Java Reference
In-Depth Information
Using those two parameters, you'll find that the code is fairly simple to write
using the approach described before:
// computes the sum of the list starting at the given index
public int sum(int[] list, int index) {
if (index == list.length) {
return 0;
} else {
return list[index] + sum(list, index + 1);
}
}
Of course, there's the issue of that extra parameter. We were asked to write a
method that is passed just the array. We could complain that we were asked to solve
the wrong problem, but that's a bad attitude to have. We should allow a client to call
methods using parameters that are convenient. We need the second parameter to write
our recursive solution, but we shouldn't burden the client with the need to understand
that detail.
This is a common situation. We often find ourselves wanting to write a recursive
method that has extra parameters relative to the public method we are being asked to
implement. The solution is to introduce a private method that has the parameter pass-
ing we want. So the client gets the convenient public method with the kind of param-
eter passing that the client wants, and we get our convenient private method with the
kind of parameter passing that we want. We call the private method a helper method
because it helps us to write the public method we've been asked to implement.
Here is the code that includes the private method:
// returns the sum of the numbers in the given array
public int sum(int[] list) {
return sum(list, 0);
}
// computes the sum of the list starting at the given index
private int sum(int[] list, int index) {
if (index == list.length) {
return 0;
} else {
return list[index] + sum(list, index + 1);
}
}
The public method simply calls the private method, passing it an appropriate
value for the second parameter. Both methods can be called
sum
because they have
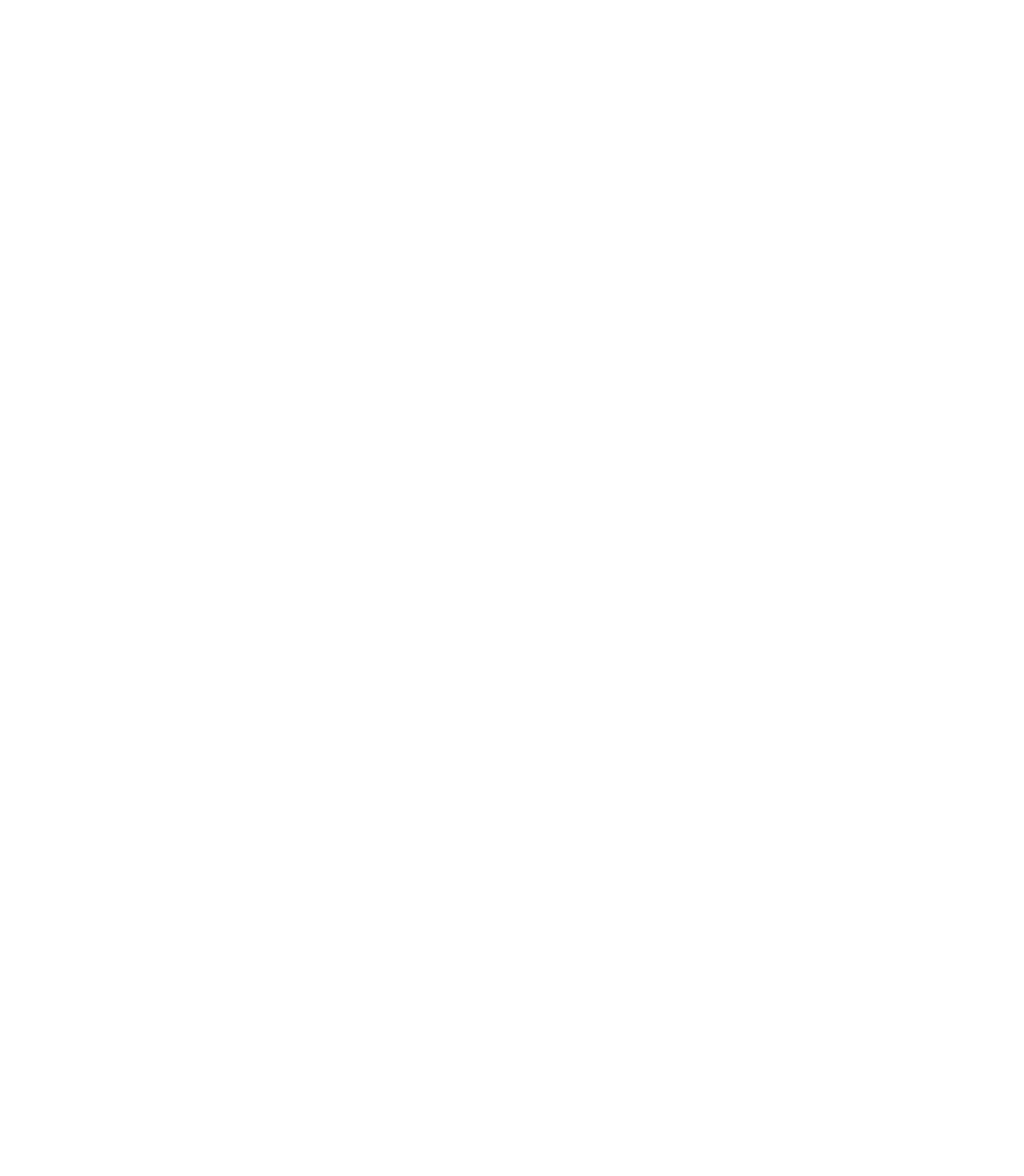
Search WWH ::

Custom Search