Java Reference
In-Depth Information
Arrays.sort(strings);
System.out.println(Arrays.toString(strings));
This code produces the following output, which may not be what you expected:
[Charlie, DELTA, Foxtrot, alpha, bravo, echo, golf, hotel]
Notice that the elements are in case-sensitive alphabetical ordering, with all the
uppercase strings placed before the lowercase ones.
Recall from Chapter 10 that many types (including
String
) have natural order-
ings that are defined by comparison functions, implemented as the
compareTo
method from the
Comparable
interface. The
compareTo
method of
String
objects
uses a case-sensitive ordering, but in this case we would prefer a case-insensitive
comparison. We might also want an option to sort in other orders, such as by length
or in reverse alphabetical order.
In situations such as these, you can define your own external comparison functions
with an object called a
comparator.
Comparators perform comparisons between pairs
of objects. While a class can have only one natural ordering, arbitrarily many compara-
tors can be written to describe other ways to order the class's objects. A comparator can
also supply an external ordering on a class that does not implement a natural ordering
of its own. The
Arrays.sort
,
Collections.sort
, and
Arrays.binarySearch
methods all have variations that accept a comparator as an additional parameter and use
the comparator to guide the searching or sorting process.
Comparators are implemented as classes that implement the interface
Comparator
in the
java.util
package. This interface has a method called
compare
that accepts
a pair of objects as parameters and compares them. Like
compareTo
, the
compare
method returns a negative number, zero, or a positive number to indicate a less-than,
equal-to, or greater-than relationship, respectively:
public interface Comparator<T> {
public int compare(T o1, T o2);
}
Implementing
Comparator
is like implementing the
Comparable
interface,
except that instead of placing the code inside the class to be compared, you write a
separate comparator class. The
compare
method is similar to the
compareTo
method
from the
Comparable
interface, except that it accepts both of the objects to compare
as parameters. (The
compareTo
method accepts only one object as a parameter
because the other object compared is the implicit parameter.)
Like
Comparable
,
Comparator
is actually a generic interface
Comparator<T>
that must be told the type of objects you'll be comparing. For example, if you wish to
write a comparison function for
String
s, you must write a class that implements
Comparator<String>
.
Before we implement a comparator of our own, let's use one that is part of Java's
class libraries. If you want to sort
String
s in case-insensitive alphabetical order, you
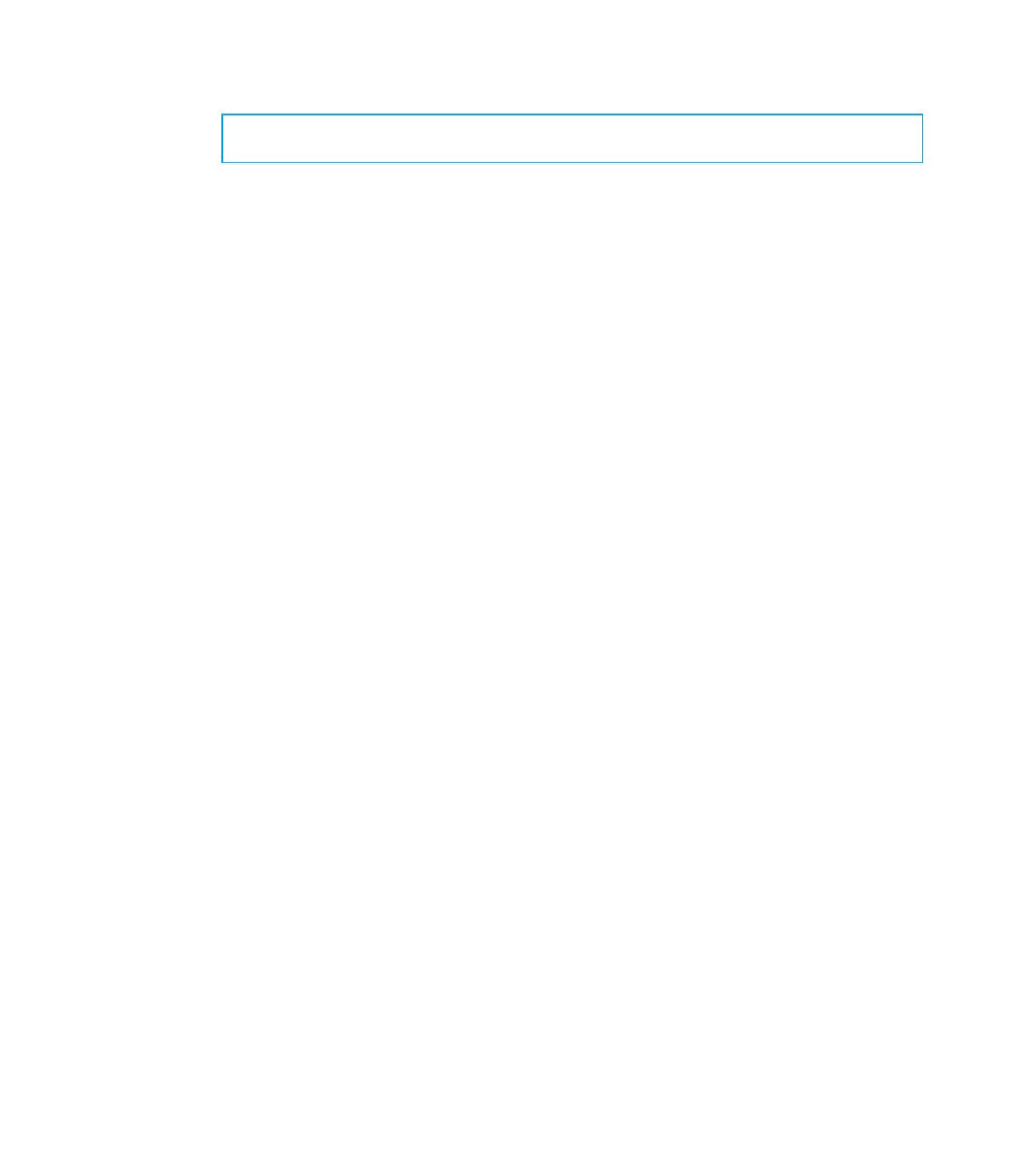
Search WWH ::

Custom Search