Java Reference
In-Depth Information
Because
c
is 0, the expression
c != 0
is
false
and evaluation stops. The variable
three
is
false
and this code does not throw an exception at runtime.
Short-Circuit Behavior
Watch for the short-circuit behavior on the exam. The exam question might alter a vari-
able in the right operand. For example, what is the output of the following code?
int x = 6;
boolean answer = (x >= 6) || (++x <= 7);
System.out.println(x);
Because
x >= 6
is true, the incrementing of
x
does not occur in the right operand, so the
output of this code is 6.
The Conditional Operator
Java contains a
conditional operator
? :
, often referred to as the
ternary operator
because
it is the only operator in Java that has three operands. The syntax for the conditional
operator is
boolean_expression
?
true_expression
:
false_expression
The fi rst operand must be a
boolean
expression. If this
boolean
expression is
true
, then
the second operand is chosen; otherwise, the third operand is chosen. The second and third
operands can be any expressions that evaluate to a value, or any method calls that return a
value.
The conditional operator is a condensed version of an if/else statement that can be handy
in a lot of different situations, especially when outputting or displaying data. For example,
what is the output of the following statements?
int x = 6;
System.out.println( x != 0 ? 10/x : 0);
Because
x
is not 0, the output is the result of
10 / 6
, which is 1.
Let's look at another example. What is the output of the following statements?
double d = 0.36;
System.out.println( d > 0 && d < 1 ? d *= 100 : “not a percent”);
Because
d
is between 0 and 1, the output is 36.0. There is no requirement that the
second and third operands be the same data types (or even compatible types).
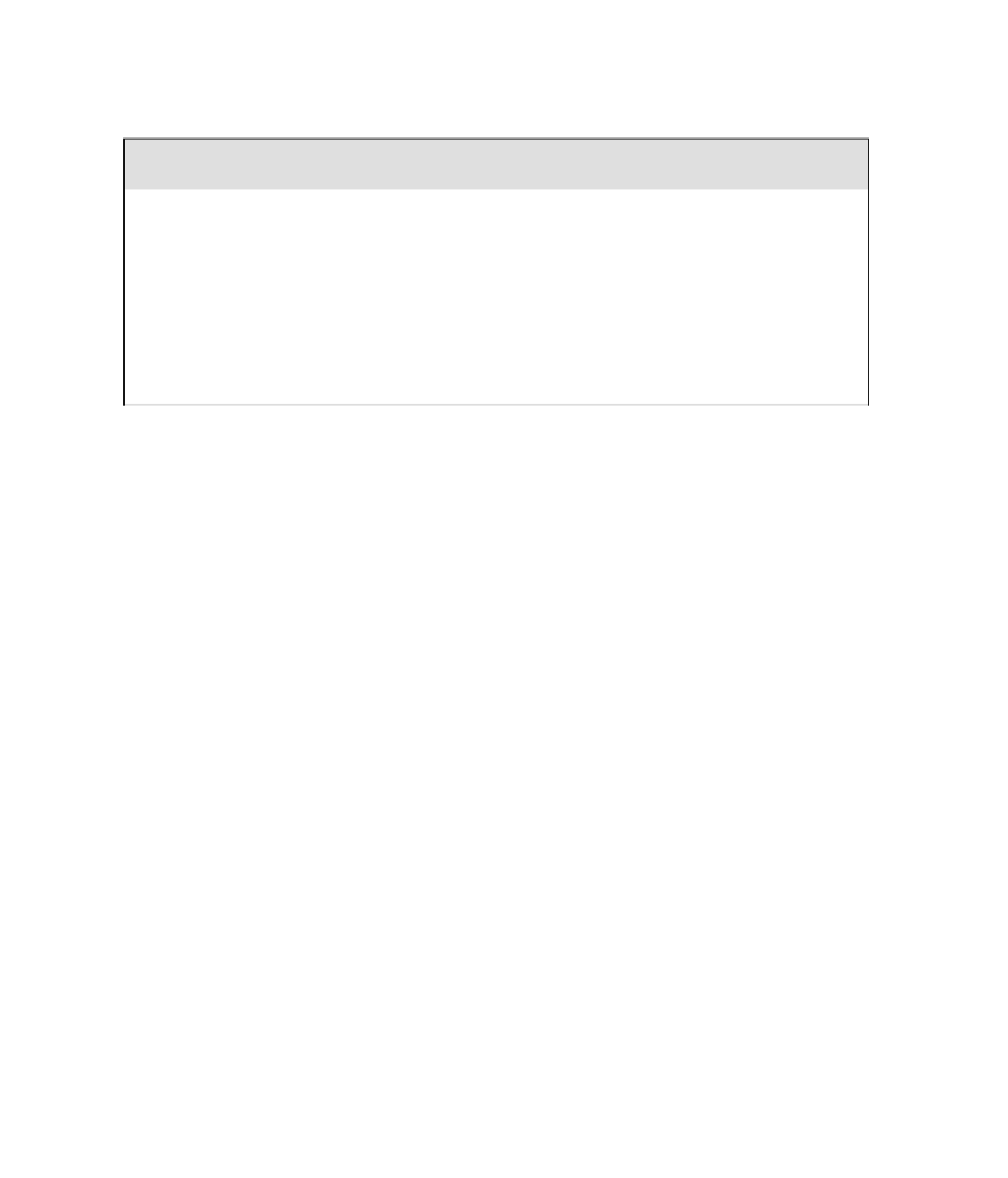




Search WWH ::

Custom Search