Java Reference
In-Depth Information
Continued from previous page
System.out.print("Day " + i + "'s high temp: ");
temps[i] = console.nextInt();
sum += temps[i];
}
Because the array is indexed starting at 0, you changed the bounds of the
for
loop to start at
0
and adjusted the
print
statement. Suppose those were the only
changes you made:
// still wrong loop bounds
for (int i = 0; i <= numDays; i++) {
System.out.print("Day " + (i + 1) + "'s high temp: ");
temps[i] = console.nextInt();
sum += temps[i];
}
This loop generates an error when you run the program. The loop asks for an
extra day's worth of data and then throws an exception. Here's a sample execution:
How many days' temperatures?
5
Day 1's high temp:
82
Day 2's high temp:
80
Day 3's high temp:
79
Day 4's high temp:
71
Day 5's high temp:
75
Day 6's high temp:
83
Exception in thread "main"
java.lang.ArrayIndexOutOfBoundsException: 5
at Temperature2.main(Temperature2.java:18)
The problem is that if you're going to start the
for
loop variable at
0
, you
need to do a test to ensure that it is strictly less than the number of iterations you
want. You changed the
1
to a
0
but left the
<=
test. As a result, the loop is per-
forming an extra iteration and trying to make a reference to an array element
temps[5]
that doesn't exist.
This is a classic off-by-one error. The fix is to change the loop bounds to use a
strictly less-than test:
// correct bounds
for (int i = 0; i < numDays; i++) {
System.out.print("Day " + (i + 1) + "'s high temp: ");
temps[i] = console.nextInt();
sum += temps[i];
}
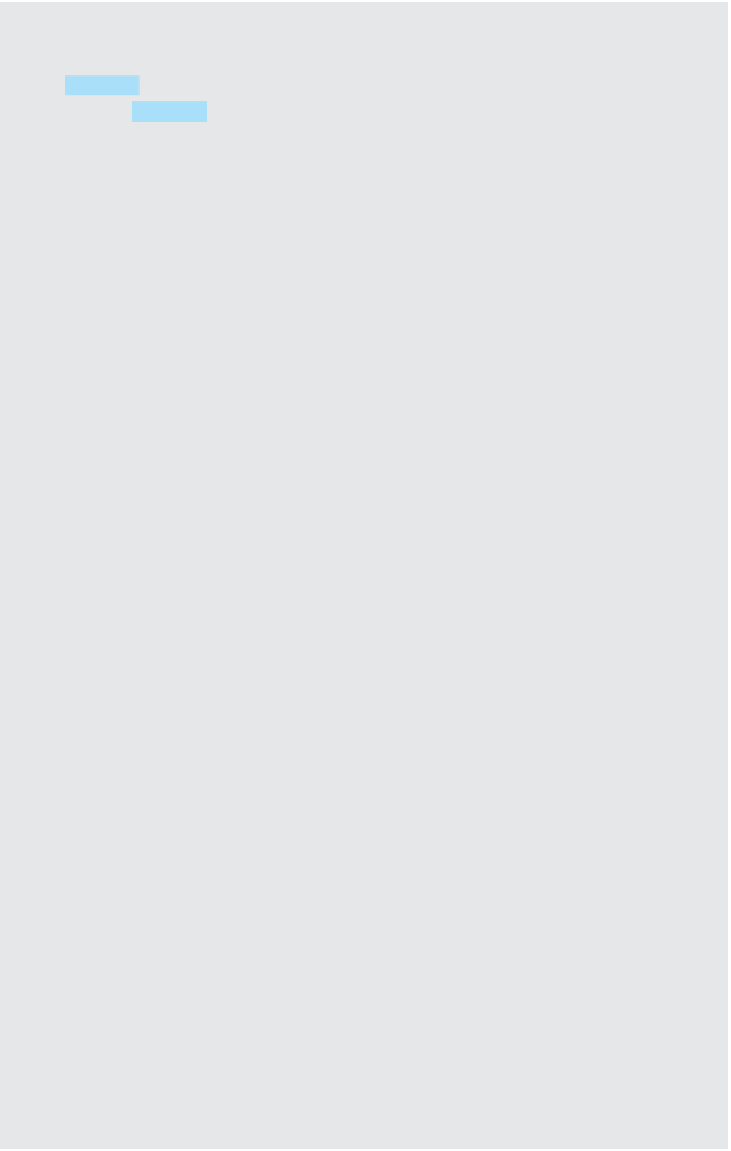



