Java Reference
In-Depth Information
array are between 0 and 99 inclusive. For example, the median of {5, 2, 4, 17, 55, 4, 3, 26, 18, 2, 17} is 5 and the
median of {42, 37, 1, 97, 1, 2, 7, 42, 3, 25, 89, 15, 10, 29, 27} is 25. (
Hint
: You may wish to look at the
Tally
pro-
gram from earlier in this chapter for ideas.)
9.
Write a method called
minGap
that accepts an integer array as a parameter and returns the minimum difference or
gap between adjacent values in the array, where the gap is defined as the later value minus the earlier value. For
example, in the array {1, 3, 6, 7, 12}, the first gap is 2 (3
1), the second gap is 3 (6
3), the third gap is 1 (7
6),
and the fourth gap is 5 (12
7). So your method should return 1 if passed this array. The minimum gap could be a
negative number if the list is not in sorted order. If you are passed an array with fewer than two elements, return 0.
10.
Write a method called
percentEven
that accepts an array of integers as a parameter and returns the percentage of
even numbers in the array as a real number. For example, if the array stores the elements {6, 2, 9, 11, 3}, then your
method should return
40.0
. If the array contains no even elements or no elements at all, return
0.0
.
11.
Write a method called
isUnique
that accepts an array of integers as a parameter and returns a
boolean
value indi-
cating whether or not the values in the array are unique (
true
for yes,
false
for no). The values in the list are con-
sidered unique if there is no pair of values that are equal. For example, if passed an array containing {3, 8, 12, 2, 9,
17, 43, -8, 46}, your method should return
true
, but if passed {4, 7, 3, 9, 12, -47, 3, 74}, your method should return
false
because the value 3 appears twice.
12.
Write a method called
priceIsRight
that mimics the guessing rules from the game show
The Price is Right
. The
method accepts as parameters an array of integers representing the contestants' bids and an integer representing a
correct price. The method returns the element in the bids array that is closest in value to the correct price without
being larger than that price. For example, if an array called
bids
stores the values {200, 300, 250, 1, 950, 40}, the
call of
priceIsRight(bids, 280)
should return 250, since 250 is the bid closest to 280 without going over 280.
If all bids are larger than the correct price, your method should return
-1
.
13.
Write a method called
longestSortedSequence
that accepts an array of integers as a parameter and returns the
length of the longest sorted (nondecreasing) sequence of integers in the array. For example, in the array {3, 8, 10, 1,
9, 14, -3, 0, 14, 207, 56, 98, 12}, the longest sorted sequence in the array has four values in it (the sequence -3, 0, 14,
207), so your method would return 4 if passed this array. Sorted means nondecreasing, so a sequence could contain
duplicates. Your method should return 0 if passed an empty array.
14.
Write a method called
contains
that accepts two arrays of integers
a1
and
a2
as parameters and that returns a
boolean
value indicating whether or not the sequence of elements in
a2
appears in
a1
(
true
for yes,
false
for no).
The sequence must appear consecutively and in the same order. For example, consider the following arrays:
int[] list1 = {1, 6, 2, 1, 4, 1, 2, 1, 8};
int[] list2 = {1, 2, 1};
The call of
contains(list1, list2)
should return
true
because the sequence of values in
list2
{1, 2, 1} is con-
tained in
list1
starting at index 5. If
list2
had stored the values {2, 1, 2}, the call of
contains(list1, list2)
would return
false
. Any two lists with identical elements are considered to contain each other. Every array contains
the empty array, and the empty array does not contain any arrays other than the empty array itself.
15.
Write a method called
collapse
that accepts an array of integers as a parameter and returns a new array containing the
result of replacing each pair of integers with the sum of that pair. For example, if an array called
list
stores the values
{7, 2, 8, 9, 4, 13, 7, 1, 9, 10}, then the call of
collapse(list)
should return a new array containing {9, 17, 17, 8, 19}.
The first pair from the original list is collapsed into 9 (7
9), and so on. If
the list stores an odd number of elements, the final element is not collapsed. For example, if the list had been {1, 2, 3, 4,
5}, then the call would return {3, 7, 5}. Your method should not change the array that is passed as a parameter.
