Java Reference
In-Depth Information
<interface>
Shape
getArea()
getPerimeter()
Circle
radius
Circle(radius)
getArea()
getPerimeter()
Rectangle
width, height
Rectangle(w, h)
getArea()
getPerimeter()
Triangle
a, b, c
Tr iangle(a, b, c)
getArea()
getPerimeter()
Figure 9.5
Hierarchy of shape classes
The major benefit of interfaces is that we can use them to achieve polymorphism.
We can create an array of
Shape
s, pass a
Shape
as a parameter to a method, return a
Shape
from a method, and so on. The following program uses the shape classes in an
example that is similar to the polymorphism exercises in Section 9.3:
1 // Demonstrates shape classes.
2
public class
ShapesMain {
3
public static void
main(String[] args) {
4 Shape[] shapes =
new
Shape[3];
5 shapes[0] =
new
Rectangle(18, 18);
6 shapes[1] =
new
Triangle(30, 30, 30);
7 shapes[2] =
new
Circle(12);
8
9
for
(
int
i = 0; i < shapes.length; i++) {
10 System.out.println("area = " + shapes[i].getArea() +
11 ", perimeter = " +
12 shapes[i].getPerimeter());
13 }
14 }
15 }
This program produces the following output:
area = 324.0, perimeter = 72.0
area = 389.7114317029974, perimeter = 90.0
area = 452.3893421169302, perimeter = 75.39822368615503
It would be fairly easy to modify our client program if another shape class, such as
Hexagon
or
Ellipse
, were added to the hierarchy. This is another example of the


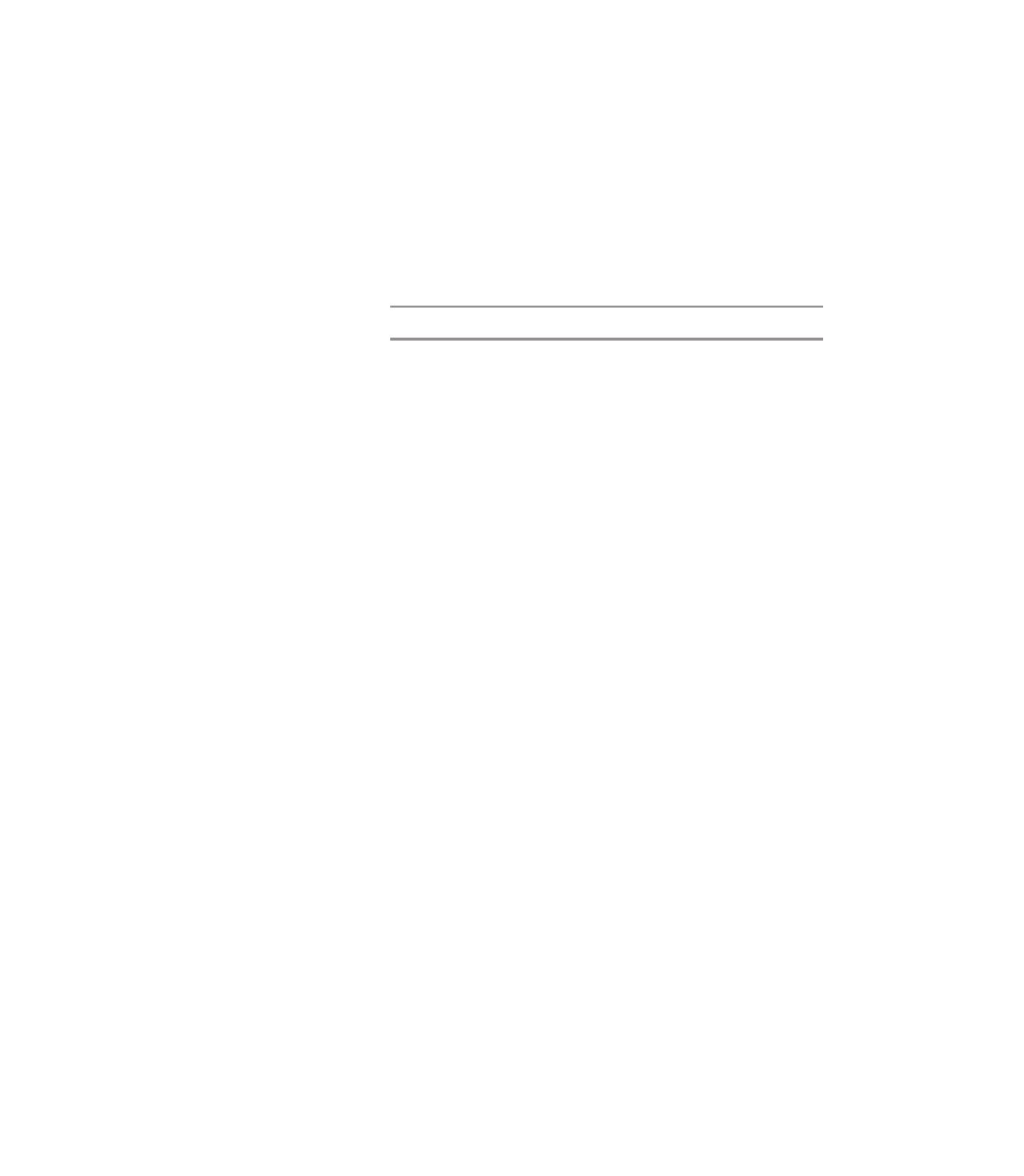
Search WWH ::

Custom Search