Java Reference
In-Depth Information
current = current.next;
}
prev.next = new ListNode(value, prev.next);
}
}
Yet another variation is to set
prev
equal to
null
initially in order to eliminate the
special case for the front of the list. Then you have to test after the loop to see
whether
prev
is still
null
:
public void addSorted(int value) {
ListNode prev = null;
ListNode current = front;
while (current != null && current.data < value) {
prev = current;
current = current.next;
}
if (prev == null) {
front = new ListNode(value, front);
} else {
prev.next = new ListNode(value, prev.next);
}
}
The
ArrayIntList
class from Chapter 15 and the
LinkedIntList
class we have
explored in this chapter have very similar methods. They each have the following
methods:
•a
size
method
•a
get
method
•a
toString
method
• an
indexOf
method
• a one-argument
add
method (the appending add)
• a two-argument
add
method (add at an index)
•a
remove
method (remove at an index)
This similarity isn't an accident. When we began studying linked lists, we pur-
posely implemented new versions of these methods that worked for linked lists. The
point is that these classes are similar in terms of what they can do but they are very
different in the way that they do it.
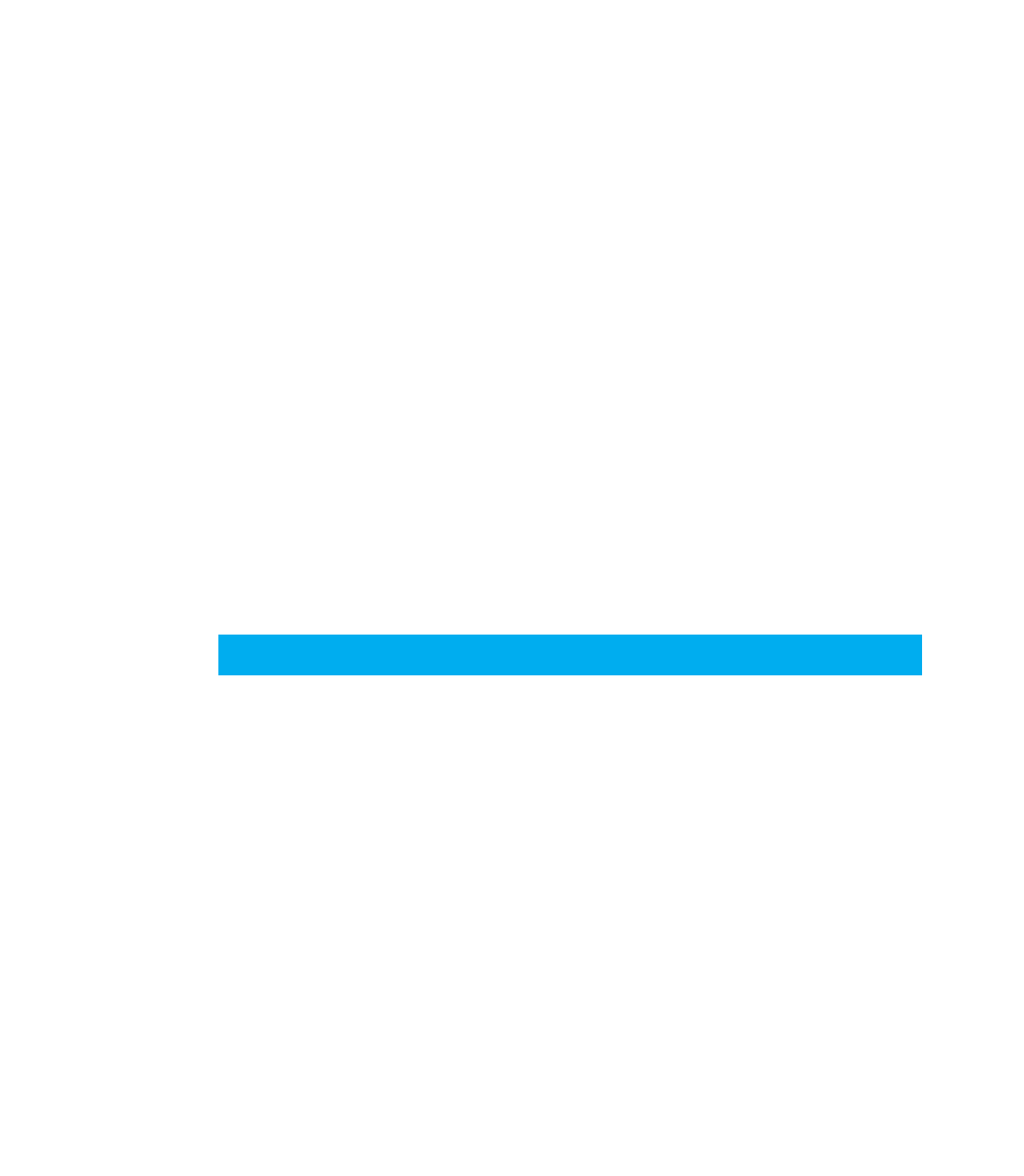
Search WWH ::

Custom Search