Java Reference
In-Depth Information
triangles. The method will also need to know the level to use and the three vertices of
the triangle, which we can pass as
Point
objects. Our method will look like this:
public static void drawFigure(int level, Graphics g,
Point p1, Point p2, Point p3) {
...
}
Our base case will be to draw the basic triangle for level 1. The
Graphics
class has
methods for filling rectangles and ovals, but not for filling triangles. Fortunately, there is a
Polygon
class in the
java.awt
package. We can construct a
Polygon
and then add a
series of points to it. Once we've specified the three vertices, we can use the
fillPolygon
method of the
Graphics
class to fill the polygon. Our base case will look like this:
public static void drawFigure(int level, Graphics g,
Point p1, Point p2, Point p3) {
if (level == 1) {
// base case: simple triangle
Polygon p = new Polygon();
p.addPoint(p1.x, p1.y);
p.addPoint(p2.x, p2.y);
p.addPoint(p3.x, p3.y);
g.fillPolygon(p);
} else {
// recursive case, split into 3 triangles
...
}
}
Most of the work happens in the recursive case. We have to split the triangle into
three smaller triangles. We'll label the vertices of the overall triangle (see Figure 12.5).
p2
p1
p3
Figure 12.5
Triangle before splitting
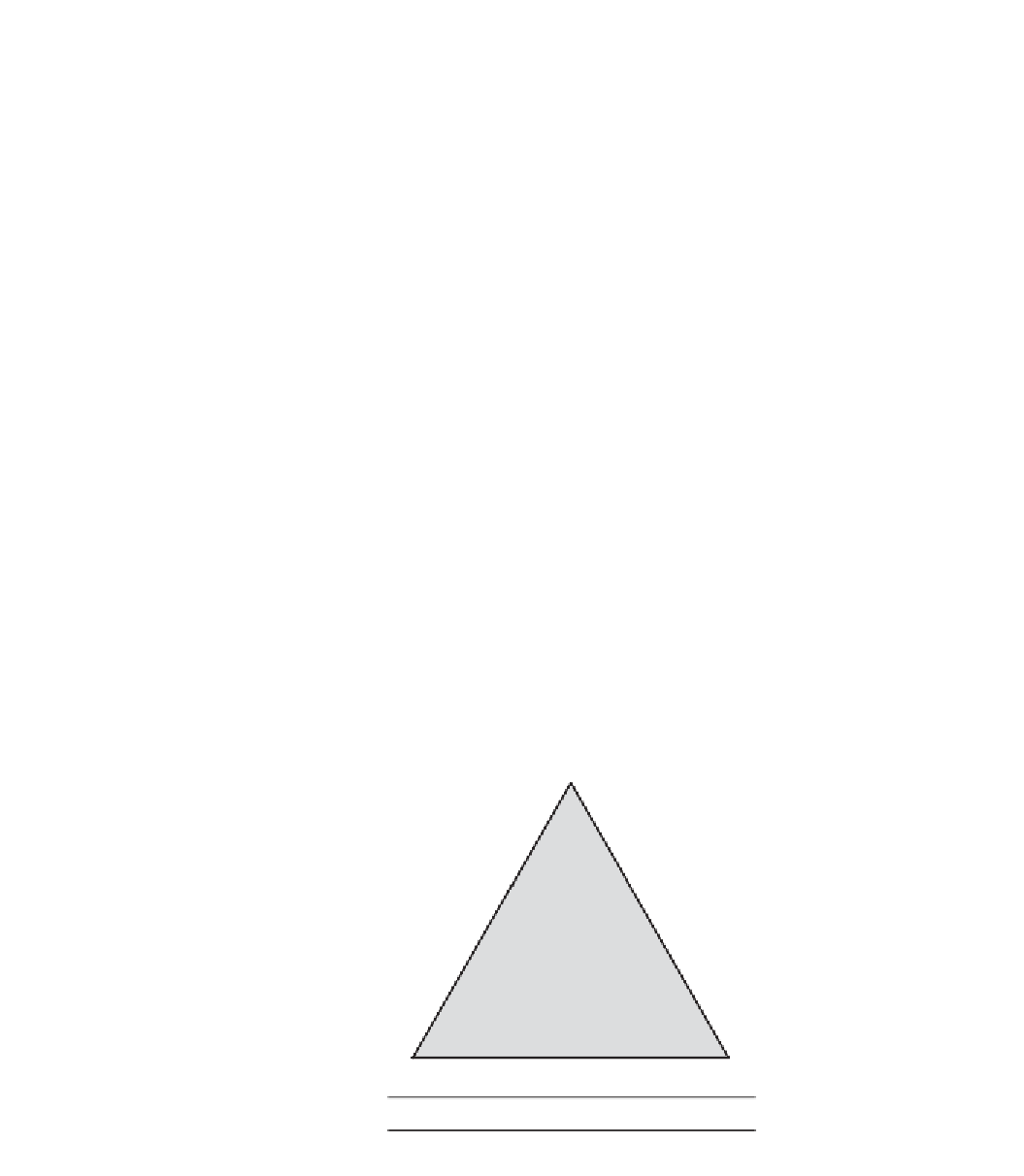
Search WWH ::

Custom Search