Java Reference
In-Depth Information
Java has a special syntax for initializing an array when you know exactly what you
want to put into it. For example, you could write the following code to initialize an
array of integers to keep track of the number of days that are in each month (“Thirty
days hath September . . .”) and an array of
Strings
to keep track of the abbreviations
for the days of the week:
int[] daysIn = new int[12];
daysIn[0] = 31;
daysIn[1] = 28;
daysIn[2] = 31;
daysIn[3] = 30;
daysIn[4] = 31;
daysIn[5] = 30;
daysIn[6] = 31;
daysIn[7] = 31;
daysIn[8] = 30;
daysIn[9] = 31;
daysIn[10] = 30;
daysIn[11] = 31;
String[] dayNames = new String[7];
dayNames[0] = "Mon";
dayNames[1] = "Tue";
dayNames[2] = "Wed";
dayNames[3] = "Thu";
dayNames[4] = "Fri";
dayNames[5] = "Sat";
dayNames[6] = "Sun";
This code works, but it's a rather tedious way to declare these arrays. Java pro-
vides a shorthand:
int[] daysIn = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
String[] dayNames = {"Mon", "Tue", "Wed", "Thu", "Fri", "Sat", "Sun"};
The general syntax for array initialization is as follows:
<element type>[] <name> = {<value>, <value>, ..., <value>};
You use the curly braces to enclose a series of values that will be stored in the
array. The order of the values is important. The first value will go into index 0, the
second value will go into index 1, and so on. Java counts how many values you
include and constructs an array that is just the right size. It then stores the various
values into the appropriate spots in the array.
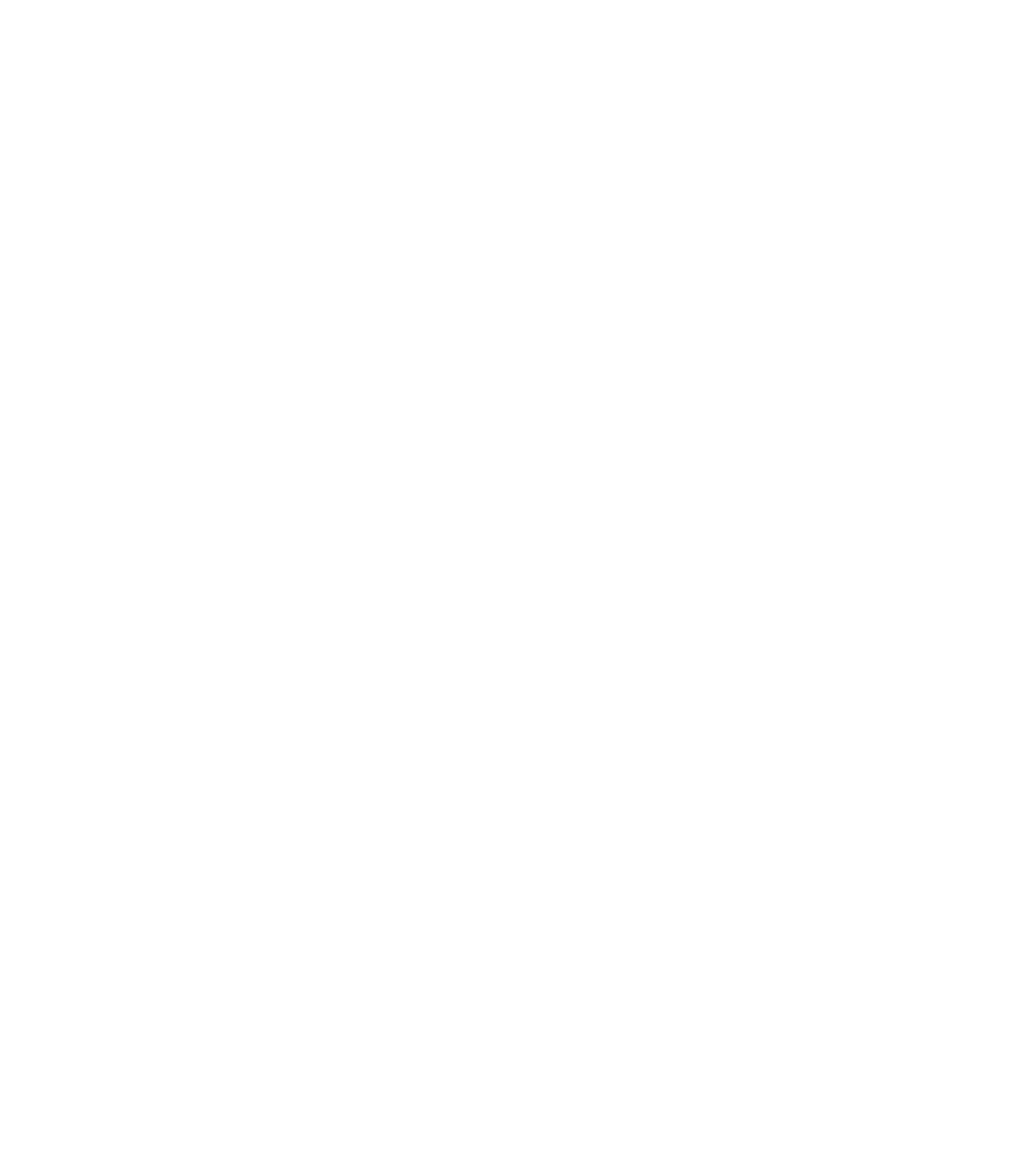
Search WWH ::

Custom Search