Java Reference
In-Depth Information
// Unary minus will succeed without overflow
// because temp cannot be Integer.MIN_VALUE
return (temp < 0) ? -temp : temp;
}
public int lookup(int hashKey) {
return hash[imod(hashKey, SIZE)];
}
Applicability
Incorrectly assuming a positive remainder from a remainder operation can result in erro-
neous code.
Bibliography
[JLS 2013]
§15.17.3, “Remainder Operator
%
”
69. Do not confuse abstract object equality with reference equality
Java defines the equality operators
==
and
!=
for testing reference equality, but uses the
equals()
method defined in
Object
and its subclasses for testing abstract object equal-
ity. Naïve programmers often confuse the intent of the
==
operation with that of the
Ob-
ject.equals()
method.Thisconfusionisfrequentlyevidentinthecontextofprocessing
String
objects.
As a general rule, use the
Object.equals()
method to check whether two objects
have equivalent contents, and use the equality operators
==
and
!=
to test whether two
references specifically refer to
the same object
. This latter test is referred to as
referential
equality
. For classes that require overriding the default
equals()
implementation, care
must be taken to also override the
hashCode()
method (see
The CERT
®
Oracle
®
Secure
Coding Standard for Java
™
[Long 2012], “MET09-J. Classes that define an
equals()
method must also define a
hashCode()
method”).
Numeric boxed types (for example,
Byte
,
Character
,
Short
,
Integer
,
Long
,
Float
,
and
Double
) should also be compared using
Object.equals()
, rather than the
==
oper-
ator. While reference equality may appear to work for
Integer
values between the range
−128and127,itmayfailifeitheroftheoperandsinthecomparisonareoutsidethatrange.
Numeric relational operators other than equality (such as
<
,
<=
,
>
, and
>=
) can be safely
used to compare boxed primitive types (see “
EXP03-J. Do not use the equality operators
when comparing values of boxed primitives
”
[Long 2012], for more information).
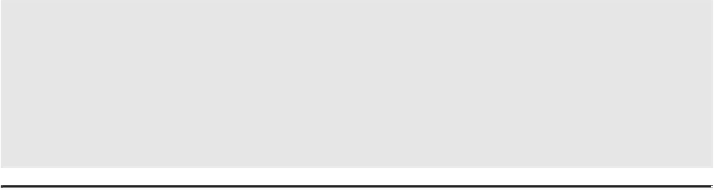
