Java Reference
In-Depth Information
An interface is like a class, but it contains only method headers without bodies. A
class can promise to
implement
an interface, meaning that the class promises to pro-
vide implementations of all the methods that are declared in the interface. Classes
that implement an interface form an is-a relationship with that interface. In a system
with an interface that is implemented by several classes, polymorphic code can handle
objects from any of the classes that implement that interface.
A nonprogramming analogy for an interface is a professional certification. It's
possible for a person to become certified as a teacher, nurse, accountant, or doctor. To
do this, the person must demonstrate certain abilities required of members of those
professions. When employers hire someone who has received the proper certification,
they expect that the person will be able to perform certain job duties. An interface
acts as a certification that classes can meet by implementing all the behavior
described in the interface. Code that receives an object implementing an interface can
rely on the object having certain behavior.
Interfaces are also used to define roles that objects can play; for example, a
Date
class might implement the
Comparable
interface to indicate that
Date
objects can be
compared to each other, or a
Point
class might implement the
Cloneable
interface
to indicate that a
Point
object can be replicated.
Interfaces are used in many other places in Java's class libraries. Here are just a
few of Java's important interfaces:
•
The
ActionListener
interface in the
java.awt
package is used to assign
behavior to events when a user clicks on a button or other graphical control.
•
The
Serializable
interface in the
java.io
package denotes classes whose
objects may be saved to files and transferred over a network.
•
The
Comparable
interface allows you to describe how to compare objects of
your type to determine which are less than, greater than, or equal to each other.
This technique can be used to search or sort a collection of objects.
•
The
Formattable
interface lets objects describe different ways that they can be
printed by the
System.out.printf
command.
•
The
Runnable
interface is used for multithreading, which allows a program to
execute two pieces of code at the same time.
•
Interfaces such as
List
,
Set
,
Map
, and
Iterator
in the
java.util
package
describe data structures that you can use to store collections of objects.
We will cover some of these interfaces in later chapters.
In this section we'll use an interface to define a polymorphic hierarchy of shape
classes without sharing code between them. Imagine that we are creating classes to
represent many different types of shapes, such as rectangles, circles, and triangles. We
might be tempted to use inheritance with these shape classes because they seem to
share some common behavior (all shapes have an area and a perimeter, for example).
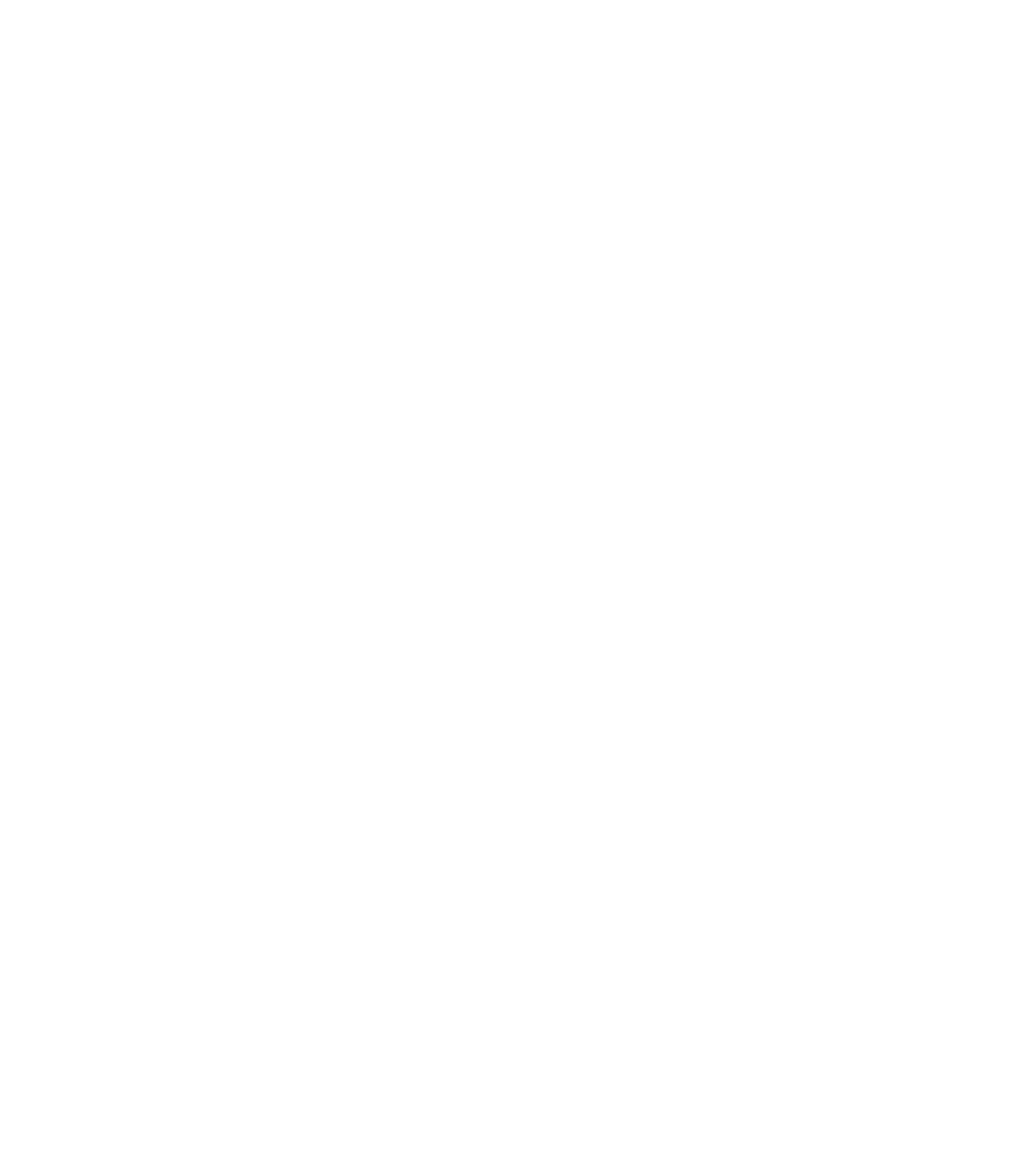
Search WWH ::

Custom Search