Java Reference
In-Depth Information
6
public class
BorderLayoutExample {
7
public static void
main(String[] args) {
8 JFrame frame =
new
JFrame();
9 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
10 frame.setSize(
new
Dimension(210, 200));
11 frame.setTitle("Run for the border");
12
13 frame.setLayout(
new
BorderLayout());
14 frame.add(
new
JButton("north"), BorderLayout.NORTH);
15 frame.add(
new
JButton("south"), BorderLayout.SOUTH);
16 frame.add(
new
JButton("west"), BorderLayout.WEST);
17 frame.add(
new
JButton("east"), BorderLayout.EAST);
18 frame.add(
new
JButton("center"), BorderLayout.CENTER);
19
20 frame.setVisible(
true
);
21 }
22 }
Here's the graphical output of the program, both before and after resizing:
Notice the stretching behavior: The north and south buttons stretch horizontally
but not vertically, the west and east buttons stretch vertically but not horizontally,
and the center button gets all the remaining space. You don't have to place compo-
nents in all five regions; if you leave a region empty, the center consumes its
space.
Here is a summary of the stretching and resizing behaviors of the layout managers:
•
FlowLayout
: Does not stretch components. Wraps to next line if necessary.
•
GridLayout
: Stretches all components in both dimensions to make them equal
in size at all times.
•
BorderLayout
: Stretches north and south regions horizontally but not vertically,
stretches west and east regions vertically but not horizontally, and stretches center
region in both dimensions to fill all remaining space not claimed by the other four
regions.
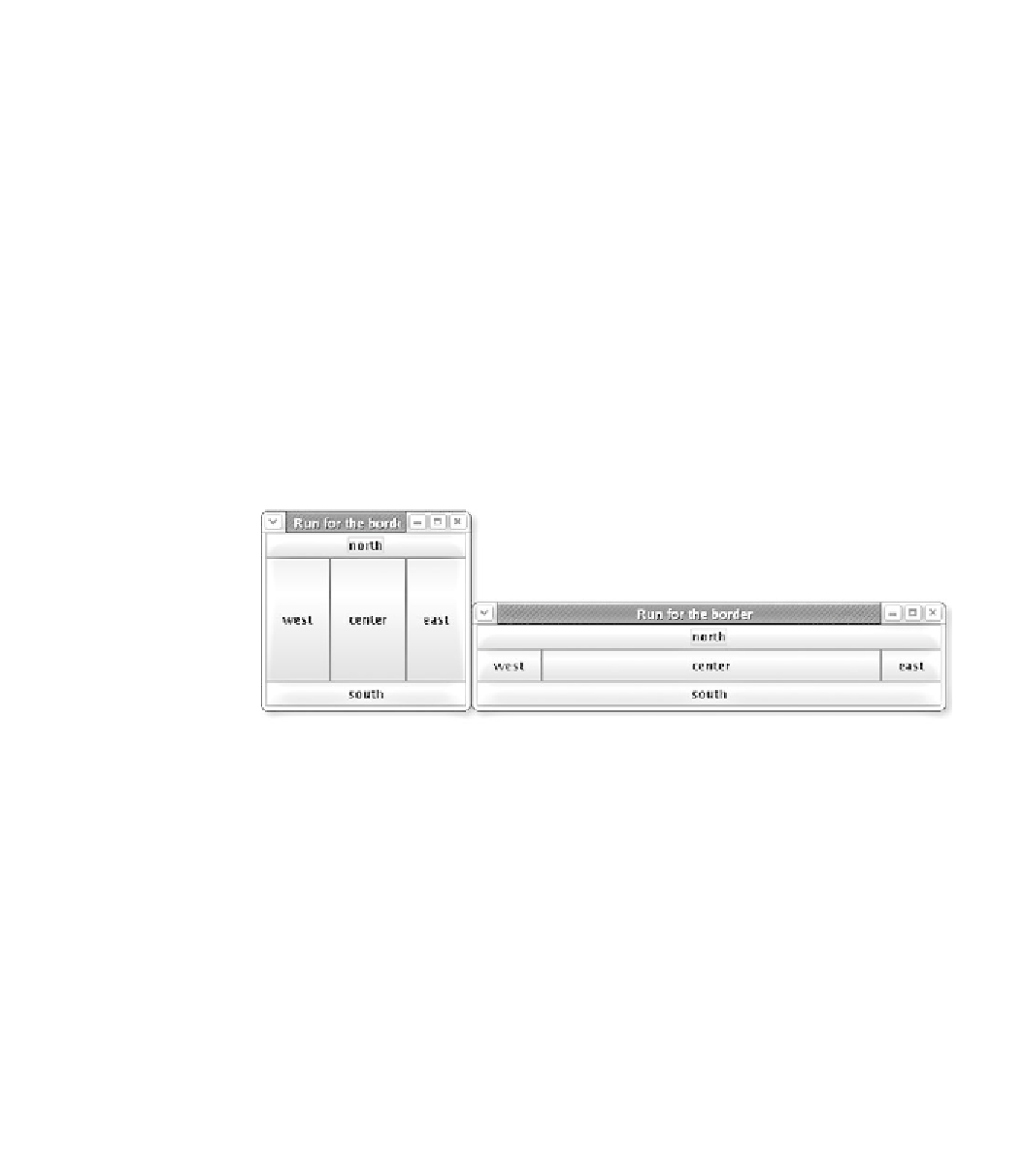
Search WWH ::

Custom Search