Java Reference
In-Depth Information
Recursion is particularly useful when you're working with data that is itself recur-
sive. For example, consider how files are stored on a computer. Each file is kept in a
folder or directory. But directories can contain more than just files: Directories can
contain other directories, those inner directories can contain directories, and even
those directories can contain directories. Directories can be nested to an arbitrary
depth. This storage system is an example of recursive data.
In Chapter 6, you learned to use
File
objects to keep track of files stored on your
computer. For example, if you have a file called
data.txt
, you can construct a
File
object that can be used to get information about that file:
File f = new File("data.txt");
Several useful methods for the
File
class were introduced in Chapter 6. For
example, the
exists
method indicates whether or not a certain file exists, and the
isDirectory
method indicates whether or not the name supplied corresponds to a
file or a directory.
Let's write a program that will prompt the user for the name of a file or directory
and recursively explore all the files that can be reached from that starting point. If the
user provides the name of a file, the program should simply print the name. But if the
user gives the name of a directory, the program should print the directory name and
list all the directories and files inside that directory.
We can write a fairly simple
main
method that prompts for a file or directory name
and checks to make sure it exists. If it does not, the program tells the user that there is
no such file or directory. If it does exist, the program calls a method to print the infor-
mation for that
File
object:
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
System.out.print("directory or file name?");
String name = console.nextLine();
File f = new File(name);
if (!f.exists()) {
System.out.println("No such file/directory");
} else {
print(f);
}
}
Our job will be to write the
print
method. The method should begin by printing
the name of the file or directory:
public static void print(File f) {
System.out.println(f.getName());
...
}
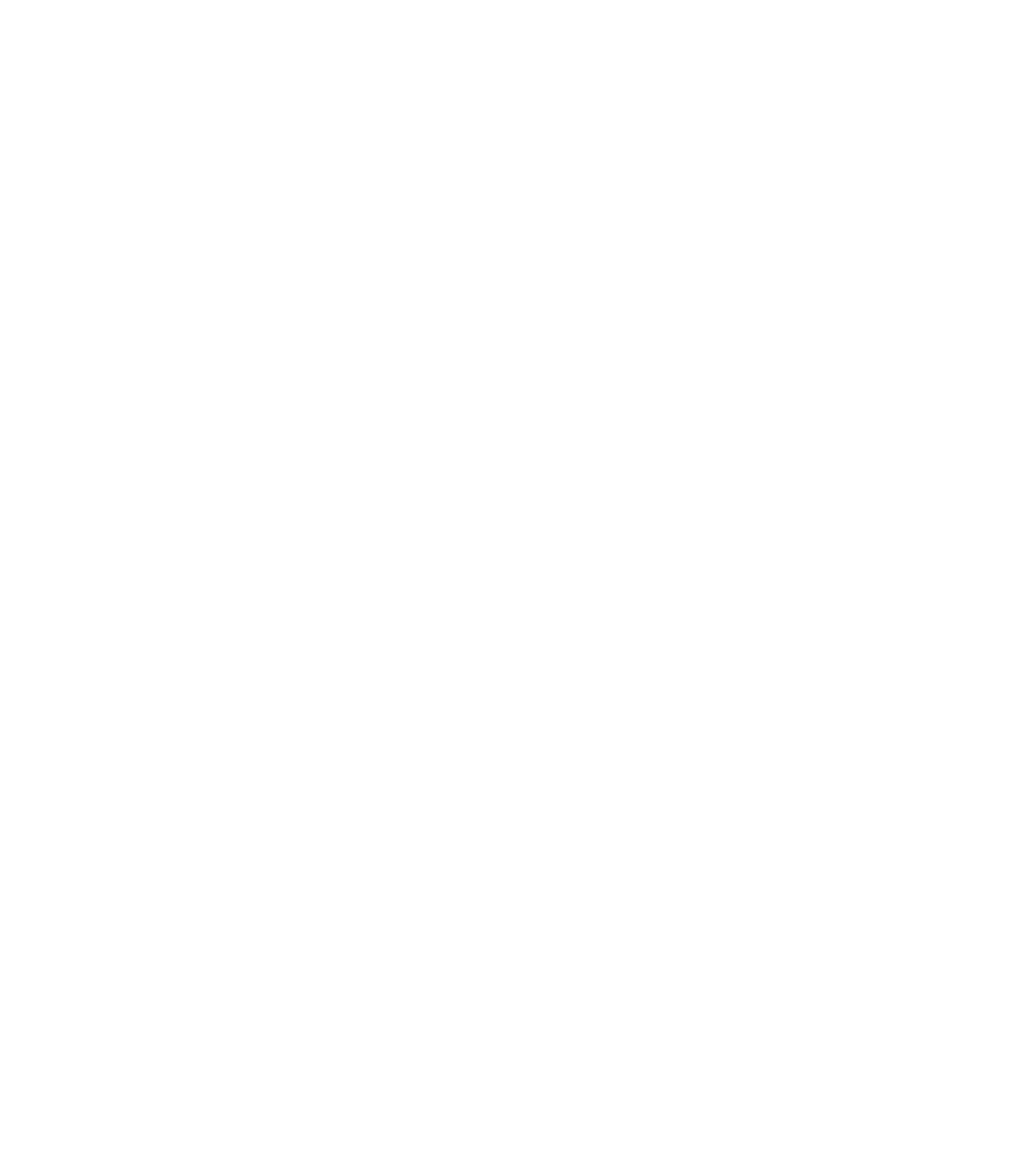
Search WWH ::

Custom Search