Java Reference
In-Depth Information
We can then rewrite the
fillWithOdds
method so that it constructs and returns
the array:
public static int[] buildOddArray(int size) {
int[] data = new int[size];
for (int i = 0; i < data.length; i++) {
data[i] = 2 * i + 1;
}
return data;
}
Pay close attention to the header of the preceding method. It no longer has the
array as a parameter, and its return type is
int[]
rather than
void
. It also ends with a
return
statement that returns a reference to the array that it constructs.
Putting this all together along with some code to print the contents of the array, we
end up with the following complete program:
1 // Sample program with arrays passed as parameters
2
3
public class
IncrementOdds {
4
public static void
main(String[] args) {
5
int
[] list = buildOddArray(5);
6 incrementAll(list);
7
for
(
int
i = 0; i < list.length; i++) {
8 System.out.print(list[i] + " ");
9 }
10 System.out.println();
11 }
12
13 // returns array of given size composed of consecutive odds
14
public static int
[] buildOddArray(
int
size) {
15
int
[] data =
new int
[size];
16
for
(
int
i = 0; i < data.length; i++) {
17 data[i] = 2 * i + 1;
18 }
19
return
data;
20 }
21
22 // adds one to each array element
23
public static void
incrementAll(
int
[] data) {
24
for
(
int
i = 0; i < data.length; i++) {
25 data[i]++;
26 }
27 }
28 }
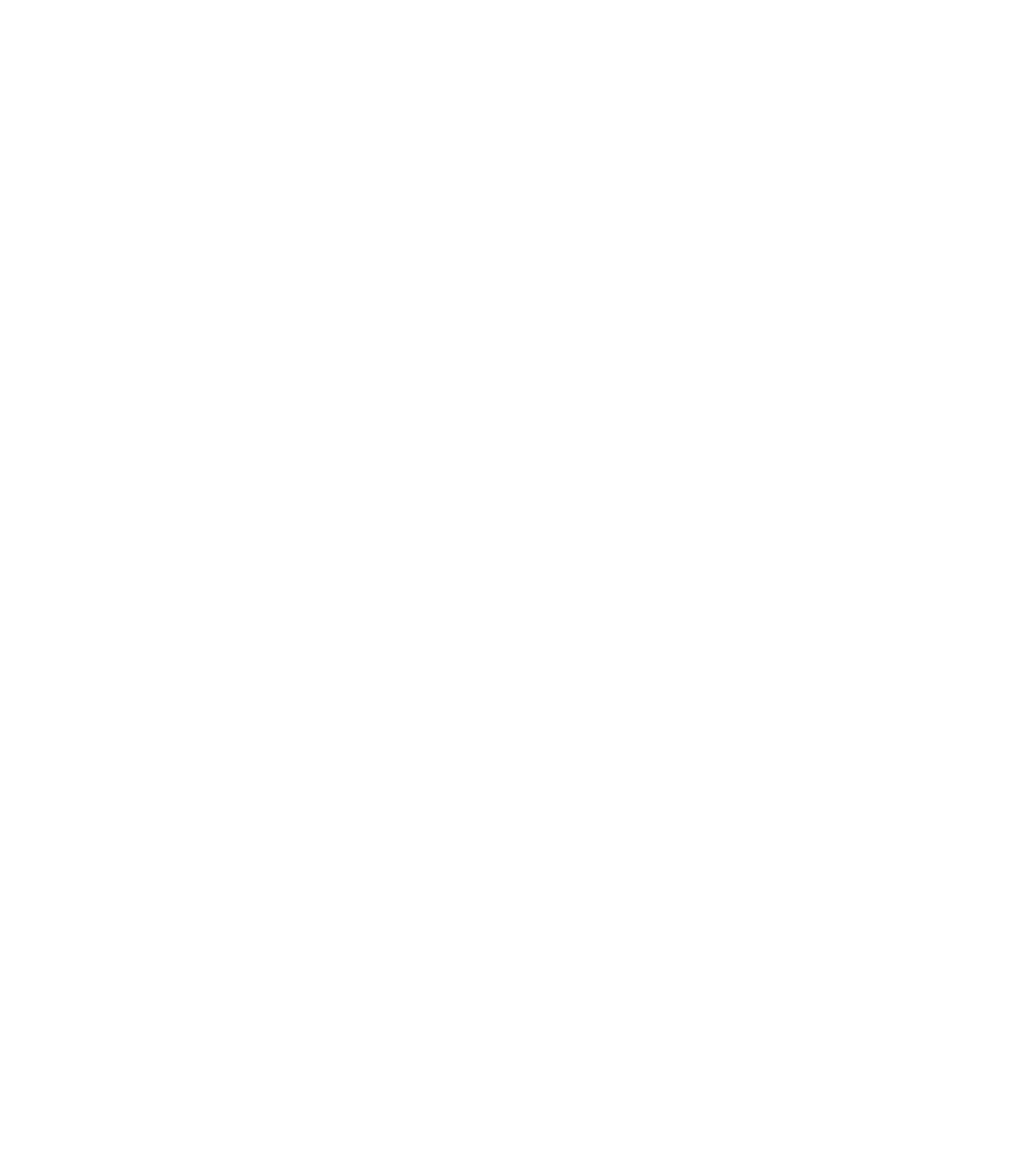
Search WWH ::

Custom Search