Java Reference
In-Depth Information
For clarity's sake, your authors opt to use longer, but easier to follow, approaches to code as opposed to
their shorthand equivalents.
Operating on Elements — The forEach() and map() Methods
The fi nal two methods are the forEach() and map() methods. Unlike the previous iterative methods,
these two methods do not test each element in the array with your function; instead, the function you write
should perform some kind of operation that uses the element in some way. Look at the following code:
var numbers = new Array(1, 2, 3, 4, 5);
for (var i = 0; i < numbers.length; i++ )
{
var result = numbers[i] * 2;
alert(result);
}
As a programmer, you'll often see and use this type of code. It defi nes an array of numbers and loops
through it to perform some kind of operation on each element. In this case, the value of each element
is doubled, and the result is shown in an alert box to the user. Wouldn't it be great if you could do this
without writing a loop?
With the forEach() method, you can. All you need to do is write a function to double a given value
and output the result in an alert box, like this:
var numbers = new Array(1, 2, 3, 4, 5);
function doubleAndAlert(value, index, array)
{
var result = value * 2;
alert(result);
}
numbers.forEach(doubleAndAlert);
Notice that the doubleAndAlert() function doesn't return a value like the testing methods. It cannot
return any value; its only purpose is to perform an operation on every element in the array. While this
is useful in some cases, it's almost useless when you want the results of the operation. That's where the
map() method comes in.
The premise of the map() method is similar to that of forEach(), except that the results of every opera-
tion are stored in another array that the map() method returns.
Let's modify the previous example. The doubleAndAlert() function still needs to double the array
element's value, but it now needs to return the result of that operation in order to be stored in map()'s
returning array.
var numbers = new Array(1, 2, 3, 4, 5);
function doubleAndAlert(value, index, array)
{
var result = value * 2;


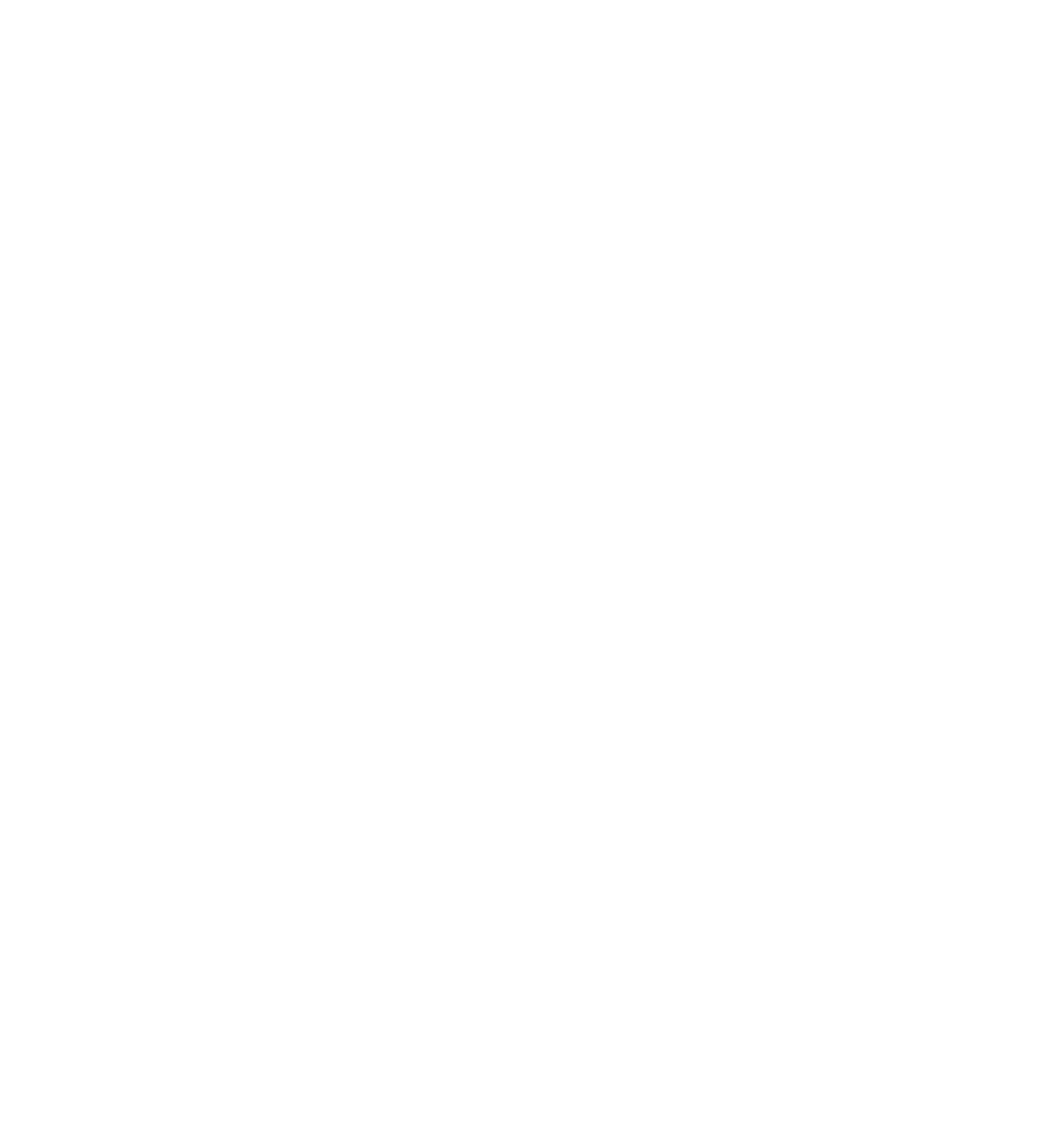



