Java Reference
In-Depth Information
FIGURE 1.15
Computing the exclusive or expression
12^45
12
45
12^45
0000 1100
0010 1101
0010 0001
The result is 00100001 in binary, which is 33 in decimal. Therefore, the value of
result
is 33.
The
&
,
^
, and
|
are also logical operators, meaning they can operate on
boolean
types.
The result of each operator is identical to Table 1.2 if you were to replace each 0 with
false
and each 1 with
true
. For example, the AND operator
&
is only
true
when both operands
are
true
. The inclusive OR operator
|
is only
false
when both operands are
false
. The
exclusive OR is only
true
when the two operands are different.
What is the output of the following logical statements?
3. int a = 5, b = 10, c = 0;
4. boolean one = a < b & c != 0;
5. System.out.println(one);
6. boolean two = true | true & false;
7. System.out.println(two);
8. boolean three = (c != 0) & (a / c > 1);
9. System.out.println(three);
The variable
one
on line 4 is the result of
true & false
, which is
false
. The result of
two
on line 6 might surprise you. The
&
operator has a higher precedence than
|
, so the
true & false
is evaluated fi rst, which results in
false
. Then
true | false
is evaluated,
which is
true
, so
two
evaluates to
true
.
You might think that the Boolean on line 8 evaluates to
false
, but that line of code
actually throws an
ArithmeticException
when attempting to compute
a / c
. The value
of
c
is 0 and integer division by 0 is undefi ned in Java. Therefore, the last
println
never
executes.
The example of
a / c
is a typical situation where a conditional operator comes in
handy. The conditional operators
&&
and
||
short-circuit, meaning the right operand may
not get evaluated if the left hand operand can determine the result.
For example, when using
&&
, if the left operand is
false
, there is no need to check the
right operand. False AND anything is
false
. In this case, the right-hand expression is not
evaluated. Similarly, when using
||
, if the left operand is
true
, there is no need to check the
right operand because true OR anything is
true
.
The following statements are a modifi cation of the previous example, except this
time the logical expression short-circuits. What is the value of
three
after the following
statements?
21. int a = 5, b = 10, c = 0;
22. boolean three = (c != 0) && (a / c > 1);
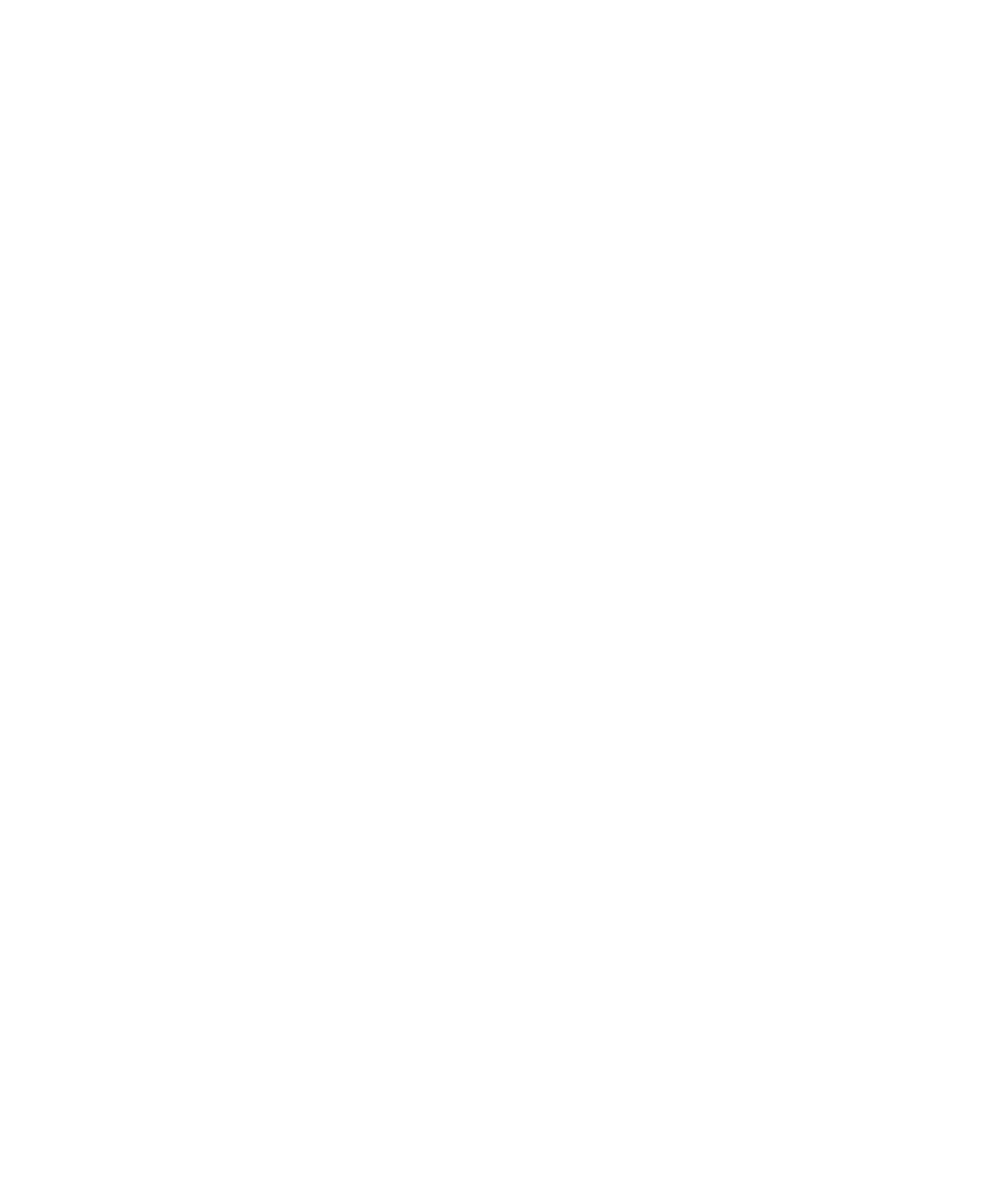





Search WWH ::

Custom Search