Java Reference
In-Depth Information
if (capacity < 0) {
throw new IllegalArgumentException("capacity: " + capacity);
}
elementData = new int[capacity];
size = 0;
}
In order to construct an exception object to be thrown, you have to decide what
type of exception to use. All of the examples we have seen so far have constructed
exceptions of type
IllegalArgumentException
. This is a kind of generic exception
that is used to indicate that some value passed as an argument was not legal. But
there are other exception types that you can use. The convention in Java is to pick the
most specific exception that you can. Table 15.1 lists of some of the most common
exception types.
NullPointerException
and
ArrayIndexOutOfBoundsException
are thrown
by Java automatically, so you don't generally write code to specify those exceptions.
Java programmers often use the other four exceptions, however.
Both of the
add
methods in your
ArrayIntList
class have a precondition that the
size of the list must be strictly less than the capacity. Otherwise you will attempt to
store a value in an array index that doesn't exist. This is an appropriate situation for
an
IllegalStateException
. It is not appropriate to add values to the list once it
has reached its capacity. In this case, you want to let the client know that the problem
doesn't come from the values passed as arguments, but rather, that the
add
method
was called at an inappropriate time.
You could add code to each of the
add
methods to check for this exception, but to
avoid redundancy, it is better to introduce a private method that each of the methods
calls. You'll be introducing some other methods that add more than one value at a
time, so it will be useful to write the private method in a fairly flexible way. You can
write a method that takes a required capacity as a parameter and that checks to make
Table 15.1
Common Exception Types
Exception Type
Description
A
null
value has been used in a case that requires
an object.
NullPointerException
A value passed as an index to an array is illegal.
ArrayIndexOutOfBoundsException
A value passed as an index to some nonarray
structure is illegal.
IndexOutOfBoundsException
A method has been called at an illegal or
inappropriate time.
IllegalStateException
A value passed as an argument to a method is illegal.
IllegalArgumentException
A call was made on an iterator's
next
method when
there are no values left to iterate over.
NoSuchElementException
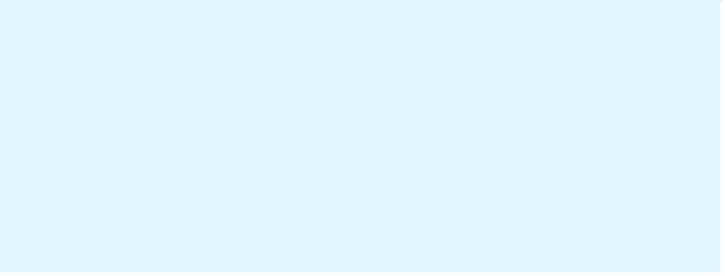



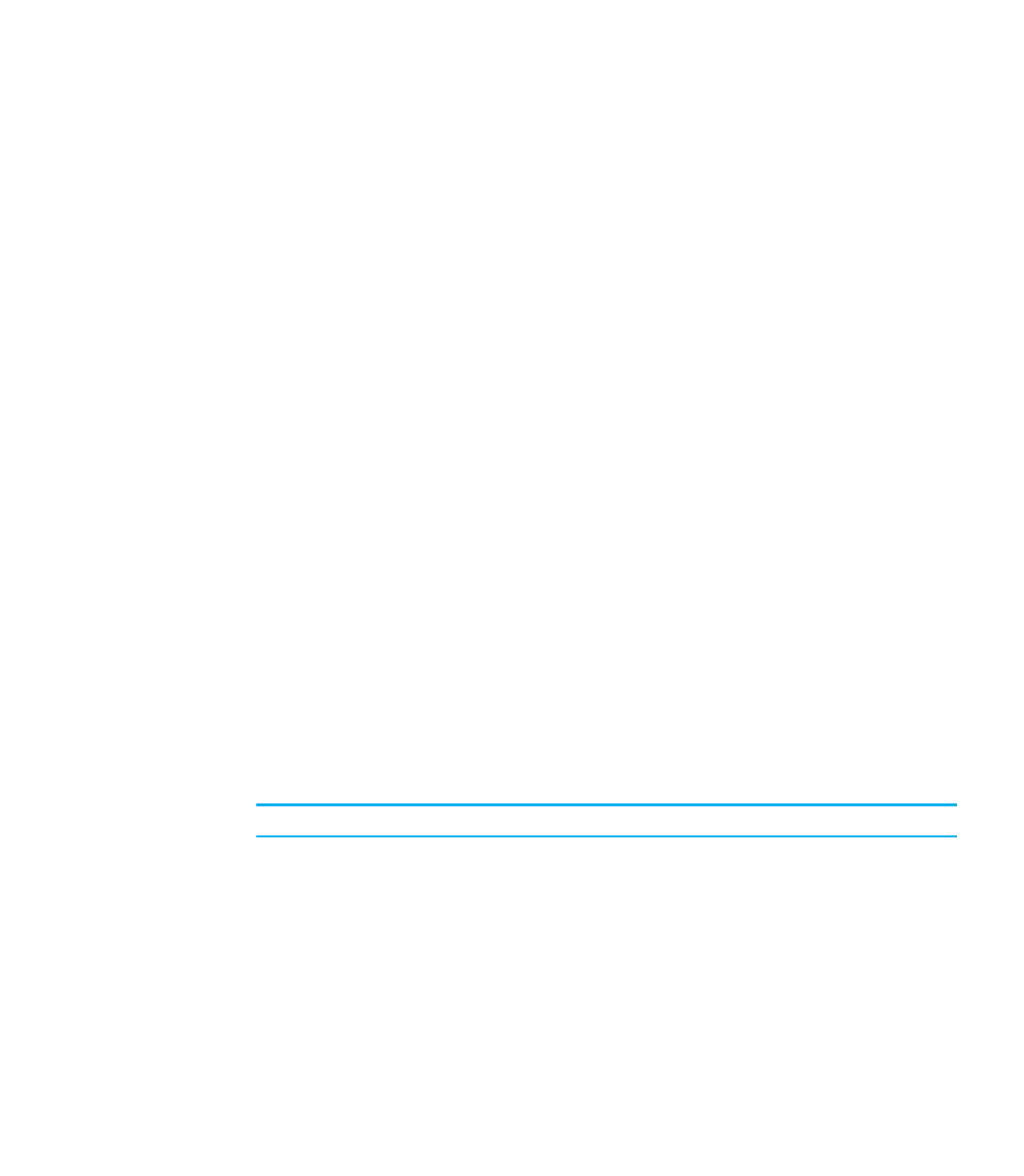
Search WWH ::

Custom Search