Java Reference
In-Depth Information
To avoid the annoyance of writing empty bodies for the
MouseInputListener
methods that you don't want to use, you can use a class named
MouseInputAdapter
that implements default empty versions of all the methods from the
MouseInputListener
interface. You can extend
MouseInputAdapter
and override
only the methods that correspond to the mouse event types you want to handle. We'll
make all the mouse listeners we write subclasses of
MouseInputAdapter
so that we
don't have to write any unwanted methods.
For example, let's write a mouse listener class that responds only when the mouse
cursor moves over a component. To do this, we'll extend the
MouseInputAdapter
class and override only the
mouseEntered
method.
1 // Responds to a mouse event by showing a message dialog.
2
3
import
java.awt.event.*;
4
import
javax.swing.*;
5
import
javax.swing.event.*;
6
7
public class
MovementListener
extends
MouseInputAdapter {
8
public void
mouseEntered(MouseEvent event) {
9 JOptionPane.showMessageDialog(
null
, "Mouse entered!");
10 }
11 }
Unfortunately, attaching this new listener to a component isn't so straightforward.
The designers of the GUI component classes decided to separate the various types of
mouse actions into two categories, mouse button clicks and mouse movements, and
created two separate interfaces (
MouseListener
and
MouseMotionListener
) to
represent these types of actions. Later, the
MouseInputListener
and
MouseInputAdapter
methods were added to merge the two listeners, but GUI com-
ponents still need mouse listeners to be attached in two separate ways for them
to work.
If we wish to hear about mouse enter, exit, press, release, and click events, we
must call the
addMouseListener
method on the component. If we wish to hear
about mouse move and drag events, we must call the
addMouseMotionListener
method on the component. If we want to hear about all the events, we can add the
mouse input adapter in both ways. (This is what we'll do in the examples in this
section.)
Table 14.8
Useful Methods of Components
public void addMouseListener(MouseListener listener)
Attaches a listener to hear mouse enter, exit, press, release, and click events.
public void addMouseMotionListener(MouseMotionListener listener)
Attaches a listener to hear mouse move and drag events.
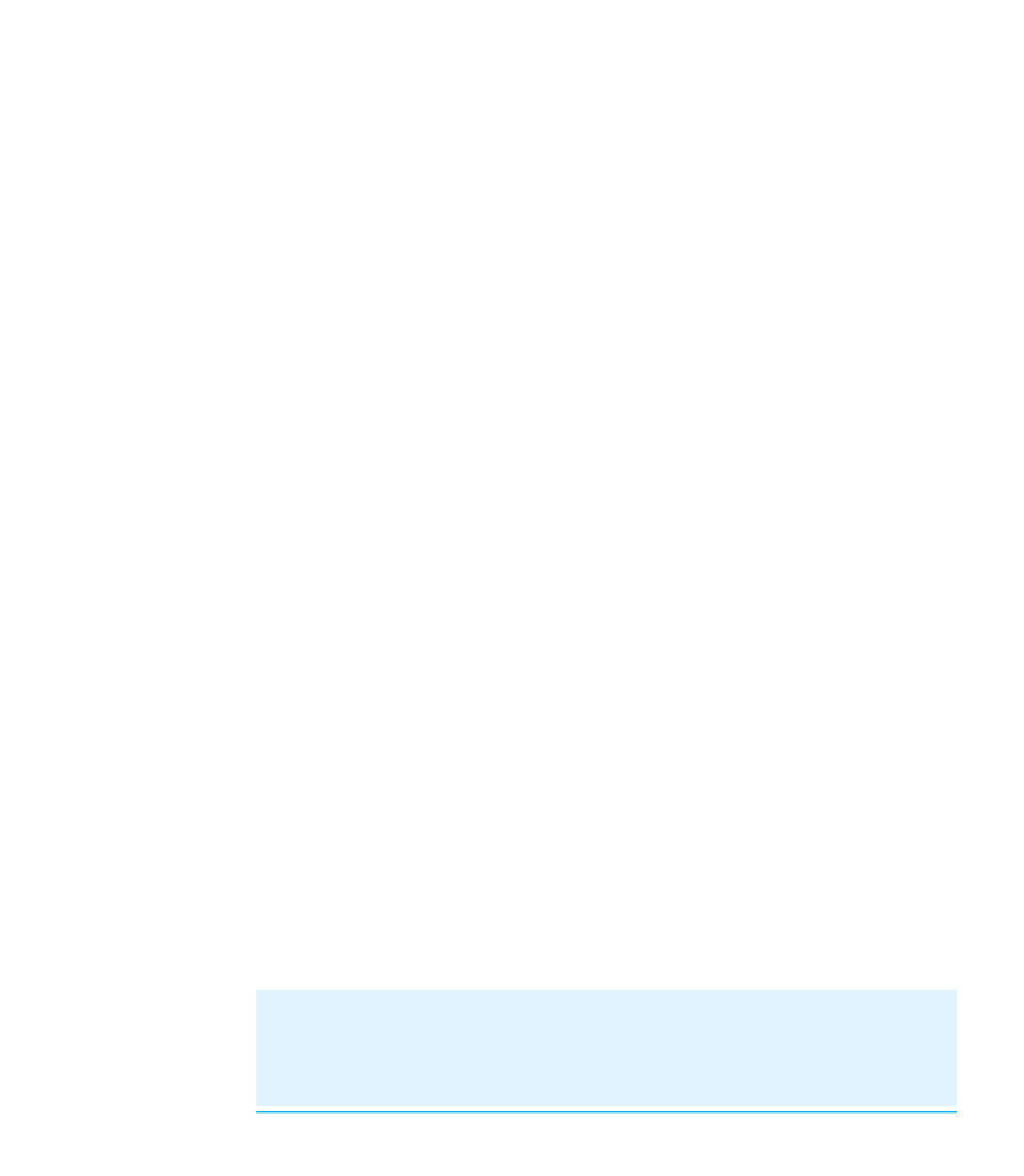
Search WWH ::

Custom Search