Java Reference
In-Depth Information
Serializing an Object
The ObjectOutputStream class is used to serialize an Object. The following
SerializeDemo program instantiates an Employee object and serializes it to a
file. When the program is done executing, a file named employee.ser is cre-
ated. The program does not generate any output, but study the code and try to
determine what the program is doing.
When serializing an object to a file, the standard convention in Java is to
give the file a .ser extension.
import java.io.*;
public class SerializeDemo
{
public static void main(String [] args)
{
Employee e = new Employee();
e.name = “Neil Young”;
e.address = “Mobile, AL”;
e.SSN = 11122333;
e.number = 101;
try
{
FileOutputStream fileOut =
new FileOutputStream(“employee.ser”);
ObjectOutputStream out =
new ObjectOutputStream(fileOut);
out.writeObject(e);
out.close();
fileOut.close();
}catch(IOException i)
{
i.printStackTrace();
}
}
}

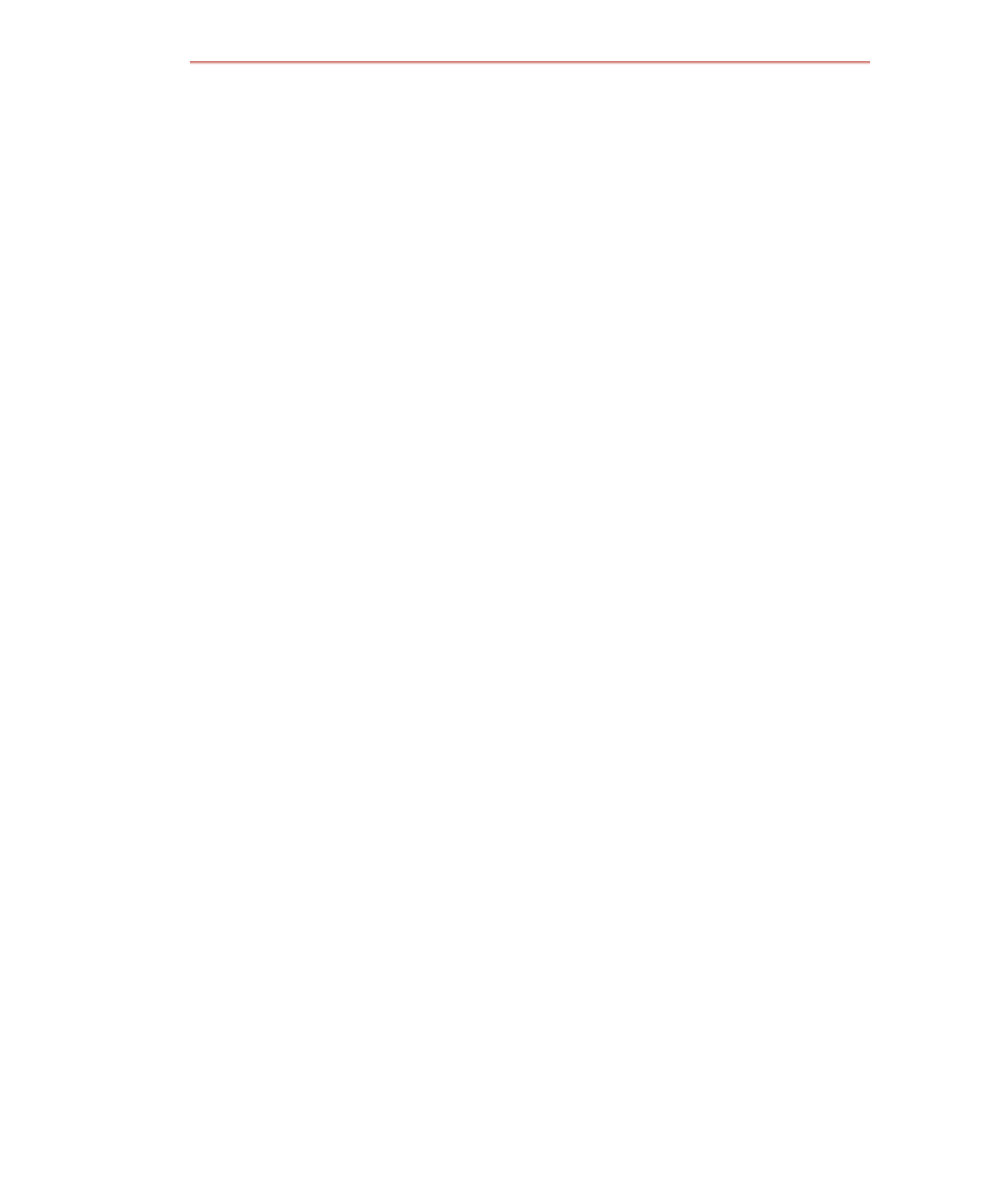