2), the second pair is collapsed into 17 (8
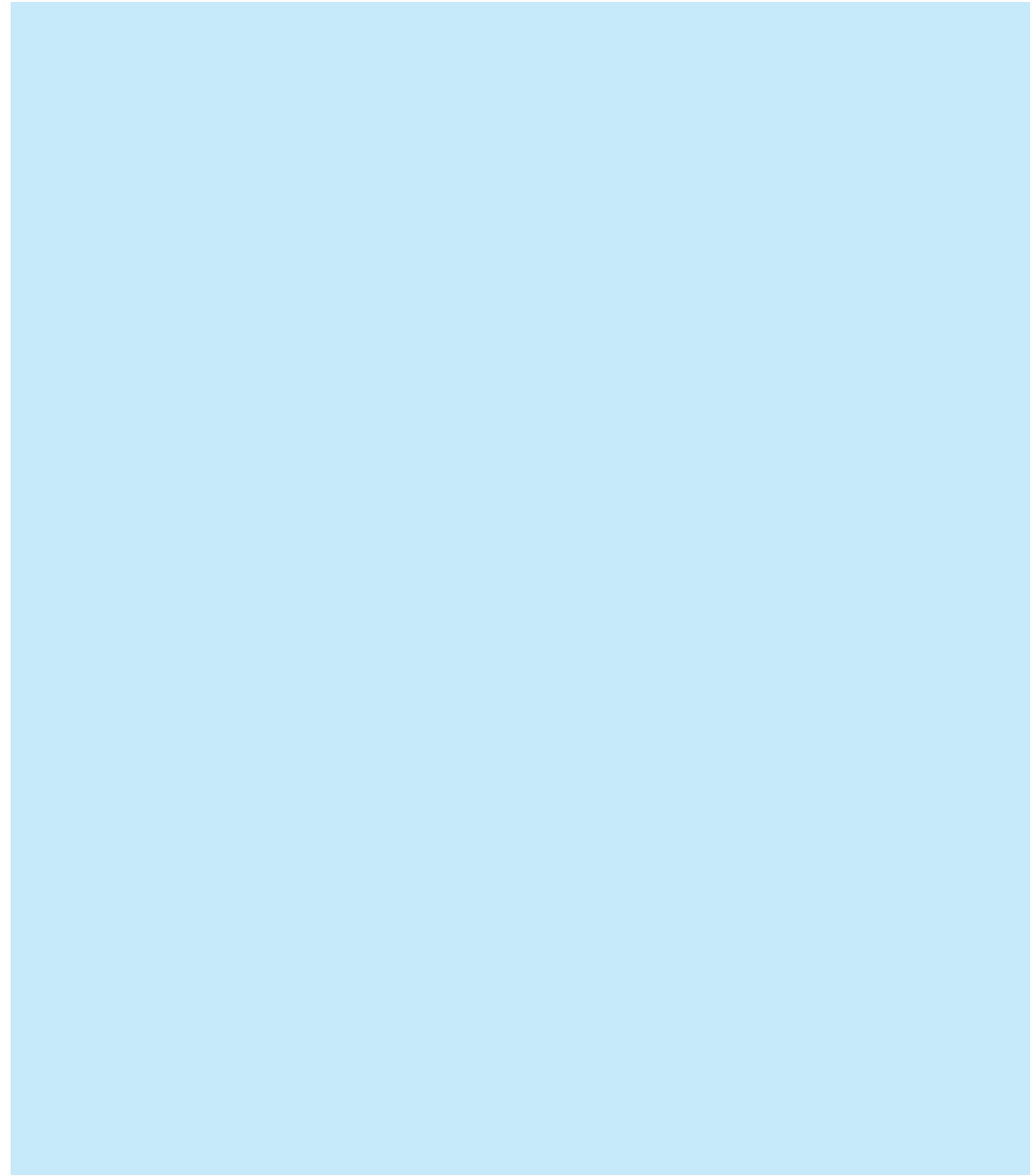
Search WWH ::

Custom Search