Java Reference
In-Depth Information
25
public void
purchase(
int
shares,
double
pricePerShare) {
26 totalShares += shares;
27 addCost(shares * pricePerShare);
28 }
29 }
1 // A MutualFund object represents a mutual fund asset.
2
public class
MutualFund
extends
ShareAsset {
3
private double
totalShares;
4
5 // constructs a new MutualFund investment with the given
6 // symbol and price per share
7
public
MutualFund(String symbol,
double
currentPrice) {
8
super
(symbol, currentPrice);
9 totalShares = 0.0;
10 }
11
12 // returns the market value of this mutual fund, which
13 // is the number of shares times the price per share
14
public double
getMarketValue() {
15
return
totalShares * getCurrentPrice();
16 }
17
18 // returns the number of shares of this mutual fund
19
public double
getTotalShares() {
20
return
totalShares;
21 }
22
23 // records purchase of the given shares at the given price
24
public void
purchase(
double
shares,
double
pricePerShare) {
25 totalShares += shares;
26 addCost(shares * pricePerShare);
27 }
28 }
Abstract classes can do everything interfaces can do and more, but this does not
mean that it is always better to use them than interfaces. One important difference
between interfaces and abstract classes is that a class may choose to implement arbi-
trarily many interfaces, but it can extend just one abstract class. That is why an inter-
face often forms the top of an inheritance hierarchy, as our
Asset
interface did in this
design. Such placement allows classes to become part of the hierarchy without having
it consume their only inheritance relationships.
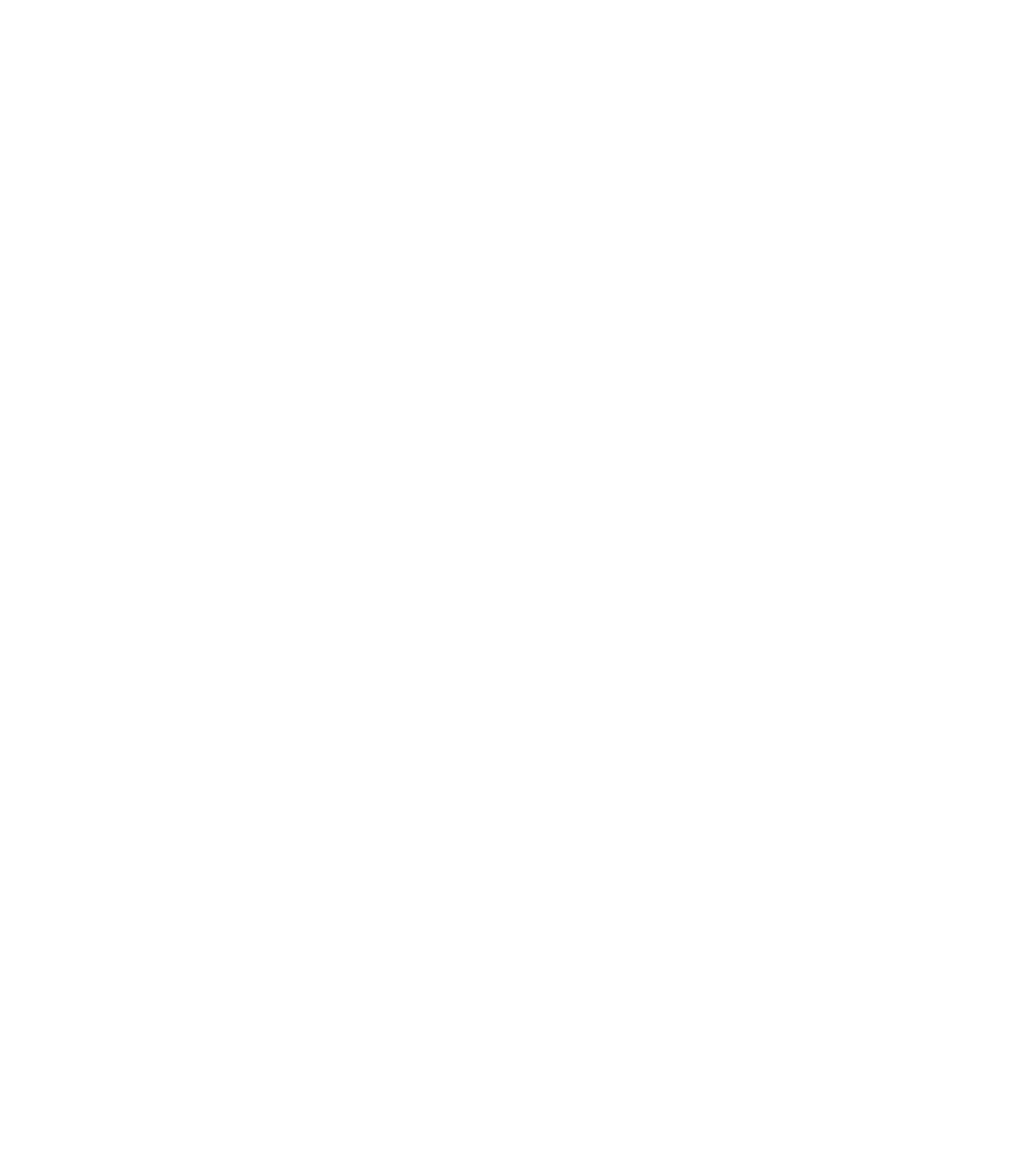
Search WWH ::

Custom Search