Java Reference
In-Depth Information
Noncompliant Code Example
This noncompliant code example declares two distinct
String
objects that contain the
same value:
public class StringComparison {
public static void main(String[] args) {
String str1 = new String("one");
String str2 = new String("one");
System.out.println(str1 == str2); // Prints "false"
}
}
The reference equality operator
==
evaluates to
true
only when the values it compares
refer to the same underlying object. The references in this example are unequal because
they refer to distinct objects.
Compliant Solution (
Object.equals()
)
This compliant solution uses the
Object.equals()
method when comparing string val-
ues:
public class StringComparison {
public static void main(String[] args) {
String str1 = new String("one");
String str2 = new String("one");
System.out.println(str1.equals(str2)); // Prints "true"
}
}
Compliant Solution (
String.intern()
)
Reference equality behaves like abstract object equality when it is used to compare two
strings that are results of the
String.intern()
method. This compliant solution uses
String.intern()
and can perform fast string comparisons when only one copy of the
string
one
is required in memory.
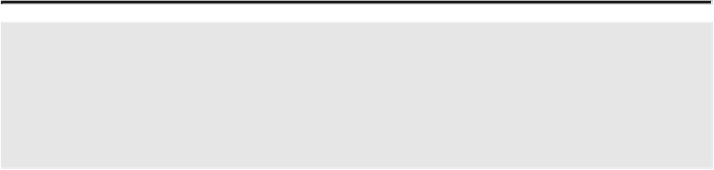


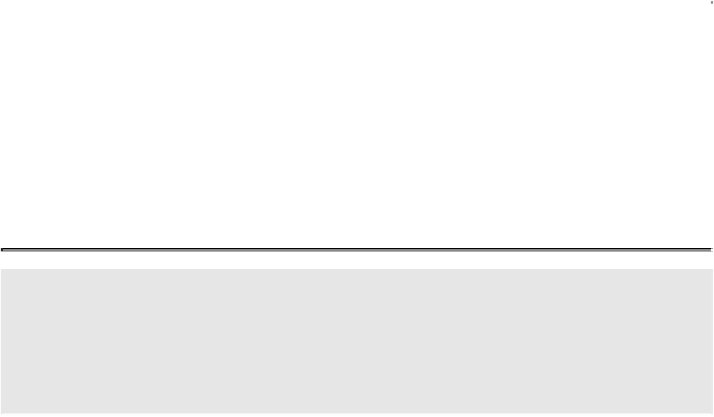




