Java Reference
In-Depth Information
Here is a complete version of the
ArrayIntList
class incorporating all of the
pieces discussed in this section:
1 // Class ArrayIntList can be used to store a list of integers.
2
3
public class
ArrayIntList {
4
private int
[] elementData; // list of integers
5
private int
size; // number of elements in the list
6
7
public static final int
DEFAULT_CAPACITY = 100;
8
9 // post: constructs an empty list of default capacity
10
public
ArrayIntList() {
11
this
(DEFAULT_CAPACITY);
12 }
13
14 // pre : capacity >= 0
15 // post: constructs an empty list with the given capacity
16
public ArrayIntList
(
int
capacity) {
17 elementData =
new int
[capacity];
18 size = 0;
19 }
20
21 // post: returns the current number of elements in the list
22
public int
size() {
23
return
size;
24 }
25
26 // pre : 0 <= index < size()
27 // post: returns the integer at the given index in the list
28
public int
get(
int
index) {
29
return
elementData[index];
30 }
31
32 // post: returns comma-separated, bracketed version of list
33
public
String toString() {
34
if
(size == 0) {
35
return
"[]";
36 }
else
{
37 String result = "[" + elementData[0];
38
for
(
int
i = 1; i < size; i++) {
39 result + = ", " + elementData[i];
40 }
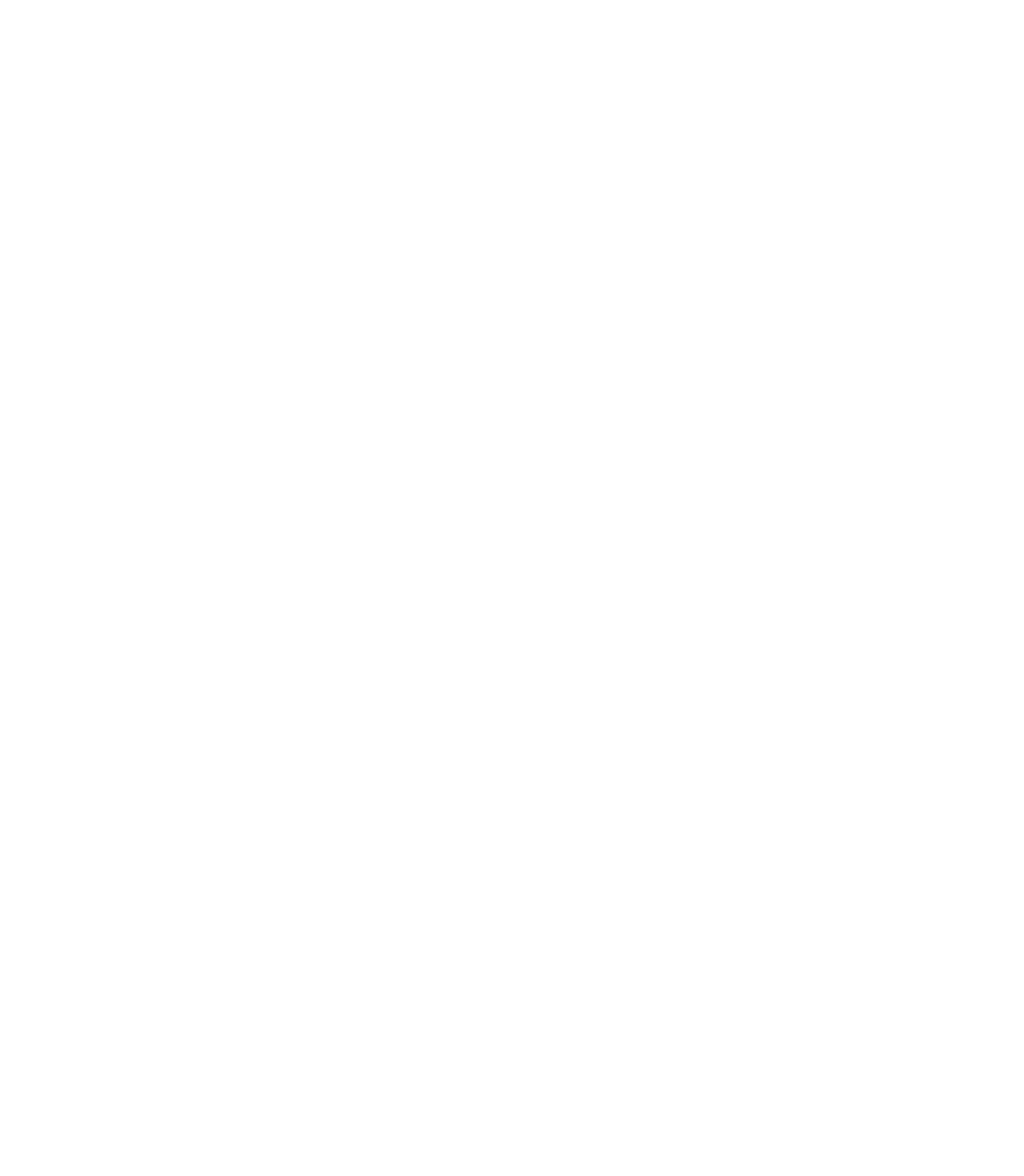
Search WWH ::

Custom Search