Java Reference
In-Depth Information
In this case the user has specified two extra arguments that are file names that the
program should use (e.g., the names of an input and output file). If the user types
these extra arguments when starting up Java, the
String[] args
parameter to
main
will be initialized to an array of length 2 that stores these two strings:
[0]
[1]
args
"temperature.dat"
"temperature.out"
All of the algorithms we have seen have been written with a single loop. But many
computations require nested loops. For example, suppose that you were asked to print
all inversions in an array of integers. An inversion is defined as a pair of numbers in
which the first number in the list is greater than the second number.
In a sorted list such as [1, 2, 3, 4], there are no inversions at all and there is noth-
ing to print. But if the numbers appear instead in reverse order, [4, 3, 2, 1], then there
are many inversions to print. We would expect output like the following:
(4, 3)
(4, 2)
(4, 1)
(3, 2)
(3, 1)
(2, 1)
Notice that any given number (e.g., 4 in the list above) can produce several differ-
ent inversions, because it might be followed by several smaller numbers (1, 2, and 3
in the example). For a list that is partially sorted, as in [3, 1, 4, 2], there are only a
few inversions, so you would produce output like this:
(3, 1)
(3, 2)
(4, 2)
This problem can't be solved with a single traversal because we are looking for
pairs of numbers. There are many possible first values in the pair and many possible
second values in the pair. Let's develop a solution using pseudocode.
We can't produce all pairs with a single loop, but we can use a single loop to con-
sider all possible first values:
for (every possible first value) {
print all inversions that involve this first value.
}




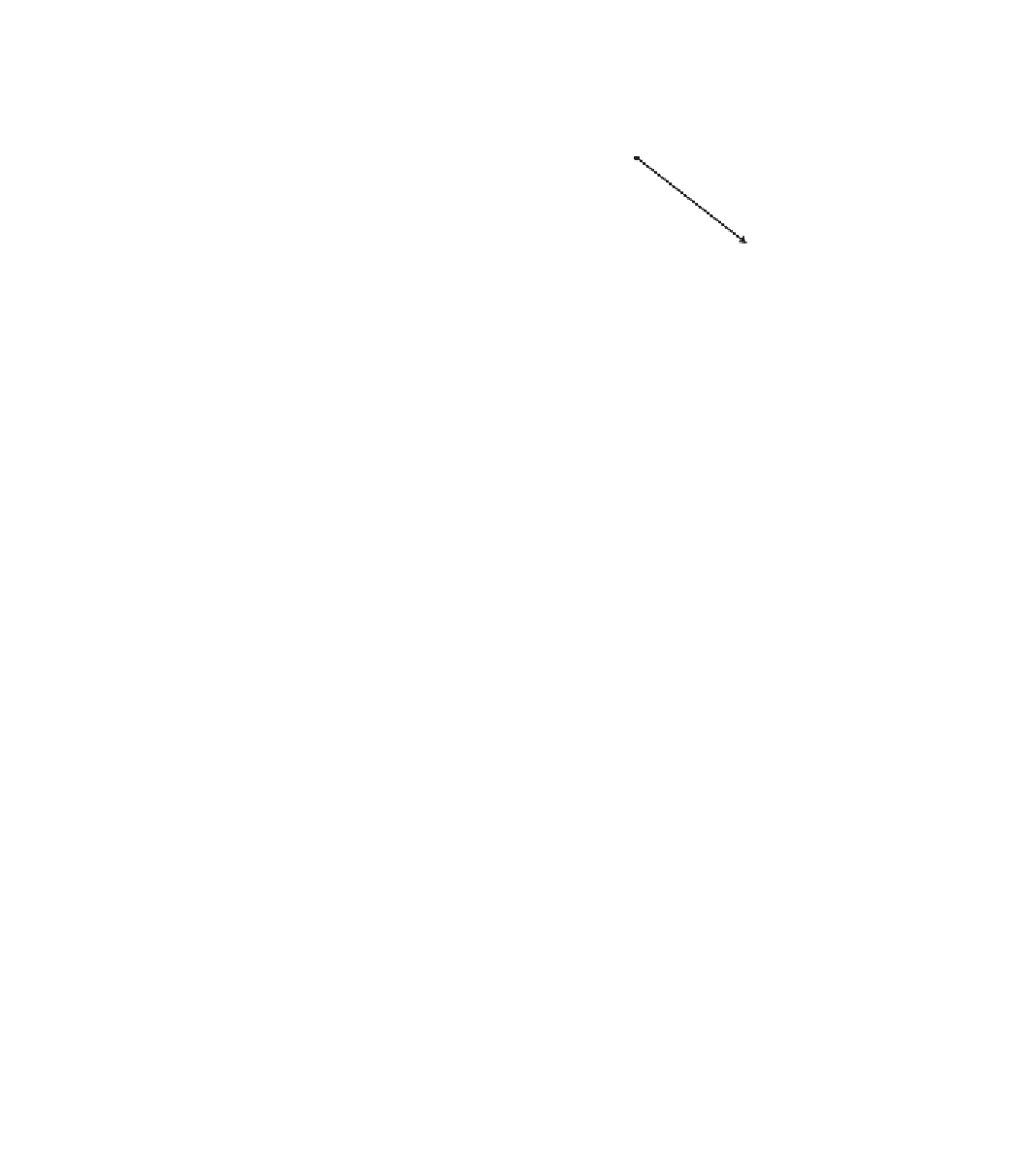
Search WWH ::

Custom Search