Java Reference
In-Depth Information
Not all types have natural orderings because not all types have comparison func-
tions. For example, in this chapter we have been exploring how to construct a variety
of
ArrayList
objects. How would you compare two
ArrayList
objects to deter-
mine whether one is less than another? What would it mean for one
ArrayList
to be
less than another? You might decide to use the lengths of the lists to determine which
one is less, but what would you do with two
ArrayList
objects of equal length that
store different values? You wouldn't want to describe them as “equal.” There is no
agreed-upon way of ordering
ArrayList
s, and therefore there is no comparison
function for this type. As a result, we say that the
ArrayList
type does not have a
natural ordering.
Java has a convention for indicating the natural ordering of a type. Any type that
has such an ordering should implement the
Comparable
interface:
public interface Comparable<T> {
public int compareTo(T other);
}
This interface provides a second example of a generic type in Java. In the case of
ArrayList
, Sun decided to use the letter “E,” which is short for “Element.” In the
case of
Comparable
, Sun used the letter “T,” which is short for “Type.”
The
compareTo
method is the comparison function for the type. A
boolean
return
type can't be used because there are three possible answers: less than, equal to, or
greater than. The convention for
compareTo
is that an object should return one of the
following results:
•
A negative number to indicate a less-than relationship
•
0
to indicate equality
•
A positive number to indicate a greater-than relationship
Let's look at a few examples to help you understand this concept. We have seen
that Java has
Integer
objects that serve as wrappers for individual
int
values. We
know how to compare
int
values to determine their relative order, so it is not surpris-
ing that the
Integer
class implements the
Comparable
interface. Consider the fol-
lowing code:
Integer x = 7;
Integer y = 42;
Integer z = 7;
System.out.println(x.compareTo(y));
System.out.println(x.compareTo(z));
System.out.println(y.compareTo(x));
This code begins by constructing three
Integer
objects:
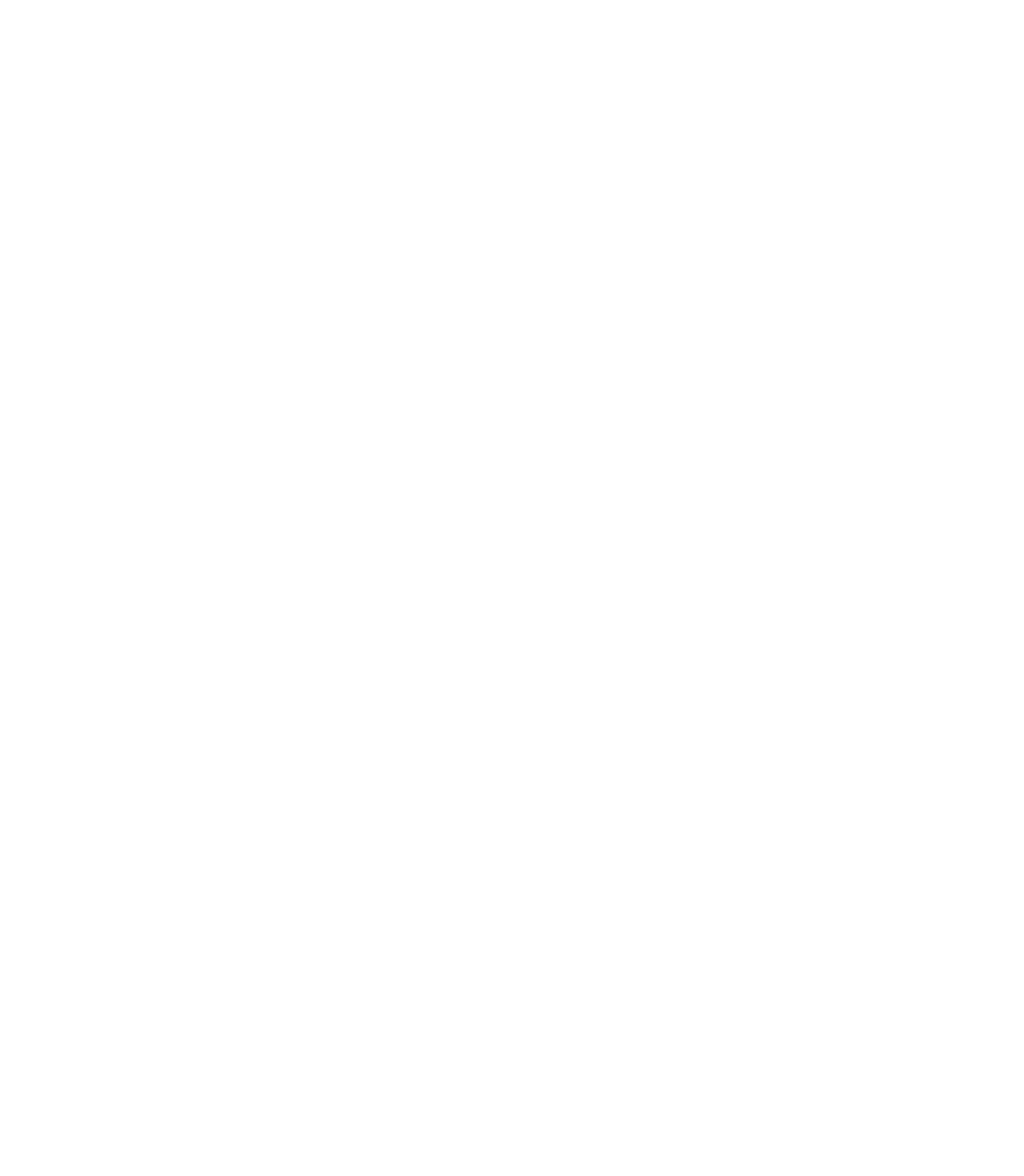
Search WWH ::

Custom Search