Java Reference
In-Depth Information
This version never finishes executing, a phenomenon called
infinite recursion.
For
example, if you ask the method to write a line of 10 stars, it tries to accomplish that
by asking the method to write a line of 10 stars, which asks the method to write a line
of 10 stars, which asks the method to write a line of 10 stars, and so on. This solution
is the recursive equivalent of an infinite loop.
Every recursive solution that you write will have two key ingredients: a
base case
and a
recursive case.
Base Case
A case within a recursive solution that is so simple that it can be solved
directly without a recursive call.
Recursive Case
A case within a recursive solution that involves reducing the overall problem
to a simpler problem of the same kind that can be solved by a recursive call.
Here is the
writeStars
method again, with comments indicating the base case
and recursive case:
public static void writeStars(int n) {
if (n == 0) {
// base case
System.out.println();
} else {
// recursive case
System.out.print("*");
writeStars(n - 1);
}
}
The base case is the task of writing a line of zero stars. This task is so simple that
it can be done immediately. The recursive case is the task of writing lines with one or
more stars. To solve the recursive case, you begin by writing a single star, which
reduces the remaining task to that of writing a line of
(n - 1)
stars. This is the task
that the
writeStars
method is designed to solve and it is simpler than the original
task, so you can solve it by making a recursive call.
As an analogy, suppose you're at the top of a ladder with
n
rungs on it. If you have
a way to get from one rung to the one below and if you can recognize when you've
reached the ground, you can handle a ladder of any height. Stepping from one rung to
the one below is like the recursive case in which you perform some small amount of
work that reduces the problem to a simpler one of the same form (get down from
rung
(n - 1)
versus get down from rung
n
). Recognizing when you reach the
ground is like the base case that can be solved directly (stepping off the ladder).

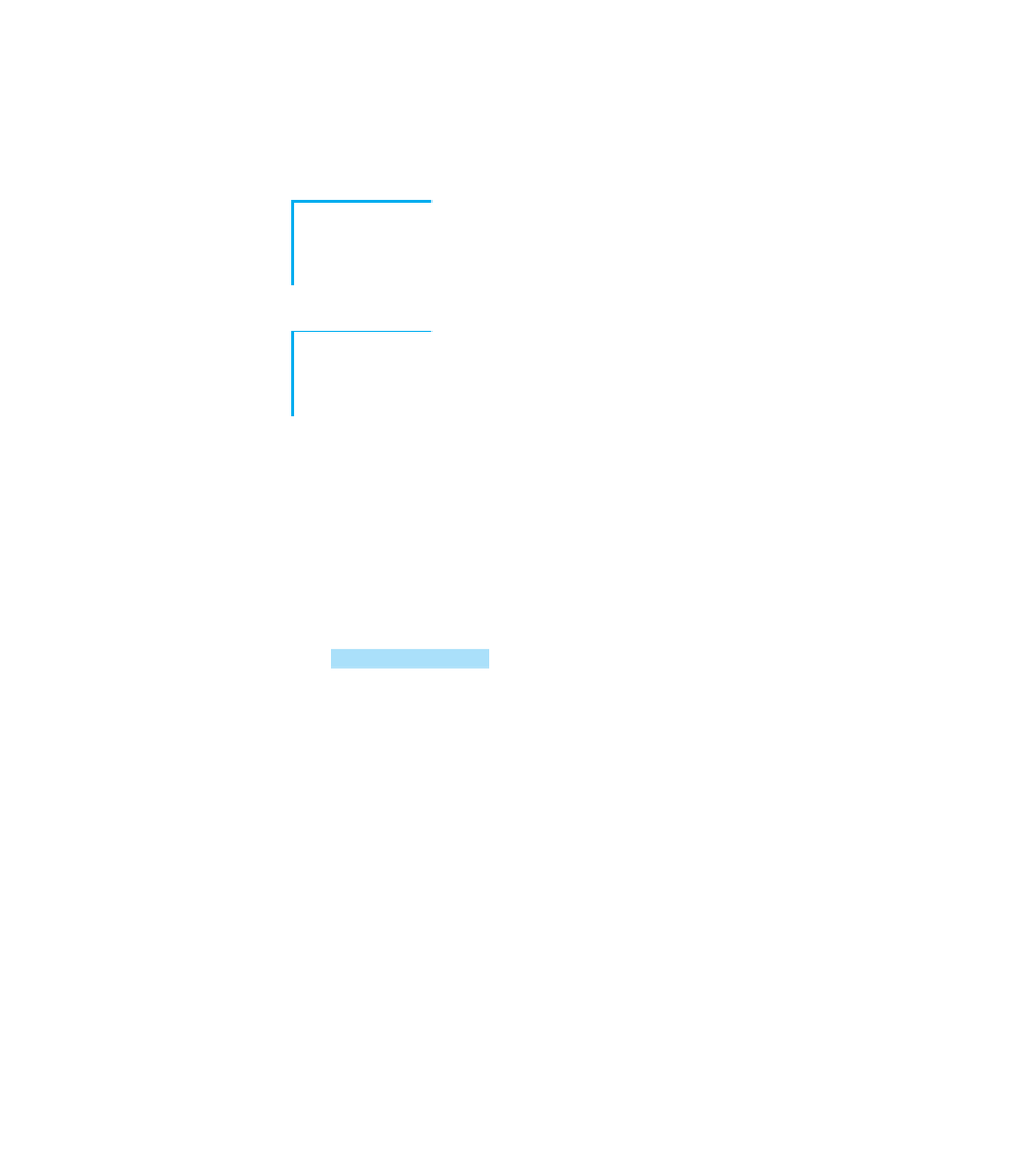
Search WWH ::

Custom Search