Java Reference
In-Depth Information
5
import
javax.swing.*;
6
7
public class
BmiGui1 {
8
public static void
main(String[] args) {
9 // set up components
10 JTextField heightField =
new
JTextField(5);
11 JTextField weightField =
new
JTextField(5);
12 JLabel bmiLabel =
new
JLabel(
13 "Type your height and weight");
14 JButton computeButton =
new
JButton("Compute");
15
16 // layout
17 JPanel north =
new
JPanel(
new
GridLayout(2, 2));
18 north.add(
new
JLabel("Height: "));
19 north.add(heightField);
20 north.add(
new
JLabel("Weight: "));
21 north.add(weightField);
22
23 // overall frame
24 JFrame frame =
new
JFrame("BMI");
25 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
26 frame.setLayout(
new
BorderLayout());
27 frame.add(north, BorderLayout.NORTH);
28 frame.add(bmiLabel, BorderLayout.CENTER);
29 frame.add(computeButton, BorderLayout.SOUTH);
30 frame.pack();
31 frame.setVisible(
true
);
32 }
33 }
The program currently does nothing when the user presses the Compute button.
Let's think about how to make our BMI GUI respond to clicks on the Compute but-
ton. Clicking a button causes an action event, so we might try putting an
ActionListener
on the button. But the code for the listener's
actionPerformed
method would need to read the text from the height and weight text fields and use
that information to compute the BMI and display it as the text of the central label.
This is a problem because the GUIs we've written so far were not built to allow so
much interaction between components.
To enable listeners to access each of the GUI components, we need to make our GUI
itself into an object. We'll declare the onscreen components as fields inside that object
and initialize them in the GUI's constructor. In our
BmiGui1
example, we can convert
much of the code that is currently in the
main
method into the constructor for the GUI.
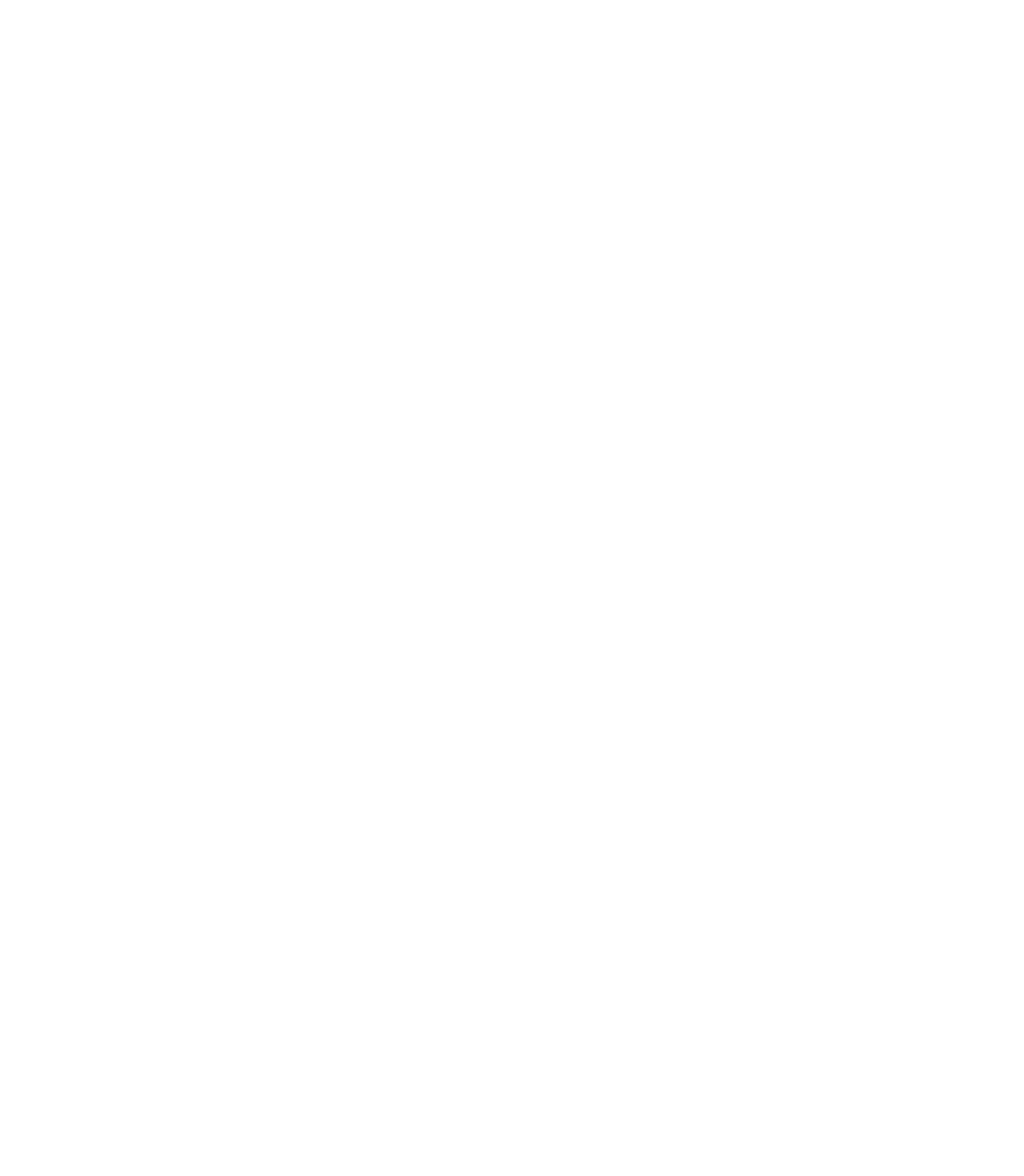
Search WWH ::

Custom Search