Java Reference
In-Depth Information
This turns out to be a fairly simple method to implement. Suppose, for example,
that the list stores the values
[3, 6, 9, 12, 15]
:
occupied
vacant
[0]
[1]
[2]
[3]
[4]
[5]
[6] [7] [...][99]
elementData
3
6
9
12
15
0
0
0
...
0
size
5
As indicated in the preceding figure, the current size of the list is 5. If you wanted
to add the value
18
to the end of this list, you'd store it in the first vacant array ele-
ment, which has index 5. In other words, when the list has a size of 5, you append the
next value into index 5. And once you've added that value into index 5, then the list
will have a size of 6 and the next vacant element will be at index 6.
Generally speaking, the size of the list will always match the index of the first
vacant cell. This seems a bit odd, but it is a result of zero-based indexing. Because
the first value is stored in index 0, the last value of a sequence of length
n
will be
stored in index
n
1.
Therefore you should begin the
add
method by appending the given value at index
size
:
public void add(int value) {
elementData[size] = value;
...
}
Storing the value into the array isn't enough. You have to increment the
size
to
keep track of the fact that one more cell is now occupied:
public void add(int value) {
elementData[size] = value;
size++;
}
If your program did not increment
size
, it would keep adding values in the same
index of the array. This would be like our hypothetical hotel checking every customer
into the same hotel room because the hotel didn't keep track of which rooms are
occupied.
It seems likely that this method will work, but so far the program doesn't have a
good way of verifying it. You need some kind of method for displaying the contents
of the list.





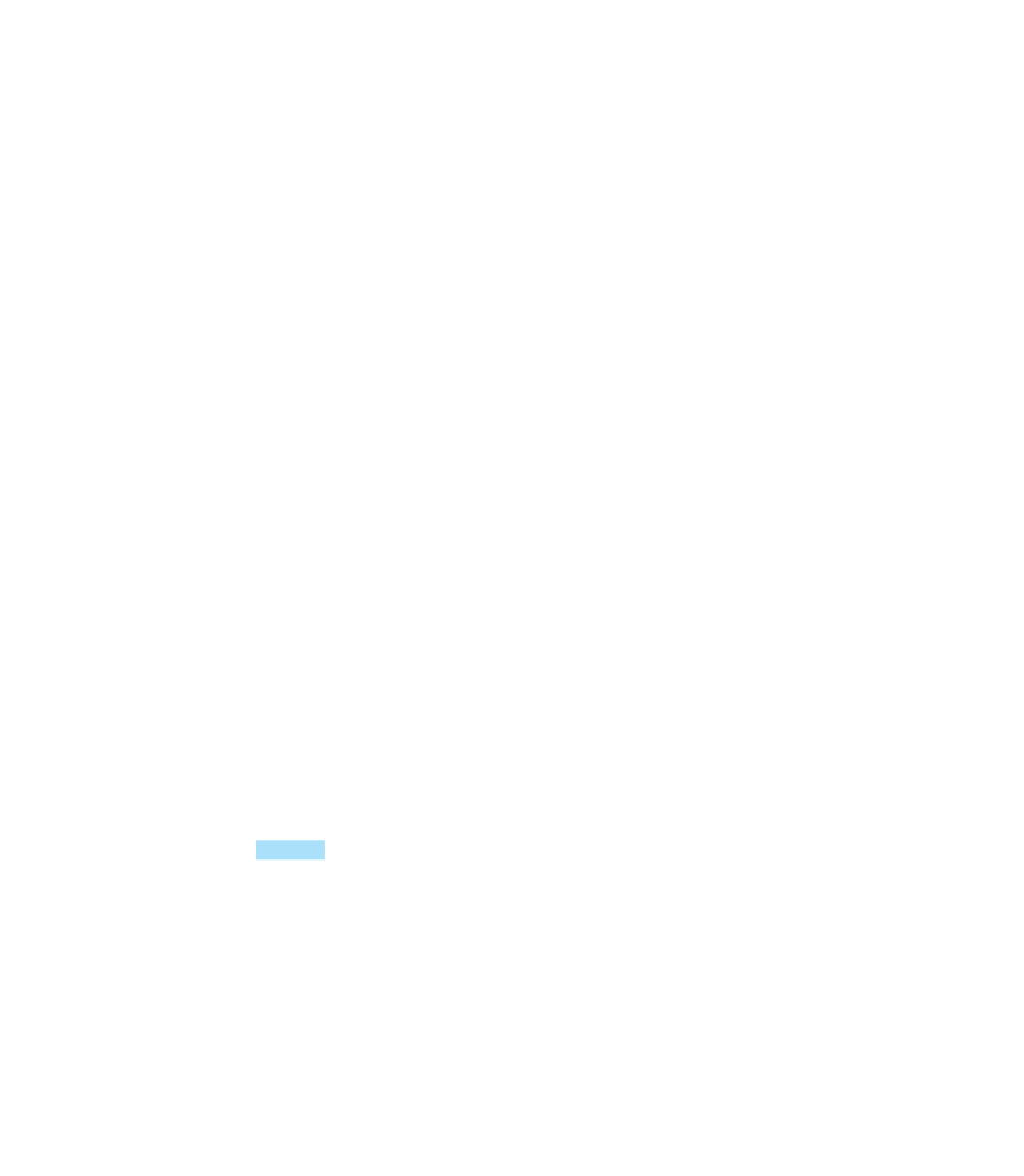
Search WWH ::

Custom Search