Java Reference
In-Depth Information
The
checkCapacity
method was declared to be private, but the preceding method
has been declared to be public, because a client might also want to make use of that
method. For example, if the client recognizes that the capacity needs to be signifi-
cantly increased, then it is useful to be able to call this method to resize it once rather
than resizing several times. The
ArrayList<E>
class has this method available as a
public method.
In Chapter 11 we saw that it is common in the collections framework to use an itera-
tor object to traverse a collection. Recall that an iterator should provide three basic
operations:
•
hasNext()
, which returns true if there are more elements to be examined
•
next()
, which returns the next element from the list and advances the position
of the iterator by one
•
remove()
, which removes the element most recently returned by
next()
We will develop a class called
ArrayIntListIterator
that implements this
functionality for an
ArrayIntList
. The usual convention in Java is to ask the collec-
tion to construct the iterator by calling the method
iterator
:
ArrayIntList list = new ArrayIntList();
// code to fill up list ...
ArrayIntListIterator i = list.iterator();
Once we have obtained an iterator from the list, we can use its three methods to
traverse the list. For example, the following program constructs an
ArrayIntList
and then computes the product of the list:
1
public class
Client2 {
2
public static void
main(String[] args) {
3 // construct and print list
4
int
[] data = {13, 4, 85, 13, 40, -8, 17, -5};
5 ArrayIntList list =
new
ArrayIntList();
6
for
(
int
n : data) {
7 list.add(n);
8 }
9 System.out.println("list = " + list);
10
11 // obtain an iterator to find the product of the list
12 ArrayIntListIterator i = list.iterator();
13
int
product = 1;
14
while
(i.hasNext()) {
15
int
n = i.next();
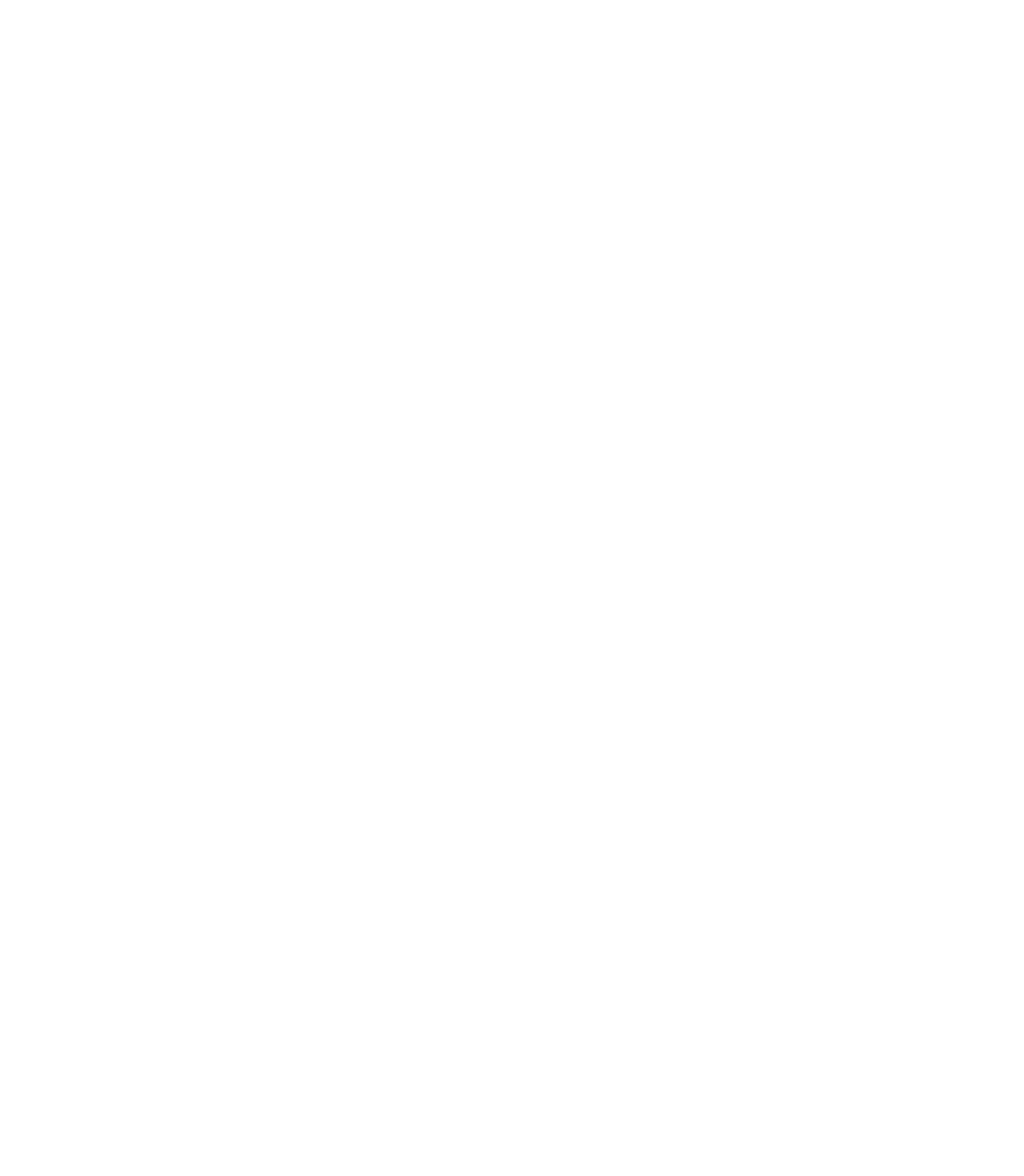
Search WWH ::

Custom Search