Java Reference
In-Depth Information
To match our
ArrayList
class and to be able to implement the
Iterable
interface
that allows us to use for-each loops, we need to implement an iterator for our
LinkedList
class. Just as we did with
ArrayList
, we can make the iterator class an
inner class that has access to the fields of the outer object. In the case of the
ArrayList
, the primary field for our iterator was an integer index. For a linked list,
we want to keep track of a current list node. We still need the
removeOK
field that we
used for
ArrayList
. So our iterator class begins as follows:
private class LinkedIterator implements Iterator<E> {
private ListNode<E> current; // location of next value to return
private boolean removeOK; // whether it's okay to remove now
...
}
Notice that our node class is now also generic (
ListNode<E>
). As in the last chap-
ter, we do not declare our class as
LinkedIterator<E>
, because the type
E
is
already declared as part of the outer linked list class. But we do place an
<E>
type
parameter after the
Iterator
and
ListNode
declarations.
To implement the iterator class, you have to take into consideration the fact that
you will have two dummy nodes, one at the front of the list and one at the back. The
first node you will want to process will come after that dummy node at the front, so
the proper initialization for your iterator is as follows:
public LinkedIterator() {
current = front.next;
removeOK = false;
}
To test whether you have a next value to examine, you will use the dummy nodes
again. You'll want to continue to move
current
forward until it reaches the dummy
node at the end of the list. That means that you'll implement
hasNext
as follows:
public boolean hasNext() {
return current != back;
}
For the other two operations, you can simply translate what you did with the array
implementation into appropriate code for your linked list implementation. For the
next
method, your code will look like the following:
public E next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
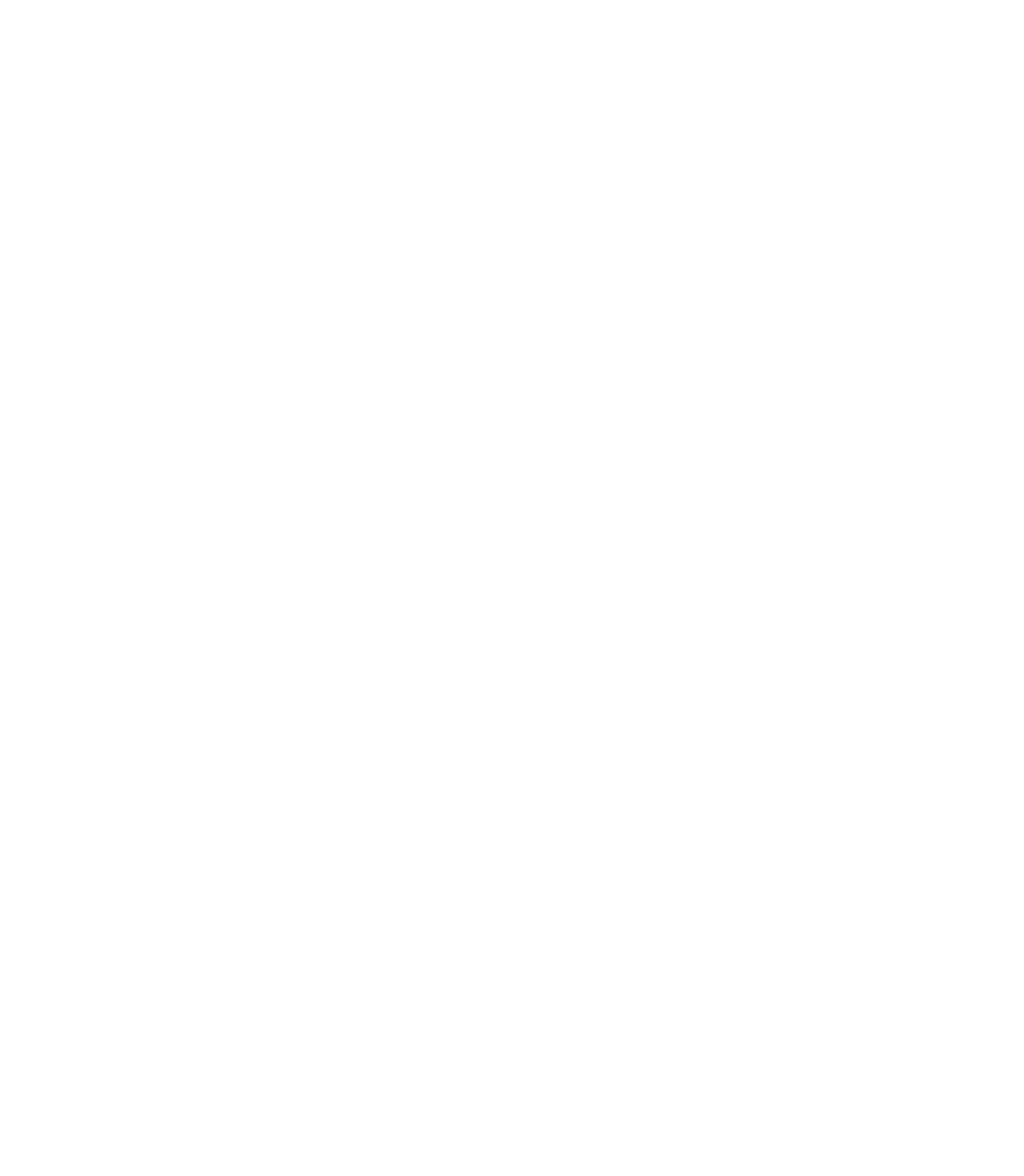
Search WWH ::

Custom Search