Java Reference
In-Depth Information
Whereas the original
ArrayList
version required up to several minutes to process
a list of one million elements on a modern computer, this new code finishes a million-
element list in under one-tenth of a second. It performs so quickly because the iterator
retains the current position in the list between calls to get or remove elements.
Iterators are also used internally by Java's for-each loop. When you use a for-each
loop like the following, Java is actually accessing the elements using an iterator
under the hood:
for (String word : list) {
System.out.println(word + " " + word.length());
}
Common Programming Error
Calling
next
on an Iterator Too Many Times
Iterators can be a bit confusing to new programmers, so you have to be careful to
use them correctly. The following code attempts to use an iterator to find and
return the longest string in a linked list, but it has a bug:
// returns the longest string in the list (does not work!)
public static String longest(LinkedList<String> list) {
Iterator<String> itr = list.iterator();
String longest = itr.next(); // initialize to first element
while (itr.hasNext()) {
if (itr.next().length() > longest.length()) {
longest = itr.next();
}
}
return longest;
}
The problem with the previous code is that its loop calls the
next
method on
the iterator in two places: once when it tests the length of the string, and again
when it tries to store the string as the longest. Each time you call
next
, the iterator
advances by one position, so if it's called twice in the loop, you'll skip an element
when you find a match. For example, if the list contains the elements (
"
oh
"
,
"
hello
"
,
"
how
"
,
"
are
"
,
"
you
"
), the program might see the
"
hello
"
and intend to
store it, but the second call to
next
would actually cause it to store the element
that follows
"
hello
"
, namely,
"
how
"
.
The solution is to save the result of the
itr.next()
call into a variable. The
following code would replace the
while
loop in the previous code:
Continued on next page

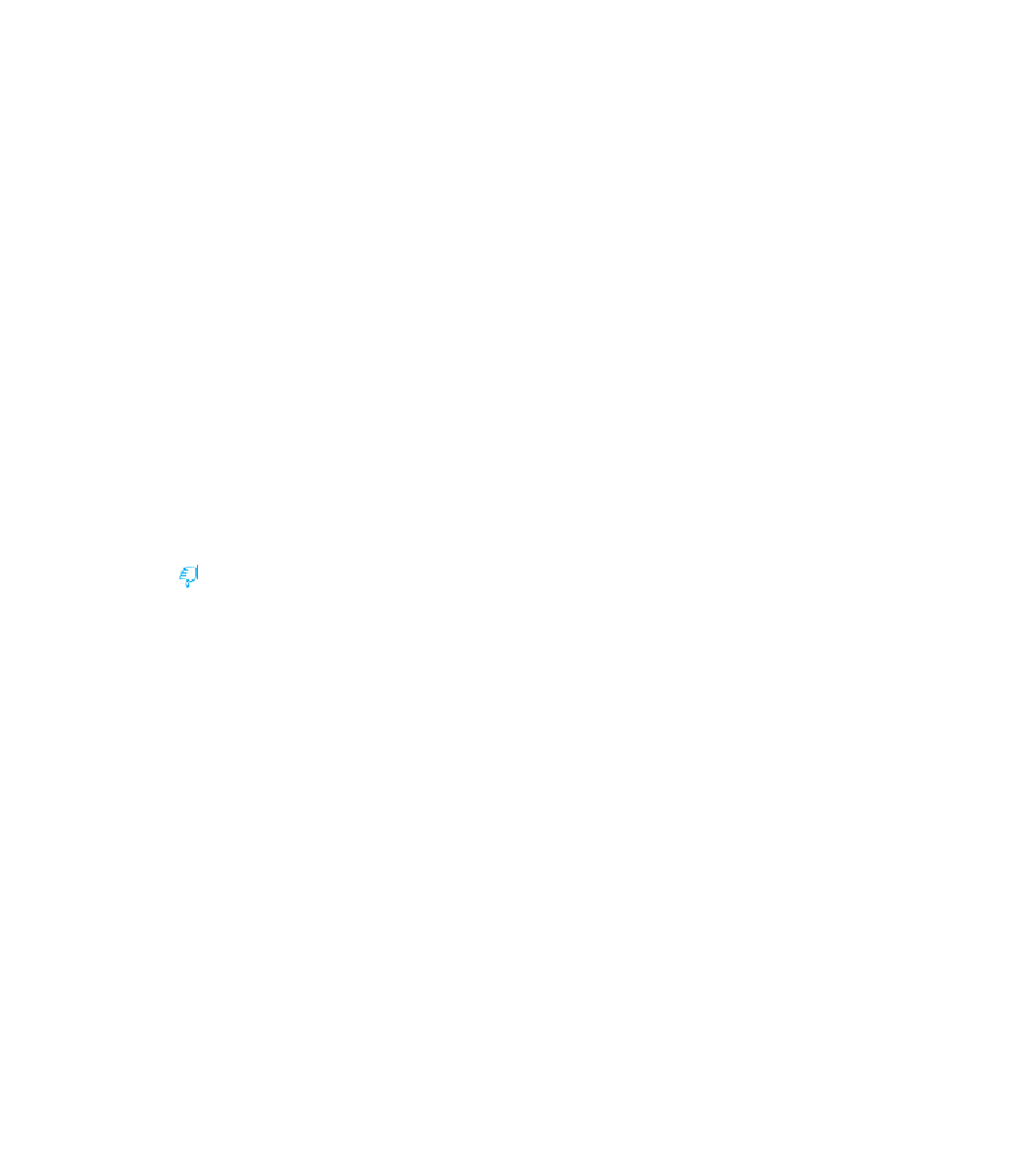
Search WWH ::

Custom Search