Java Reference
In-Depth Information
The following program demonstrates a better example of when to use a
while
loop
because it executes an indeterminate number of times. Examine the code and see if you can
determine the result:
1. public class RollDice {
2. public static int rollDice() {
3. return ((int) (Math.random() * 6)) + 1;
4. }
5.
6. public static void main(String [] args) {
7. int one = rollDice();
8. int two = rollDice();
9. System.out.print(“You rolled a “ + (one + two));
10. while(one + two != 11) {
11. one = rollDice();
12. two = rollDice();
13. System.out.print(“, “ + (one + two));
14. }
15. }
16.}
The
while
loop on line 10 executes until the two variables
one
and
two
add up to
11
.
Because they are randomly generated, this could happen right away or it could take a while.
A sample output follows:
You rolled a 7, 8, 9, 6, 11
The output changes every time you run
RollDice
because it uses randomly generated
numbers.
You can easily write an infi nite loop with a
while
statement:
13. while(true) {
14. System.out.println(“This could take a while.”);
15. }
In this example, line 14 will print
This could take a while.
until the user terminates
the JVM.
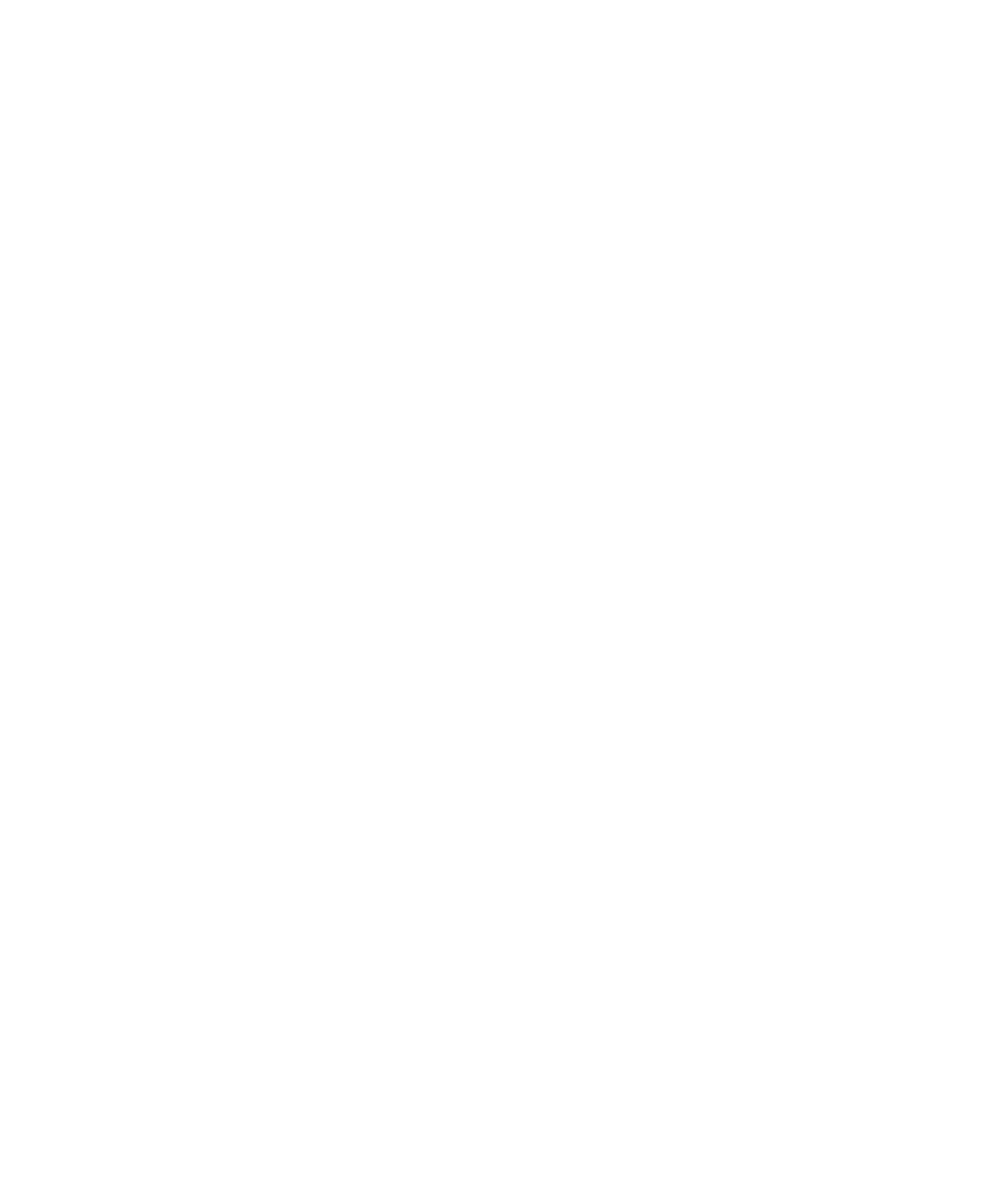




Search WWH ::

Custom Search