Java Reference
In-Depth Information
Continued from previous page
return the difference between the two characters.
} else {
return the difference between the two lengths.
}
Notice that this approach returns
0
in just the right case, when there are no character
pairs that differ and when the strings have the same length. Having the flexibility to
return any negative integer for “less than” and any positive integer for “greater than”
makes it easier to implement this approach.
The final reason not to specify the return values for
compareTo
is effi-
ciency. By having a less strict rule, Sun allows programmers to write faster
compareTo
methods. The
compareTo
method in the
String
class is one
of the most frequently called methods. All sorts of data comparisons are built
on
String
comparisons, and performing a task like sorting thousands of
records will lead to thousands of calls on the
String
class's
compareTo
method. As a result, it's important for the method to run quickly. We wouldn't
want to unnecessarily complicate the code by requiring that it always return
-1
,
0
, or
1
.
We can implement this class of dates with two fields to store the month and day:
public class CalendarDate {
private int month;
private int day;
public CalendarDate(int month, int day) {
this.month = month;
this.day = day;
}
// other methods
}
Remember that to implement an interface, you include an extra notation in the
class header. Implementing the
Comparable
interface is a little more challenging
because it is a generic interface (
Comparable<T>
). We can't simply write code like
the following:
// not correct
public class CalendarDate implements Comparable {
...
}
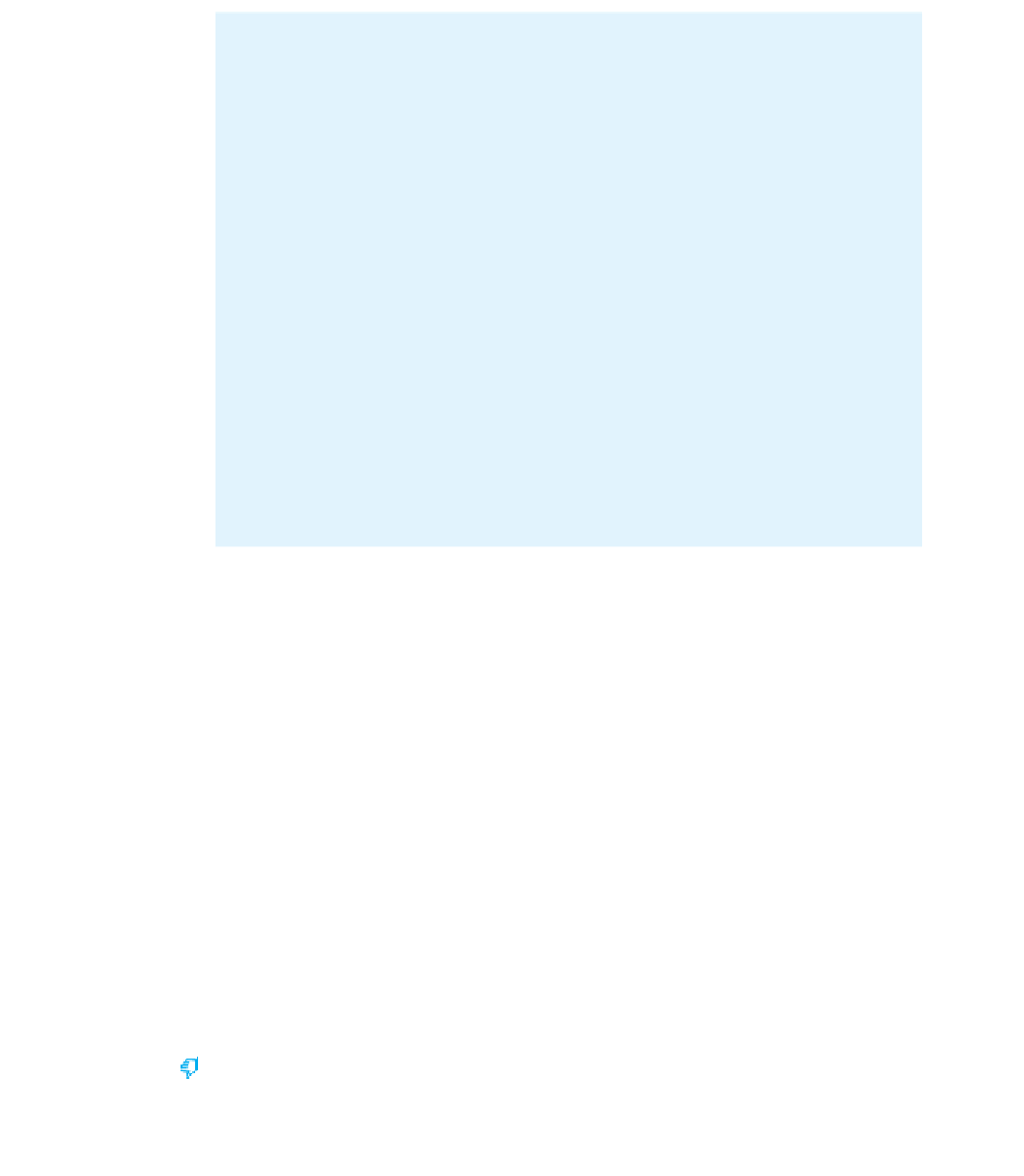
Search WWH ::

Custom Search