Java Reference
In-Depth Information
13
14 // returns the total profit or loss earned on this stock
15
public double
getProfit(
double
currentPrice) {
16
double
marketValue = totalShares * currentPrice;
17
return
marketValue - totalCost;
18 }
19
20 // records purchase of the given shares at the given price
21
public void
purchase(
int
shares,
double
pricePerShare) {
22 totalShares += shares;
23 totalCost += shares * pricePerShare;
24 }
25 }
Now let's imagine that you want to create a type of object for stocks which pay
dividends. Dividends are profit-sharing payments that a corporation pays its share-
holders. The amount that each shareholder receives is proportional to the number of
shares that person owns. Not every stock pays dividends, so you wouldn't want to
add this functionality directly to the
Stock
class. Instead, you should create a new
class called
DividendStock
that extends
Stock
and adds this new behavior.
Each
DividendStock
object will inherit the symbol, total shares, and total cost
from the
Stock
superclass. You'll simply need to add a field to record the amount of
the dividends paid:
public class DividendStock extends Stock {
private double dividends; // amount of dividends paid
...
}
Using the
dividends
field, you can write a method in the
DividendStock
class
that lets the shareholder receive a per-share dividend. Your first thought might be to
write code like the following, but this won't compile:
// this code does not compile
public void payDividend(double amountPerShare) {
dividends += amountPerShare * totalShares;
}
A
DividendStock
cannot access the
totalShares
field it has inherited, because
totalShares
is declared private in
Stock
. A subclass may not refer directly to any
private fields that were declared in its superclass, so you'll get a compiler error like
the following:
DividendStock.java:17: totalShares has private access in Stock
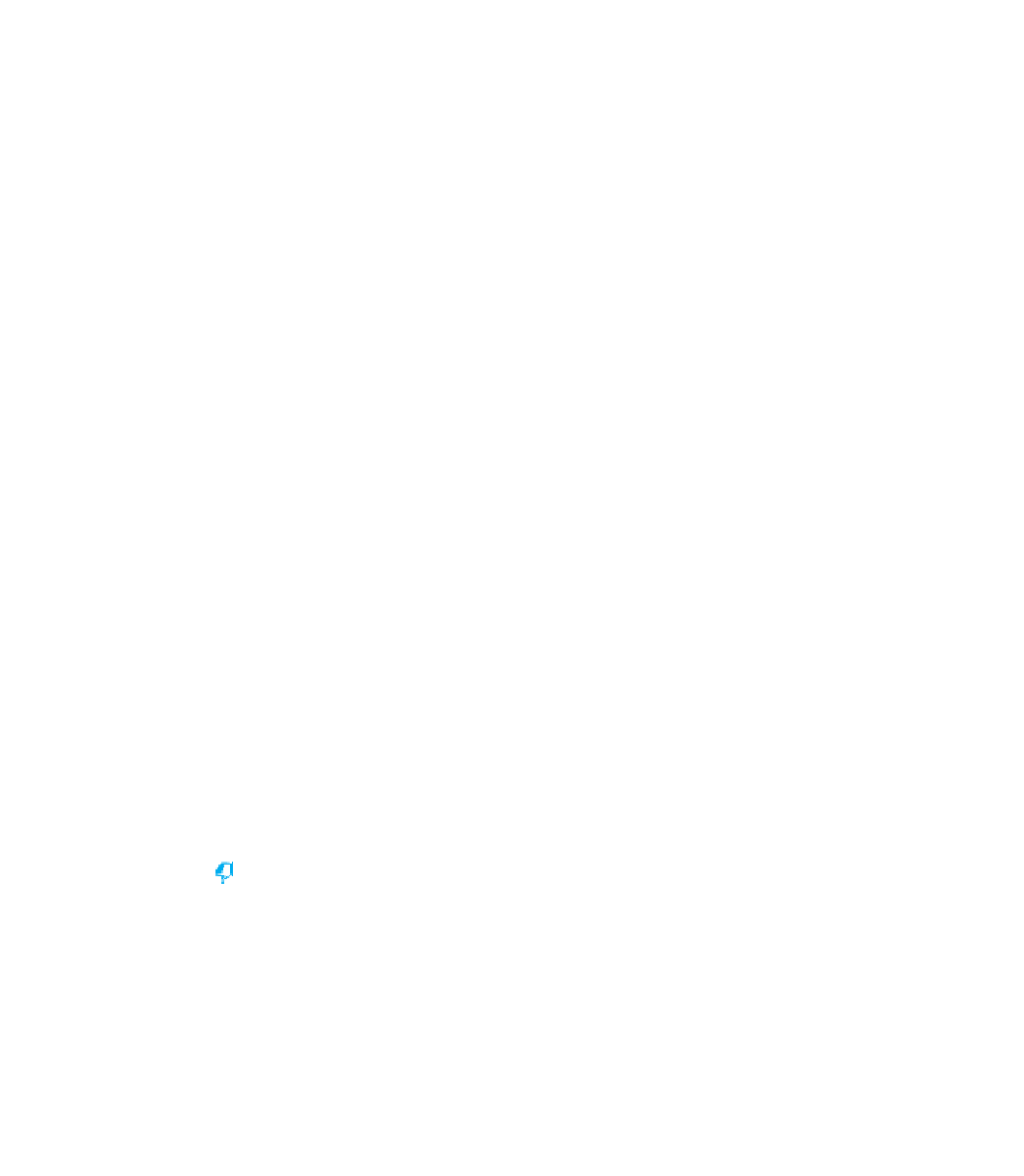
Search WWH ::

Custom Search