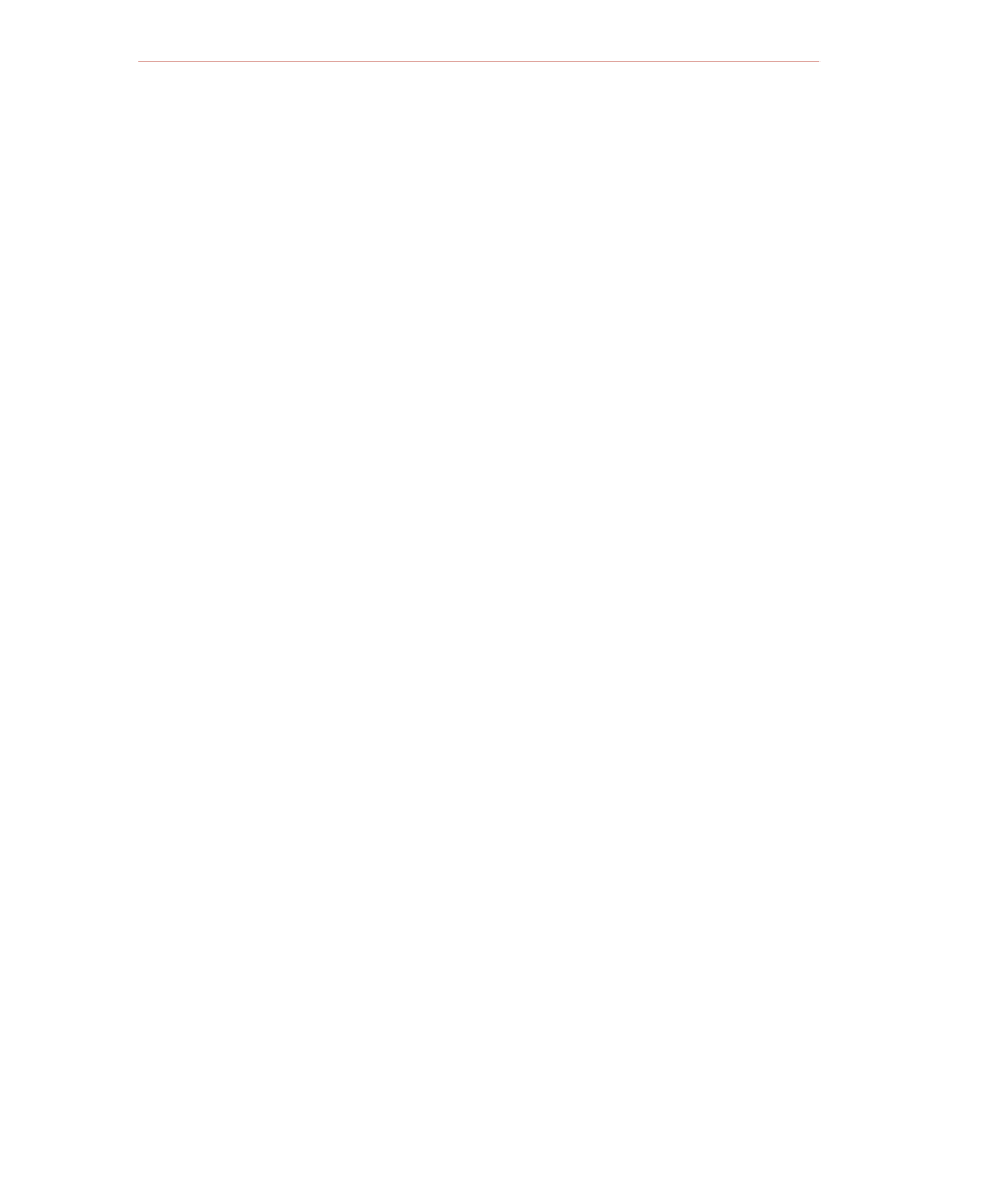
