Java Reference
In-Depth Information
These diagrams included just 4 individuals for the sake of brevity, but this process
would still work even if there were 30 or even 300 people in the line.
One of the key aspects to notice here is that recursion involves many cooperating
entities, each of which solves a little bit of the problem. Instead of one person doing
all of the counting, each individual asks one question as we go towards the front of
the line and answers one question as we come back down the line.
In programming, the iterative solution of having one person do all the counting is
like having a loop that repeats some action. The recursive solution of having many
people each do a little bit of work translates into many different method calls, each of
which performs a little bit of work. Let's look at an example of how a simple iterative
solution can be turned into a recursive solution.
As a first example, we will explore a problem that has a simple iterative solution. It
won't be a very impressive use of recursion because the problem is easily solved with
iteration. But exploring a problem that has a straightforward iterative solution allows
us to compare the two solutions.
Suppose you want to create a method called
writeStars
that will take an integer
parameter
n
and will produce a line of output with exactly
n
stars on it. You can solve
this problem with a simple
for
loop:
public static void writeStars(int n) {
for (int i = 1; i <= n; i++) {
System.out.print("*");
}
System.out.println();
}
The action being repeated here is the call on
System.out.print
that prints a star.
To write the stars recursively, you need to think about different cases. You might ask
the method to produce a line with 10 stars, 20 stars, or 50 stars. Of all of the possible
star-writing tasks you might ask it to perform, which is the simplest?
Students often answer that printing a line of one star is very easy and they're right
that it's easy. But there is a task that is even easier. Printing a line of zero stars requires
almost no work at all. You can create such a line by calling
System.out.println
,so
you can begin your recursive definition with a test for this case:
public static void writeStars(int n) {
if (n == 0) {
System.out.println();
} else {
...
}
}
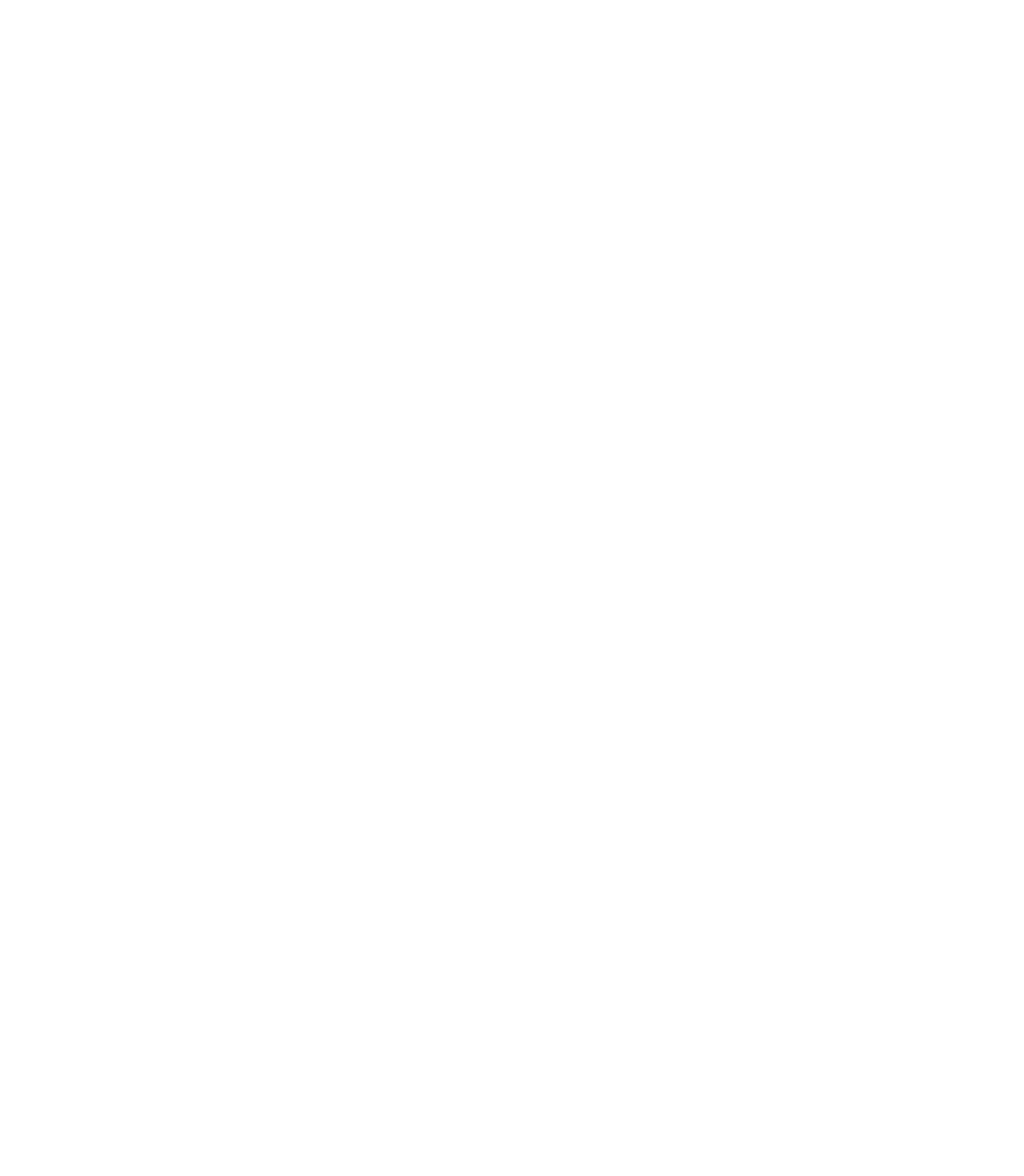
Search WWH ::

Custom Search