Java Reference
In-Depth Information
Table 10.3
Common Wrapper Classes
Primitive type
Wrapper class
int
Integer
double
Double
char
Character
boolean
Boolean
Notice that you can write a for-each loop to use a variable of type
int
even though
the
ArrayList
stores values of type
Integer
. Java will unbox the objects and per-
form the appropriate conversions for you.
Because Java has boxing and unboxing, the only place you generally need to use
the wrapper class is when you describe a type like
ArrayList<Integer>
. You can't
actually declare it to be of type
ArrayList<int>
, but you can manipulate it as if it is
of type
ArrayList<int>
.
Table 10.3 lists the major primitive types and their corresponding wrapper classes.
The method
Collections.sort
can be used to sort an
ArrayList
. It is part of the
java.util
package. The following short program demonstrates how to use
Collections.sort
:
1 // Constructs an ArrayList of Strings and sorts it.
2
3
import
java.util.*;
4
5
public class
SortExample {
6
public static void
main(String[] args) {
7 ArrayList<String> words =
new
ArrayList<String>();
8 words.add("four");
9 words.add("score");
10 words.add("and");
11 words.add("seven");
12 words.add("years");
13 words.add("ago");
14
15 // show list before and after sorting
16 System.out.println("before sort, words = " + words);
17 Collections.sort(words);
18 System.out.println("after sort, words = " + words);
19 }
20 }

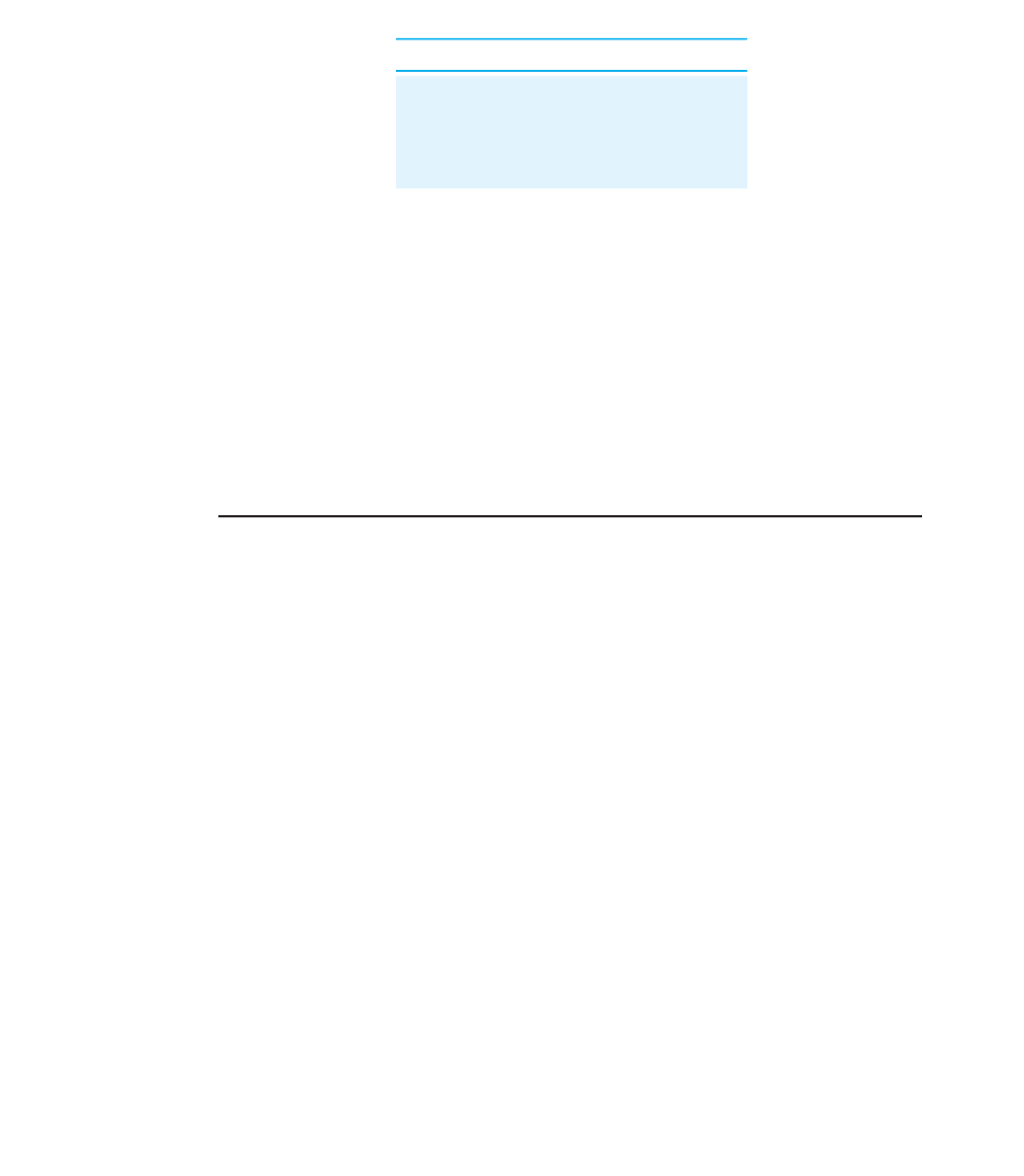
Search WWH ::

Custom Search