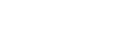

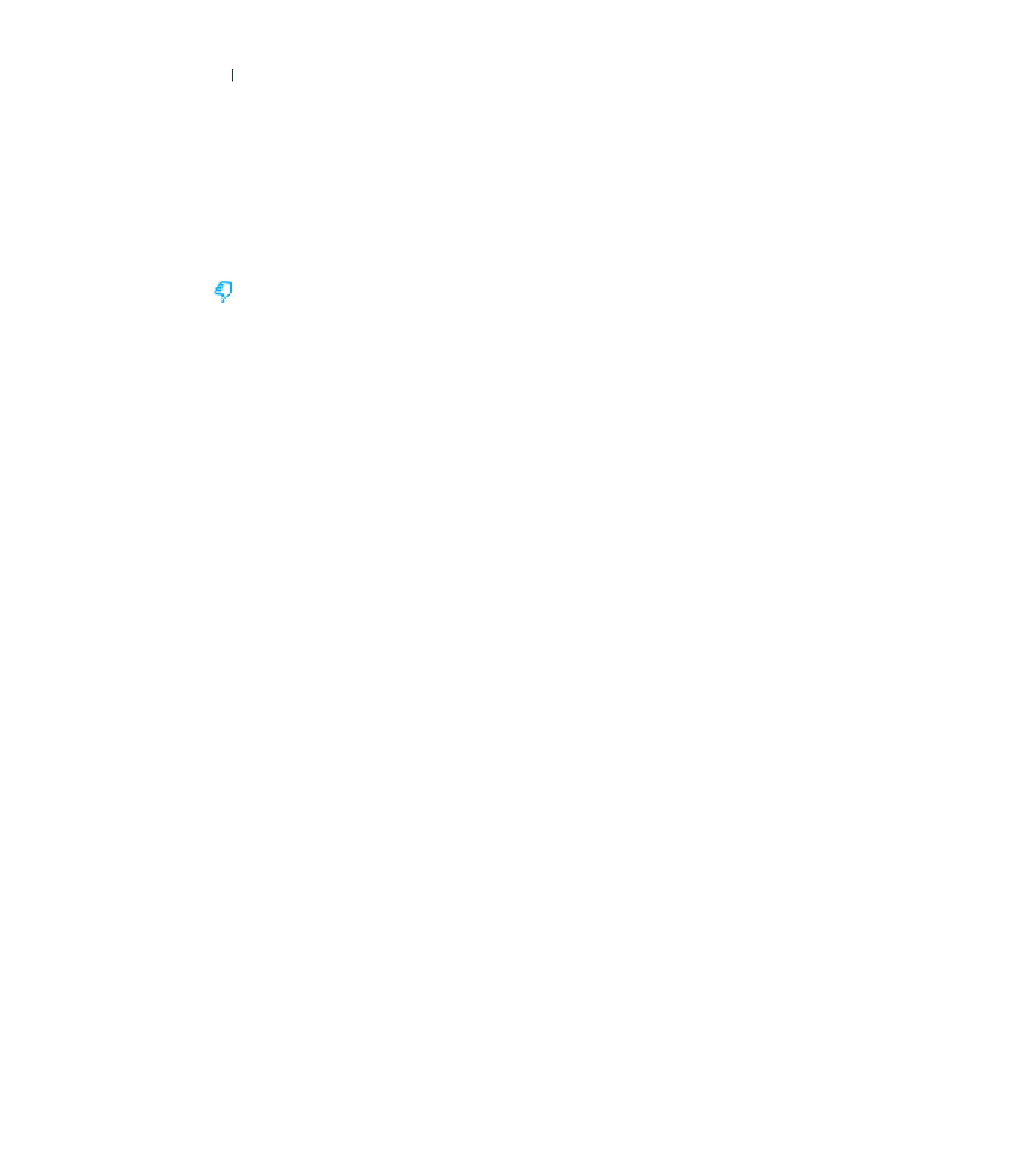

Search WWH ::

Custom Search