Java Reference
In-Depth Information
return "[]";
} else {
String result = "[" + elementData[0];
for (int i = 1; i < size; i++) {
result += ", " + elementData[i];
}
result += "]";
return result;
}
}
Here is the complete class:
1
public class
ArrayIntList {
2
private int
[] elementData;
3
private int
size;
4
5
public
ArrayIntList() {
6 elementData =
new
int[100];
7 size = 0;
8 }
9
10
public void
add(
int
value) {
11 elementData[size] = value;
12 size++;
13 }
14
15
public
String toString() {
16
if
(size == 0) {
17
return
"[]";
18 }
else
{
19 String result = "[" + elementData[0];
20
for
(
int
i = 1; i < size; i++) {
21 result += ", " + elementData[i];
22 }
23 result + = "]";
24
return
result;
25 }
26 }
27 }
This version of the class correctly executes the sample client program, producing
the correct output.
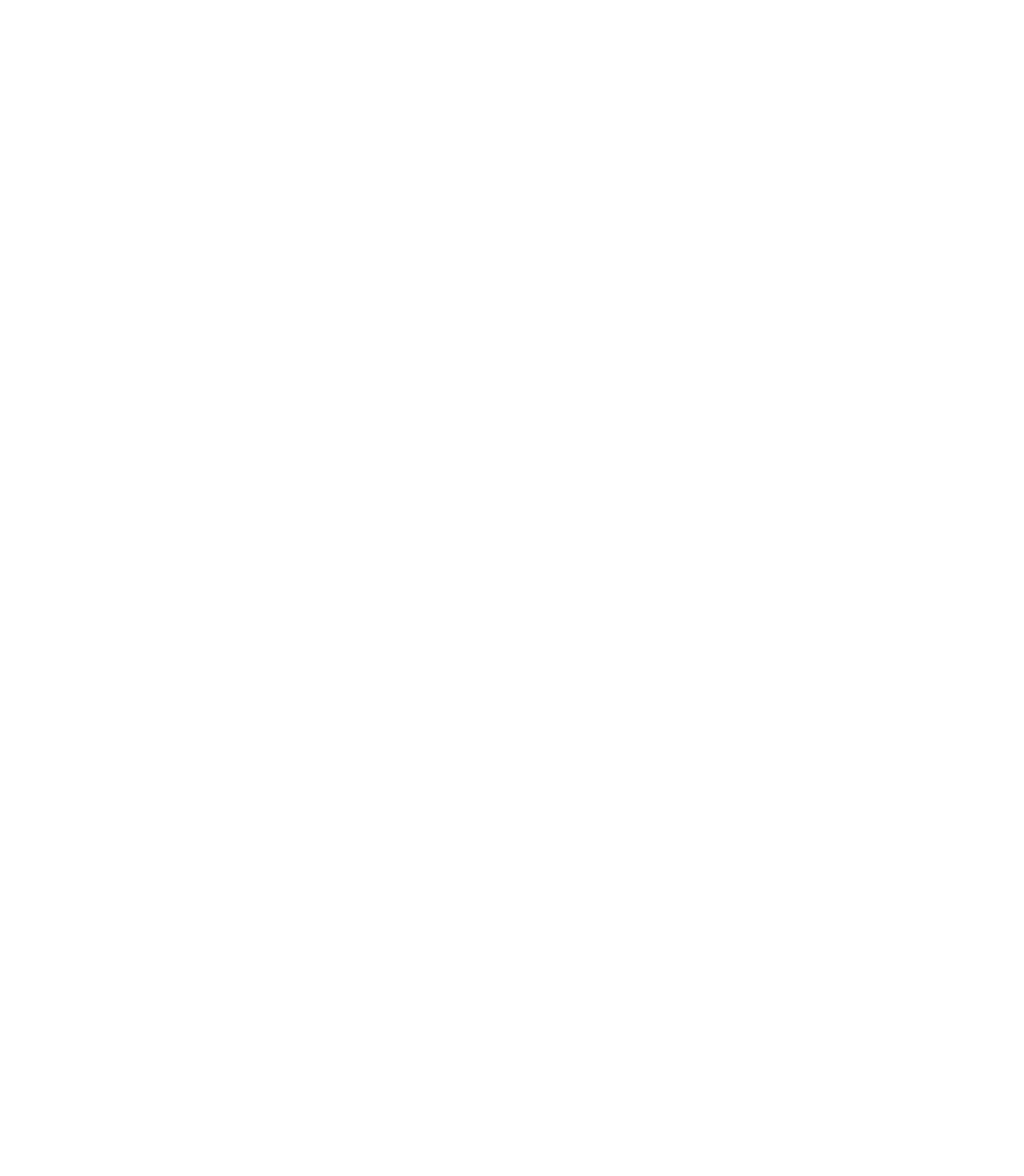
Search WWH ::

Custom Search